What is Brightcove Player debug mode?
When used, player debug mode causes players and plugins to show parts of their logs in the console to help in troubleshooting issues. Currently not every piece of the player interacts with debug mode, but more will in the future.
When debug mode is on, the player's Video.js logging level will be set to debug and logging history tracking will be enabled. When debug mode is off, the player's logging level will be set to off
and logging history tracking will be disabled.
The debugging info is displayed in the console. Here is a sample of some debugging segments with info on proxy-tracks, IMA3 ads and seek events:
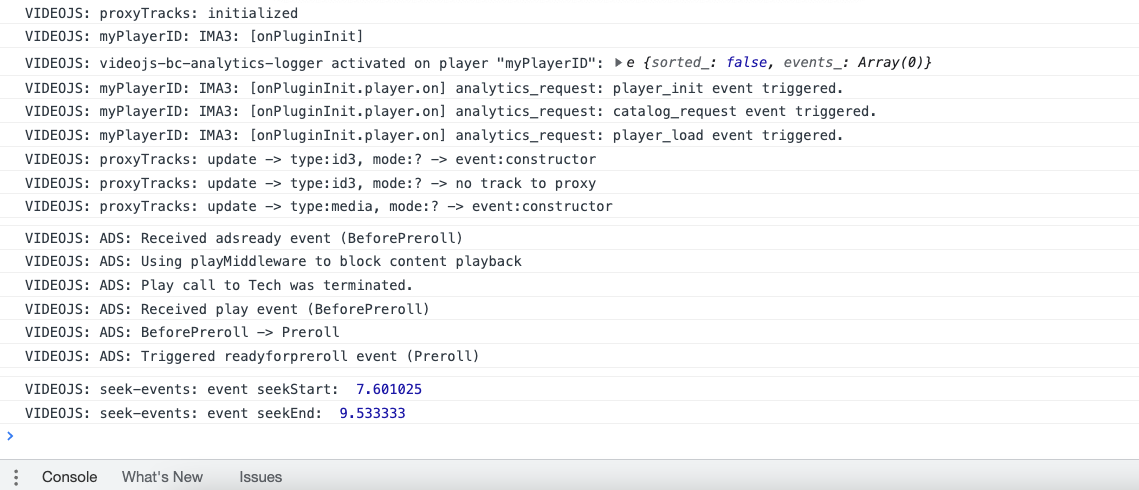
There are a number of ways to turn debug mode on and off, detailed next in this document.
Enabling debug mode
There are four ways to enable debug mode:
- Using the player configuration
- Using query parameters in player standard code
- Using the
data-debug
attribute in player advanced code - Manual option using the
bc()
method
Player configuration
In Studio, you can edit a player and in the JSON Editor you can add "debug": true,
to enable debug mode.
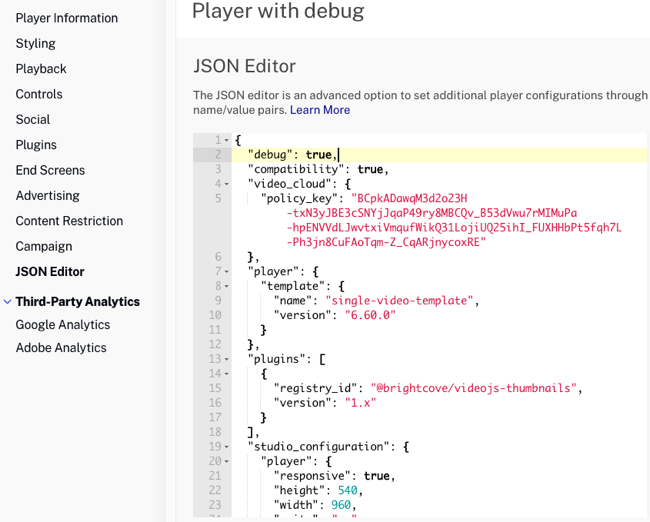
After adding the line of JSON, be sure to save and publish the player.
Query parameters
If you are using the Standard player code (iframe), you can use a query parameter to enable debug mode. You add the query parameter ?debug
(highlighted )as shown here:
<iframe src="https://players.brightcove.net/.../index.html?debug"></iframe>
Attribute
If you are using the Advanced player code, you can use an attribute to enable debug mode. You add the attribute data-debug
(highlighted) as shown here:
<video-js id="myPlayerID"
data-debug
data-account="1507807800001"
data-player="5qbwL2GCf"
...></video-js>
<script src="https://players.brightcove.net/1507807800001/5qbwL2GCf_default/index.min.js">
Manual with bc()
method
When manually calling the bc()
function, debug
can be passed as an option, as shown here:
const player = bc('example', {debug: true});
Controlling player debug mode at runtime
If you choose to control debug at runtime, you can do this using the player.debug()
method. Here is some code that allows debug mode to be turned off and on.
...
<button onclick="toggleDebug()">Toggle Debug Mode</button>
...
<script>
var myPlayer;
videojs.getPlayer('myPlayerID').ready(function () {
myPlayer = this;
});
function toggleDebug() {
if (myPlayer.debug()) {
myPlayer.debug(false);
} else {
myPlayer.debug(true);
}
console.log('debug state: ', myPlayer.debug());
}
</script>
Debug mode events
When debug mode changes, the appropriate event is dispatched:
- debugon
- debugoff
Here is the code from above, but this time with event handlers added:
...
<button onclick="toggleDebug()">Toggle Debug Mode</button>
...
<script>
var myPlayer;
videojs.getPlayer('myPlayerID').ready(function () {
myPlayer = this;
myPlayer.on('debugon', function () { console.log('dispatch debugon') });
myPlayer.on('debugoff', function () { console.log('dispatch debugoff') });
});
function toggleDebug() {
if (myPlayer.debug()) {
myPlayer.debug(false);
} else {
myPlayer.debug(true);
}
console.log('debug state: ', myPlayer.debug());
}
</script>