Overview
This plugin enables FreeWheel ad technology for HTML5 in Brightcove Player.
Using versions of the plugin
When using the FreeWheel plugin you will need to use versioned URLs.
Version | Type | Versioned URL |
---|---|---|
Latest Release | JS | https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.js |
CSS | https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.css |
For reference, the versions of the plugin are tracked in the Plugin Version Reference document.
Testing the ad server
The first thing you should do is verify the validity of the ad tag you are planning to use. Be sure you have copied its URL and browse to the following page: Ad Previewer (clicking this link will cause the page to open in a new window or tab).
Paste your ad tag URL into the indicated form input field. Click OPEN IN AD PREVIEWER. A popup titled Open In Ad Previewer will display, and you then click the OPEN button to test your ad. Click PLAY and, if functioning correctly, your ad will display interspersed with a video. If your ad tag is not working in this test environment, it will not work with Brightcove Player.
Using the correct SDK URL
The next two sections provide alternatives to implementing FreeWheel. In both sections you will use a sdkurl
. In the past you could simply use http://adm.fwmrm.net/p/[]/AdManager.js
for the value of the option, and know it would point to the latest version of the FreeWheel SDK. Now you will need to enter a URL pointing to a specific version, like https://mssl.fwmrm.net/libs/adm/6.24.0/AdManager.js
. In addition, after initial setup, you will also need to update that value when a newer version of the SDK is released by FreeWheel, if you wish to be on the latest version. Note that after making this change, you will need to re-publish your Brightcove Player.
If you do not update the URL and continue to use the auto upgrade URL, you will forever be pointing to version 6.23.
You may want to manage your own SDK version in their Brightcove Player integration. This involves passing the Html5.sdkurl
option to the FreeWheel plugin:
player.FreeWheelPlugin({
Html5: {
sdkurl: 'preferred-sdk-url'
}
});
Consent/GDPR support
In Freewheel's SDK version 6.28.x, they added two new fields to the ad request: _fw_gdpr
and _fw_gdpr_consent
. Using 6.28.x, you had to pass these values to Freewheel via API.
In SDK version 6.30.0, all you have to do is include the link to your specific CMP (consent management platform) implementation script you are using and as of Freewheel version 6.30.0, the SDK does the rest. The SDK checks if a CMP script exists, whether the you are in a GDPR-protected country, and/or requires consent. It then calls the appropriate API to set the _fw_gdpr
and _fw_gdpr_consent
flags.
Steps
-
Include the link to the CMP script in the page where the player is embedded
Example
<script src="//your_cmp_address.net/cmp/cmp.complete.bundle.js" async></script>
-
Make sure you are using version 6.30.0+ of the Freewheel SDK by setting the
sdkurl
property in your Freewheel configuration to 6.30 or later:Example
https://mssl.fwmrm.net/libs/adm/6.30.0/AdManager.js
Implement using Players module
To implement the FreeWheel Plugin using the Players module, follow these steps:
- Open the PLAYERS module and either create a new player or locate the player to which you wish to add the plugin.
- Click the link for the player to open the player's properties.
- Click Plugins in the left navigation menu.
- From the Add a Plugin dropdown, select Custom Plugin.
- For the Plugin Name enter
FreeWheelPlugin
. - For the JavaScript URL, enter:
https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.js
- For the CSS URL, enter:
https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.css
- Enter the configuration options in the Options(JSON) text box. The options provided in the form configure playback technology where
Flash
andHtml5
configuration sections are provided by FreeWheel. A slightly altered (for security reasons) complete configuration is shown below . A stub example is shown here:{ "Html5": { "sdkurl": "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...", "serverUrl": "http://cue.v.fwmrm.net/..." "profile": "3aaa_profile", ... } }
- Click Save.
- To publish the player, click Publish & Embed > Publish Changes.
- To close the open dialog, click Close.
Implement using code
To implement a plugin the player needs to know the location of the plugin code, a stylesheet if needed, the plugin name and plugin configuration options. The location of the plugin code and stylesheet are as follows:
https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.js
https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.css
The name of the plugin is FreeWheelPlugin
. The options configure playback technology where Flash
and Html5
configuration sections are provided by FreeWheel. A slightly altered (for security reasons) complete configuration is shown below . A stub example is shown here:
{
Html5: {
sdkurl: "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...",
serverUrl: "http://cue.v.fwmrm.net/..."
profile: "3aaa_profile",
...
}
The following shows using the In-Page Embed implementation of the player to associate the FreeWheel Plugin with a single instance of a player.
- Line 90: Uses a
link
tag to include the plugin's CSS in thehead
of the HTML page. - Line 92: Gives the
video
tag anid
attribute, with some value, in this case myPlayerID. - Line 100: Uses a
script
tag to include the plugin's JavaScript in thebody
of the HTML page. - Lines 105-125: Initialize the plugin and pass in custom options.
- Line 107: Creates a reference to the player.
<link href="https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.css" rel="stylesheet">
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="default"
data-embed="default"
controls=""
data-video-id="4607746980001"
data-playlist-id=""
data-application-id=""
width="960" height="540"></video-js>
<script src="https://players.brightcove.net/1507807800001/default_default/index.min.js"></script>
<script src="https://players.brightcove.net/videojs-freewheel/3/videojs-freewheel.min.js"></script>
<script>
var myPlayer;
videojs.getPlayer('myPlayerID').ready(function() {
// get a reference to the player
myPlayer = this;
// initialize the FreeWheel plugin
myPlayer.FreeWheelPlugin({
Html5: {
sdkurl: "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...",
serverUrl: "http://cue.v.fwmrm.net/..."
profile: "3aaa_profile",
...
}
});
});
</script>
Configuration
The following settings are used to configure the FreeWheel plugin:
adTechOrder
:- Type:
Array
Default Value:["html5"]
- The array contains the ad integration technologies to attempt to use, in order of descending precedence.
- Type:
- Flash:
- Type:
Object
- An object for Tech specific FreeWheel configuration (provided by FreeWheel). The object contains the following options:
swfurl
:- Type:
string
Default Value:https://players.brightcove.net/videojs-freewheel/3/videojs.freewheel.swf
(compatible with FW Flash SDK v6.4.6) - Optional values can be used based on FreeWheel Flash Plugin Version. Those values can be found in the Using versions of the plugin section near the top of this document.
- Must be a child property of the
Flash
object. This will be directly applied to the object element if the Flash ad tech is used.
- Type:
autoplay
:- Type:
boolean
Default Value:true
- Indicates if the content is autoplayed.
- Must be a child property of the
Flash
object. This will be directly applied to the object element if the Flash ad tech is used.
- Type:
unattendedPlay
:- Type:
boolean
Default Value:false
- When
autoPlay
is set to true,unattendedPlay
indicates whether the user is aware of the autoplaying. - Must be a child property of the
Flash
object. This will be directly applied to the object element if the Flash ad tech is used.
- Type:
visitorCustomId
:- Type:
string
Default Value:unset
- Allows publishers to pass a custom visitor id for the ad request. When set, this value will be passed in the visitor block of the request payload as customId="CustomID_001". The id will be returned in the response to the ad request in the opening visitor tag as an attribute: customId="CustomID_001".
- Must be a child property of the
Flash
object. This will be directly applied to the object element if the Flash ad tech is used.
- Type:
- Type:
Html5
:- Type:
Object
- An object for Tech specific FreeWheel configuration (provided by FreeWheel). The object contains the following options:
autoPlayType
:- Type:
integer
Default Value:1
- Indicates that the content video will autoPlay and the user is aware the content is autoplaying.
- The values passed into autoPlayType sets flags in the ad request url sent to the FreeWheel ad server and can be one of three integer values which equate to the related FreeWheel SDK constants:
- 1: tv.freewheel.SDK.VIDEO_ASSET_AUTO_PLAY_TYPE_ATTENDED: Means
autoPlay
is true andunattendedPlay
is false. This sets flag = +play-uapl. - 2: tv.freewheel.SDK.VIDEO_ASSET_AUTO_PLAY_TYPE_UNATTENDED: means
autoPlay
is true andunattendedPlay
is also true. This sets flag = +play+uapl. - 3: tv.freewheel.SDK.VIDEO_ASSET_AUTO_PLAY_TYPE_NONE: means
autoPlay
is false andunattendedPlay
is unset. This sets flag = -play.
- 1: tv.freewheel.SDK.VIDEO_ASSET_AUTO_PLAY_TYPE_ATTENDED: Means
- Must be a child property of the
Html5
object. This will be directly applied to the object element if the Html5 ad tech is used.
- Type:
visitorCustomId
:- Type:
string
Default Value:(optional)
- The
visitorCustomId
option allows publishers to pass a custom visitor id for the ad request. When set, this value will be passed in the ad request URL as the queryString vcid=CustomID_001. The id will be returned in response of the ad request in the visitor JSON block as "customId" : "CustomID_001". - Must be a child property of the
Html5
object. This will be directly applied to the object element if the Html5 ad tech is used.
- Type:
subsessionToken
:- Type:
int
Default Value:(optional)
- The
subsessionToken
option allows publishers to start a subsession with the given token. When set, this value will be passed in the ad request URL as the queryString:ssto=1234567890
- Must be a child property of the
Html5
object. This will be directly applied to the object element if the Html5 ad tech is used.
- Type:
- compatibleDimensions:
- Type:
array
Default Value:(optional)
-
The
compatibleDimensions
option allows publishers to pass an array of compatible dimensions. For example:compatibleDimensions: [ {width: A, height: B}, {width: X, height: Y} ]
When set, this value will be passed in the ad request URL as the queryString:
cd=widthA,heightA|widthX,heightY
- Must be a child property of the
Html5
object. This will be directly applied to the object element if the Html5 ad tech is used.
- Type:
- Type:
-
videoAssetFallbackId
- Type:
string
Default Value: none - Video asset fallback id. A FreeWheel video asset id when the video asset id in ad request is not recognized. Note this needs to be a "FreeWheel id", which is all digital. It is not a custom id.
- Type:
-
siteSectionFallbackId
- Type:
string
Default Value: none - Site section fallback id. A FreeWheel site section id when the site section id in ad request is not recognized. Note this needs to be a "FreeWheel id", which is all digital. It is not a custom id.
- Type:
loadWithCookie
:- Type:
boolean
Default Value:false
- Enables or disables cookies for VAST requests.
- Type:
requestAdsMode
:- Type:
string
Default Value:onload
- Possible values for the property are:
onload
: Ads are requested when the player is completely loaded.onplay
: Ads are requested when the video starts to play, either user initiated or autoplay.oncue
: Ads are inserted at each cue point, according to a request made at startup. When using this property, also note:- Only functional when the
useMediaCuePoints
property is also set totrue
. - Video Cloud ad cue points must be set up on content video. See the Working with Cue Points in the Media Module document for instructions on how to create cue points in the content video.
- If there is a
temporalSlots
option under theFlash
orHtml5
properties, the setting is ignored.
- Only functional when the
- Type:
- useMediaCuePoints:
- Type:
boolean
Default Value:false
- Must be set to true to use Video Cloud ad cue points to trigger ads. Must be used in conjunction with
requestAdsMode: 'oncue'
property and assigned value.
- Type:
Ad macros
There are ad macros available to make your job easier when configuring FreeWheel. The ad macros are replaced with corresponding values anywhere in your configuration.
The following is the complete list of variable for which substituted values will be used:
Macro | Description |
---|---|
{player.id} | Player ID |
{mediainfo.id} | Video ID |
{mediainfo.name} | Video title |
{mediainfo.description} | Short description (250 chars max) |
{mediainfo.tags} | Tags (metadata) associated with the video |
{mediainfo.reference_id} | Reference ID |
{mediainfo.duration} | Duration of the video as reported by Video Cloud |
{mediainfo.ad_keys} | Free form text string that can be added and edited in the Media module of Studio; you should use the query parameter in the form
|
{player.duration} | Duration of video as measured by player (possibly slightly different from mediainfo.duration and probably more accurate) |
{document.referrer} | Referring page URL |
{window.location.href} | Current page URL |
{player.url} | URL of the player |
{timestamp} | Current local time in milliseconds since 1/1/70 |
{random} | A random number 0-1 trillion |
Example configuration
"plugins": [{
"name": "FreeWheelPlugin",
"options": {
"Html5": {
"keyValues": [{
"feature": "simpleAds",
"module": "DemoPlayer"
}, {
"feature": "trackingURLs"
}],
"networkId": 99999,
"profile": "global-js",
"sdkurl": "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...",
"serverUrl": "http://cue.v.fwmrm.net/ad/h/5",
"siteSectionCustomId": "your value here",
"temporalSlots": [{
"adUnit": "preroll",
"id": "Preroll_1",
"timePosition": 0
}, {
"adUnit": "postroll",
"id": "Postroll_1",
"timePosition": 60
}, {
"adUnit": "overlay",
"id": "Overlay_1",
"timePosition": 5
}],
"videoAssetCustomId": "your value here",
"videoAssetDuration": 500
},
"debug": true,
"prerollTimeout": 1000,
"timeout": 5000
}
}]
Events
The plugin emits some custom event types during loading, initialization, and playback. You can listen for the ad framework events just like you would any other event:
player.on('ads-ad-started', function(event) {
console.log('event', event);
});
Event | Dispatched when: |
---|---|
ads-request | Upon request ad data. |
ads-load | When ad data is available following an ad request. |
ads-ad-started | An ad has started playing. |
ads-ad-ended | An ad has finished playing. |
ads-pause | An ad is paused. |
ads-play | An ad is resumed from a pause. |
ads-first-quartile | The ad has played 25% of its total duration. |
ads-midpoint | The ad has played 50% of its total duration. |
ads-third-quartile | The ad has played 75% of its total duration. |
ads-click | A viewer clicked on the playing ad. |
ads-volumechange | The volume of the playing ad has been changed. |
ads-pod-started | The first ad in a linear ad pod (a sequenced group of ads) has started. |
ads-pod-ended | The last ad in a linear ad pod (a sequenced group of ads) has finished. |
ads-allpods-completed | All linear ads have finished playing. |
fw-before-ad-request | This event is exposed on the player object and is triggered before submitting an ad request. Usually it is used in the context of playlists, to make updates to the FreeWheel Configuration settings via: player.FreeWheelPlugin.settings.Html5 or player.FreeWheelPlugin.settings.Flash |
Dynamic server URL assignment
You can use the fw-before-ad-request
event to dynamically assign the server URL. You will use the on()
method to listen for the ad request, then assign the desired server URL. Of course, you will need to supply your desired server URLs for the placeholders in the code:
player.on('fw-before-ad-request', function () {
player.FreeWheelPlugin.settings.Html5.serverUrl = '[your server url]'
player.FreeWheelPlugin.settings.Flash.serverUrl = '[your server url]'
})
If you have configured the server URLs previously, the code shown will override the previous configuration.
Demos
This demo will play a pre-roll, a mid-roll at 5 seconds and then a post-roll. The adTechOrder
is set for html5
first, as shown here:
"adTechOrder": [
"html5",
"flash"
],
adtech order: html5, flash
This demo uses html5
first in the adtechOder
.
Following is the actual configuration used with the player. Note that this can be used for the options value in Studio.
{
"Flash": {
"networkId": 90750,
"profile": "3pqa_profile",
"sdkurl": "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...",
"serverUrl": "//cue.v.fwmrm.net/ad/g/1",
"siteSectionCustomId": "your value here",
"swfurl": "https://players.brightcove.net/videojs-freewheel/3/videojs.freewheel.swf",
"temporalSlots": [{
"adUnit": "preroll",
"id": "Preroll_1",
"timePosition": 0
}, {
"adUnit": "postroll",
"id": "Postroll_1",
"timePosition": 60
}, {
"adUnit": "midroll",
"id": "Midroll_1",
"timePosition": 5
}],
"videoAssetCustomId": "your value here",
"videoAssetDuration": 500
},
"Html5": {
"keyValues": [{
"feature": "simpleAds",
"module": "DemoPlayer"
}, {
"feature": "trackingURLs"
}],
"networkId": 96749,
"profile": "global-js",
"sdkurl": "https://mssl.fwmrm.net/libs/adm/{LATEST_VERSION}/...",
"serverUrl": "//demo.v.fwmrm.net/ad/g/1",
"siteSectionCustomId": "your value here",
"temporalSlots": [{
"adUnit": "preroll",
"id": "Preroll_1",
"timePosition": 0
}, {
"adUnit": "postroll",
"id": "Postroll_1",
"timePosition": 60
}, {
"adUnit": "midroll",
"id": "Midroll_1",
"timePosition": 5
}],
"videoAssetCustomId": "your value here",
"videoAssetDuration": 500
},
"adTechOrder": [
"html5",
"flash"
],
"debug": true,
"prerollTimeout": 1000,
"timeout": 5000
}
Playlist
This demo uses the HTML first configuration with a playlist. You will see for each video in the playlist a pre-roll, a mid-roll at the 5 second mark, and a post-roll.
Player Ad Libraries
The videojs/videojs-contrib-ads GitHub repository contains a plugin which provides common functionality needed by video advertisement libraries working with Brightcove Player. The plugin provides common functionality needed by video ad integrations and takes care of a number of concerns for ad integrators, reducing the code you have to write for your ad integration.
Properties
videojs-contrib-ads provides some properties that can be helpful. They are:
Name | Data Type | Description |
---|---|---|
ads.ad.id | String | Unique identifier for an ad that plays |
ads.ad.index | Number | The index of the ad that plays at a specified time; the index would identify the ordinal value of an ad in an ad pod |
ads.ad.duration | Number | The duration of the ad in seconds |
ads.ad.type | String | Either PREROLL, MIDROLL or POSTROLL |
ads.ad.currentTime() | Function | A function that returns the current time of ad playback |
The following code demonstrates the use of the properties:
myPlayer.on('ads-ad-started',function( evt ){
console.log('*****ads-ad-started event passed to event handler', evt);
console.log('myPlayer.ads.ad.id',myPlayer.ads.ad.id);
console.log('myPlayer.ads.ad.index',myPlayer.ads.ad.index);
console.log('myPlayer.ads.ad.duration',myPlayer.ads.ad.duration);
console.log('myPlayer.ads.ad.type',myPlayer.ads.ad.type);
setTimeout(function(){
console.log('****myPlayer.ads.ad.currentTime()',myPlayer.ads.ad.currentTime());
},500);
setTimeout(function(){
console.log('****myPlayer.ads.ad.currentTime()',myPlayer.ads.ad.currentTime());
},1000);
});
The output in the console from the above code is shown here:
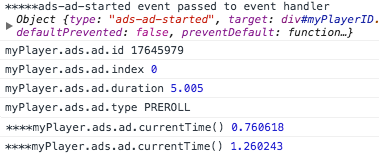
Methods
videojs-contrib-ads provides some methods that can be helpful. They are:
Method | Description |
---|---|
inAdBreak() | This method returns true during the time between startLinearAdMode and endLinearAdMode where an integration may play ads. This is part of ad mode. |
isAdPlaying() | Deprecated |
isContentResuming() | Returns true if content is resuming after an ad. This is part of ad mode. |
isInAdMode() | Returns true if the player is in ad mode. |
Ad parameters
Ad parameters can be used with a GET
request to the FreeWheel ad server.
Name | Parameter | Description |
---|---|---|
Video Asset Fallback ID | ad.afid |
Sets the video asset fallback ID (videoAsset@fallbackId ) included with the FreeWheel GET ad request. This ID is used when an ad request's video asset ID is not recognized.Example: ad.afid=AssetFallBackId
|
Known issues
Dynamically re-using the same player with FreeWheel
If you are loading videos dynamically and re-using the same player, the FreeWheel configuration settings will contain the same values as they were set at the time the FreeWheel plugin was instantiated, with two exceptions. The first exception is if you are using ad macros. The second exception is you can update the settings using player.FreeWheelPlugin.settings.Html5
or player.FreeWheelPlugin.settings.Flash
on an fw-before-ad-request
event.
Resizing player during ad playback
If the player is resized during ad or video playback, ad content will not resize unless the player's dimensions function is called to resize the player. Resizing the player using other methods (for example: style width and height) will not resize the ad.
When using player.dimensions(width,height)
to resize the player, you will have to trigger the fw-resizeplayer
event so that the plugin will know the dimensions have changed. This is because some VPAID ads retain the default dimensions of the ad and will not automatically resize when the player resizes.
Here is an example:
player.dimensions(960,540);
player.trigger('fw-resizeplayer');
Overlay ads
If the FreeWheel plugin is rendering an ad in Flash, overlay ads are not clickable. If the FreeWheel plugin is rendering an ad in HTML5, overlays are not displayed at correct coordinates. Clicking on an HTML5 overlay ad will not pause the content player when the click-url is followed.
FreeWheel Live
FreeWheel ads in a live stream can be implemented with assistance from Brightcove Global Services (BGS).
VPAID
It is not possible to resume ads via VPAID controls if the ad control bar is enabled.
Changelog
See the FreeWheel Plugin Release Notes.
For older releases, see the changelog here.