Implementing the ModalDialog
Example
The following shows an example of the ModalDialog component. In this case, the modal window is displayed on initial player display, but methods exist to display it programmatically also. Here some yellow text is all that is displayed in the modal window. The modal window blocks all interaction with the player until the X in the top-right corner is clicked, or ESC is pressed.
Creating the ModalDialog
There are various ways to create implement the ModalDialog, the two being used in this document are:
- Using
createModal()
, a helper function - Using the
ModalDialog()
constructor
Using the createModal() helper function
The createModal()
method encapsulates code to make it easier for you to use the ModalDialog. The syntax is as follows:
createModal(content,options)
Parameter | Required | Description |
---|---|---|
content | No (although if not provided, nothing will be displayed by the modal) |
Content to display in the modal |
options | No | An object to set other options for the modal; detailed later in this document |
The following shows a simple implementation of the ModalDialog being created and used with Brightcove Player.
<video-js id="myPlayerID"
data-video-id="5079788144001"
data-account="1507807800001"
data-player="HJLWns1Zbx"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/HJLWns1Zbx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.createModal('Using the helper function');
});
</script>
<video-js id="myPlayerID"
data-account="3676484087001"
data-player="S1lOCfk6Ze"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/3676484087001/S1lOCfk6Ze_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.createModal('Using the helper function');
});
</script>
Using the ModalDialog constructor
You can also use the class' constructor to implement the ModalDialog. This is a two step process, getting the class then instantiating an object. The syntax is as follows:
var ModalDialog = videojs.getComponent('ModalDialog');
var myModal = new ModalDialog(player, options);
Parameter | Required | Description |
---|---|---|
player | Yes | The player to which the modal will be applied |
options | No | An object to set other options for the modal; detailed in the next section |
The following shows an implementation of the ModalDialog being created and used with Brightcove Player.
<video-js id="myPlayerID"
data-video-id="5079788144001"
data-account="1507807800001"
data-player="B1mMJs3Ge"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/B1mMJs3Ge_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.content = 'In the modal';
options.label = 'the label';
var ModalDialog = videojs.getComponent('ModalDialog');
var myModal = new ModalDialog(myPlayer, options);
myPlayer.addChild(myModal);
myModal.open();
});
</script>
<video-js id="myPlayerID"
data-account="3676484087001"
data-player="S1lOCfk6Ze"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/3676484087001/S1lOCfk6Ze_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.content = 'In the modal';
options.label = 'the label';
var ModalDialog = videojs.getComponent('ModalDialog');
var myModal = new ModalDialog(myPlayer, options);
myPlayer.addChild(myModal);
myModal.open();
});
</script>
The options object
The following are properties you can use as part of the options object for use with the ModalDialog class:
Property | Data type | Default Value | Description |
---|---|---|---|
content | Mixed (String or DOM element) | Undefined | Customizable content that appears in the modal |
description | String | Undefined | A text description for the modal, primarily for accessibility |
fillAlways | Boolean | true | Normally, modals are automatically filled only the first time they open; this option tells the modal to refresh its content every time it opens |
label | String | Undefined | A text label for the modal, primarily for accessibility |
temporary | Boolean | true | If true the modal can only be opened once; it will be disposed as soon as it's closed |
uncloseable | Boolean | false | If true the user will not be able to close the modal through the UI in the normal ways; programmatic closing is still possible |
The previous code sample shows using the options
object to set and use the content
and label
properties with a ModalDialog.
Methods
The following are methods that are part of the ModalDialog class:
Method | Description |
---|---|
close() | Closes the modal |
closeable() | Returns a Boolean reflecting if the modal is closeable or not |
description() | Returns the description string for this modal; primarily used for accessibility |
empty() | Empties the content element; this happens automatically anytime the modal is filled |
fill() | Fill the modal's content element with the modal's content option; the content element will be emptied before this change takes place |
label() | Returns the label string for this modal; primarily used for accessibility |
open() | Opens the modal |
opened() | Returns a Boolean reflecting if the modal is opened currently |
Using HTML content
Up to this point, the content displayed in the modal has been simple strings. If you wish to use HTML elements as content you need to take a slightly different approach.
The key to using HTML as content is that you need to create some HTML element you will later assign to the content. In the code below, JavaScript's createElement()
method is used to create a container element in which you can place other HTML. The dynamically created element is then assigned to the content
property. The code and screenshot of the result both are shown.
//Create a div in which to place HTML content
var newElement = document.createElement('div');
//Place data in div
newElement.innerHTML = "<p style='font-size: 1em;'>this is in a paragraph</p><ul><li>in a list</li></ul>";
//Assign element to content
options.content = newElement;
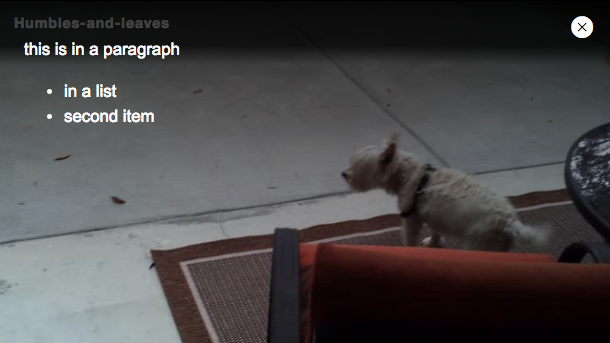
Styling the content
By default the text displayed in the modal is white and located on the top-left, as shown here:
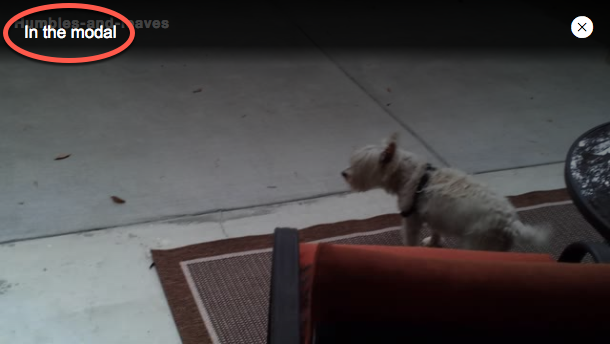
You can change location and style of the text by using CSS. To do this you use can add a class to the ModalDialog and create a class selector and style as you choose. The process to style the modal follows these steps:
- Programmatically add a class to the modal; in this document
.vjs-my-custom-modal
is used, but you can name this class whatever you wish - Create the style using the newly added class and the class that is automatically a child of the modal; this class is
.vjs-modal-dialog-content
, for example:.vjs-my-custom-modal .vjs-modal-dialog-content { color: red; margin-top: 40px; margin-left: 40px; }
The style shown above would appear as follows:
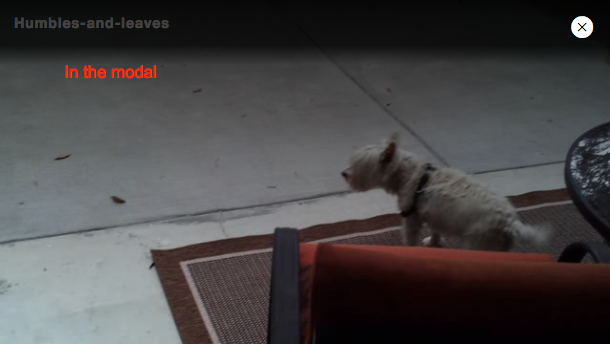
Here is the code to use a style with the createModal()
helper function:
<head>
<meta charset="UTF-8">
<title>Untitled Document</title>
<style>
.video-js {
height: 344px;
width: 610px;
}
.vjs-my-custom-modal .vjs-modal-dialog-content {
color: red;
margin-top: 40px;
margin-left: 40px;
}
</style>
</head>
<body>
<video-js id="myPlayerID"
data-video-id="5079788144001"
data-account="1507807800001"
data-player="HJLWns1Zbx"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/HJLWns1Zbx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
const myModal = myPlayer.createModal('In the modal');
myModal.addClass('vjs-my-custom-modal');
});
</script>
<head>
<meta charset="UTF-8">
<title>Untitled Document</title>
<style>
.video-js {
height: 344px;
width: 610px;
}
.vjs-my-custom-modal .vjs-modal-dialog-content {
color: red;
margin-top: 40px;
margin-left: 40px;
}
</style>
</head>
<body>
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="HJLWns1Zbx"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/HJLWns1Zbx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
const myModal = myPlayer.createModal('In the modal');
myModal.addClass('vjs-my-custom-modal');
});
</script>
Here is the complete code to use a style with the ModalDialog
constructor function:
<head>
<meta charset="UTF-8">
<title>Untitled Document</title>
<style>
.video-js {
height: 344px;
width: 610px;
}
.vjs-my-custom-modal .vjs-modal-dialog-content {
color: red;
margin-top: 40px;
margin-left: 40px;
}
</style>
</head>
<body>
<video-js id="myPlayerID"
data-video-id="5079788144001"
data-account="1507807800001"
data-player="HJLWns1Zbx"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/HJLWns1Zbx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.content = 'In the modal';
options.label = 'the label';
var ModalDialog = videojs.getComponent('ModalDialog');
var myModal = new ModalDialog(myPlayer, options);
myModal.addClass('vjs-my-custom-modal');
myPlayer.addChild(myModal);
myModal.open();
});
</script>
<head>
<meta charset="UTF-8">
<title>Untitled Document</title>
<style>
.video-js {
height: 344px;
width: 610px;
}
.vjs-my-custom-modal .vjs-modal-dialog-content {
color: red;
margin-top: 40px;
margin-left: 40px;
}
</style>
</head>
<body>
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="HJLWns1Zbx"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/HJLWns1Zbx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.content = 'In the modal';
options.label = 'the label';
var ModalDialog = videojs.getComponent('ModalDialog');
var myModal = new ModalDialog(myPlayer, options);
myModal.addClass('vjs-my-custom-modal');
myPlayer.addChild(myModal);
myModal.open();
});
</script>