Domain Restrictions Messaging
Overview
It is possible to restrict where a video can be viewed via domain restrictions. The Configuring Player Content Restrictions document shows you how to use Video Cloud to apply domain restrictions. The document you are now reading instructs you how to implement code to inform users that the player is using domain restrictions.
By default, Brightcove Player does not inform the user that a video they are trying to view is in a domain restricted player. The player simply displays a black screen. If you view the console you will see the following.

This is not terribly helpful, but if you check the Network tab and click a Name that corresponds to your video ID then check the Preview tab, you will see a more illuminating error.
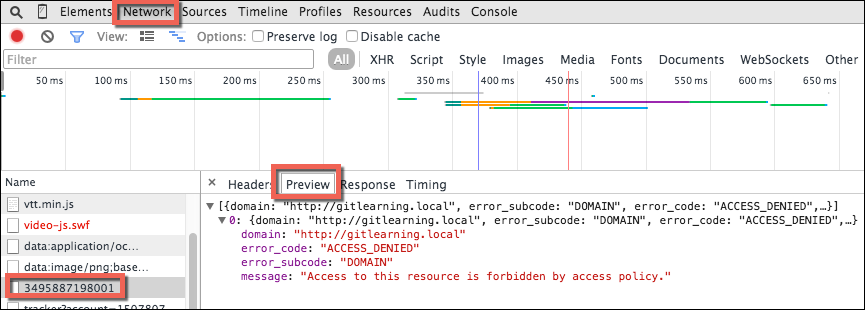
This document implements a strategy to let the user know the video they are trying to watch is domain restricted.
Player catalog's error object
The key to creating a user friendly message is the error
object contained in Brightcove Player's catalog
. An example of a domain restriction error is shown below in a screenshot from a browser's console. Notice that the object contains a data
property, which itself contains an array. The array will hold the last error the catalog encountered loading a video. In the example you see the element in the array is reporting a domain restriction issue.

Handle bc-catalog-error
It is possible that handling errors in the normal ready()
section in the script
block can cause issues. For instance, it can happen that the bc-catalog-error
event could be dispatched before the player is ready, and if you listen for the error in the ready()
section, you will not be able to handle the error. You may find that there is not a problem in your code, but the issue can browser dependent, so use caution. For this reason, the error handling code in this document will use the one()
event handling method to listen for the bc-catalog-error
in a separate code block rather than inside the ready()
section.
Message in HTML
Once you understand the structure of the error that is produced, and where it is located, you can use JavaScript to display a descriptive error for the user. The basic implementation steps are:
- Create a target element in which to display the message.
- Be sure the player is ready to act upon.
- Check to see if any error has occurred.
- If yes, check to see if the error is a domain restriction issue.
- If yes, display message to user.
The following code implements these steps.
- Line 8: Insert an HTML element, in this case a paragraph, in which to inject text; give the element an
id
for targeting purposes. - Line 13: Use the
one()
method add an event listener for thebc-catalog-error
event only once. - Line 16: Use an
if
statement to be sure some error has occurred. - Line 17: Assign a variable the specific error information from Brightcove Player catalog's error object.
- Line 18: Use an
if
statement to be sure the specific error information exists AND the type of error is from domain restriction. - Line 19: Inject an appropriate text message into the HTML element created at line 12.
<video-js id="myPlayerID"
data-video-id="3495887198001"
data-account="1507807800001"
data-player="4c8eb732-ba76-485c-a906-407365721633"
data-embed="default"
controls=""></video-js>
<p id="textTarget"></p>
<script src="https://players.brightcove.net/1507807800001/4c8eb732-ba76-485c-a906-407365721633_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function(){
var myPlayer = this,
specificError;
if (myPlayer.catalog.error !== undefined) {
specificError = myPlayer.catalog.error.data[0];
if (specificError !== undefined & specificError.error_subcode == "DOMAIN") {
document.getElementById("textTarget").innerHTML = "The video you are trying to watch cannot be viewed as it is domain restricted.";
};
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
console.log('in ready');
});
</script>
The following screenshot shows the message displayed below the player.
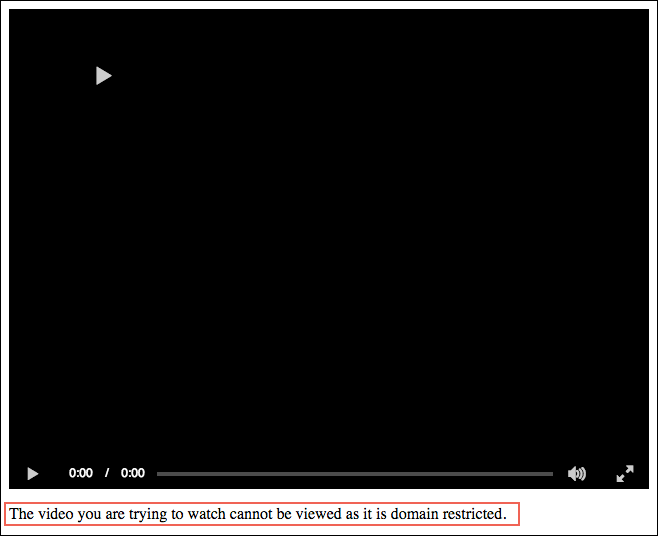
Message via errors plugin
You may wish to have the message displayed in the player if the video is domain restricted. You can do this utilizing the errors plugin. The errors plugin is automatically loaded into the player, so you do not need to explicitly perform that operation. For details on the errors plugin beyond what will be mentioned here, see the Display Error Messages Plugin document.
- Line 43: Use the
one()
method add an event listener for thebc-catalog-error
event only once. - Lines 46-53: Call the player's
error()
method, passing in as an argument JSON that defines theerrors
information to display. Note that the choice of-3
for the error code is arbitrary, and the only guidance is not to use a standard error code (currently 1-5). - Line 57: Call the
error()
method to display the custom error information. The logic used to determine when the domain restriction error has occurred is the same as above. The object passed as an argument defines which error message to display.
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function(){
var myPlayer = this,
specificError;
myPlayer.errors({
'errors': {
'-3': {
'headline': 'The video you are trying to watch cannot be viewed because of domain restrictions.',
'type': 'DOMAIN'
}
}
});
if (typeof(myPlayer.catalog.error) !== 'undefined') {
specificError = myPlayer.catalog.error.data[0];
if (specificError !== 'undefined' & specificError.error_subcode == "DOMAIN") {
myPlayer.error({code:'-3'});
};
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
});
</script>
The following screenshot shows the error plugin's display of the defined error.
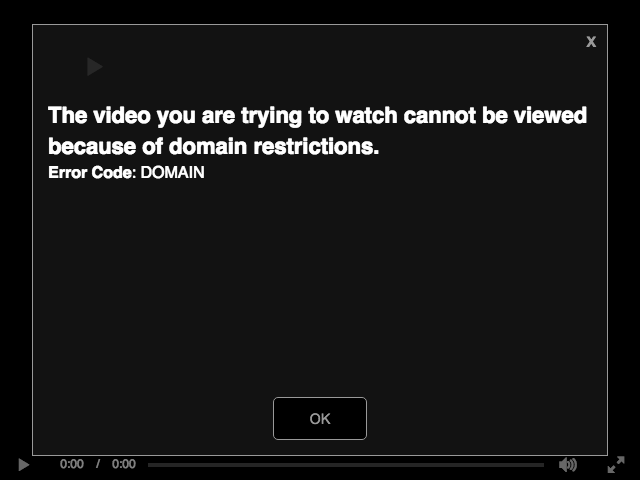
General error display
The two sections above deal specifically with displaying the error generated when a geo-restricted video is viewed from a restricted locale. Some errors do not provide the level of detail the domain restriction does. An example error object of this type is shown here. Notice that the data field is empty, whereas with the domain restriction error it contained key error information.
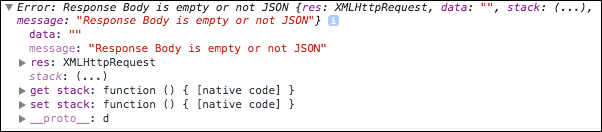
The following code shows how to display information from catalog.error
no matter the level of detail. At a high-level, the code performs the following:
- Checks if a catalog error has occurred.
- Checks if in the error object the
data
field contains specific error information. If it does NOT, the general error message is injected into the HTML. - If there is specific error information AND it is domain restriction related an appropriate message is injected into the HTML.
- If there is specific error information, and it is not domain restriction related, the specific error's message is injected into the HTML.
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function(){
var myPlayer = this,
specificError;
if (myPlayer.catalog.error != undefined) {
specificError = myPlayer.catalog.error.data[0];
if (specificError == undefined) {
document.getElementById("textTarget").innerHTML = "The following error has occurred: <strong>" + myPlayer.catalog.error.message + "</strong>";
} else if (specificError.error_subcode == "DOMAIN") {
document.getElementById("textTarget").innerHTML = "The video you are trying to watch cannot be viewed because of domain restrictions.";
} else {
document.getElementById("textTarget").innerHTML = "The following error has occurred: " + specificError.message;
};
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
});
</script>
The following screenshot shows the general error message being displayed in HTML. Of course you could also use the errors plugin to display the message, as shown previous section of this document.
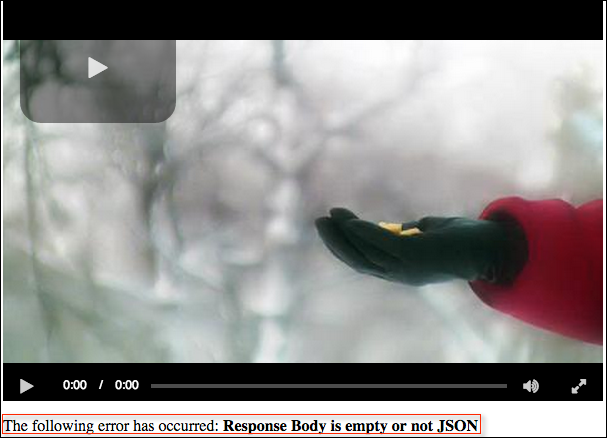