Overview
The following methods, events and configuration options are available as part of the API:
Category | Name | Description |
---|---|---|
Object | Playlist Item Object |
A playlist is an array of playlist items. |
Method | autoadvance() |
Determines if, and how long to wait before, the next video in the playlist is played. |
Method | contains() |
Checks if an item is in the playlist. |
Method | currentIndex() |
Retrieves index of the current item in the playlist. |
Method | currentItem() |
Assigns or retrieves the index for current video in the player. |
Method | first() |
Plays the first video in the playlist. |
Method | indexOf() |
Checks the presence of a video in the playlist, and returns its index. |
Method | last() |
Plays the last video in the playlist. |
Method | lastIndex() |
Retrieves the index of the last item in the playlist. |
Method | next() |
Plays the video following the video currently playing in the player. |
Method | nextIndex() |
Retrieves the index of the video following the video currently playing in the player. |
Method | playlist() |
Assigns or retrieves the current playlist associated with the player. |
Method | previous() |
Plays the video proceeding the video currently playing in the player. |
Method | previousIndex() |
Retrieves the index of the video proceeding the video currently playing in the player. |
Method | repeat() |
When all videos in the playlist have played, starts playing the playlist again. |
Method | reverse() |
Reverses the order of the videos in the playlist. |
Method | shuffle() |
Shuffles/randomizes the order of playlist items. |
Method | sort() |
Sorts the items in a playlist. |
Event | beforeplaylistitem |
Dispatched before switching to a new video within the playlist. |
Event | duringplaylistchange |
This event is fired after the contents of the playlist are changed when calling playlist() , but before the current playlist item is changed. |
Event | playlistchange |
Dispatched whenever the playlist associated with the player is changed. |
Event | playlistitem |
Dispatched when switching to a new video within a playlist. |
Event | playlistsorted |
Dispatched when any method is called that changes the order of playlist items. |
Configuration Option | playOnSelect |
Causes a clicked video from the playlist to start playing, even if the current video in the player was previously paused. |
Example
The following Brightcove Player has a playlist loaded and using the buttons you can move around in the playlist.
Playlist Item Object
A playlist item is an object with the following properties:Property | Type | Required | Description |
---|---|---|---|
sources | Array | Yes | An array of sources that video.js understands. |
poster | String | No | A poster image to display for these sources. |
textTracks | Array | No | An array of text tracks that Video.js understands. |
autoadvance( ) method
The player.playlist.autoadvance( )
method acts as a setter only, and sets playlist auto advancement. Once enabled it will wait the specified amount of seconds at the end of a video before proceeding automatically to the next video. Any value which is not a positive, finite integer will be treated as a request to cancel and reset the auto advancing. If you change the auto advance feature during a timeout period, the auto advance will be canceled and it will not advance to the next video, but it will use the new timeout value for the following videos.
Parameter:
- timeout: An integer number in seconds to delay before loading the next video in the playlist
Returned value:
The method does not return a value.
Example uses of the method follow:
player.playlist.autoadvance(0);
Will not wait before loading the next item
player.playlist.autoadvance(5);
Waits 5 seconds before loading the next item
player.playlist.autoadvance(null);
Resets and cancels the auto advance
contains( ) method
The player.playlist.contains( item )
method checks a playlist for the presence of the object in the item
parameter. If the item
is in the playlist true
is returned, if not present false
is returned.
Parameters:
- item: A value that represents a video element in the playlist.
Valid forms for the item parameter include:
- URL to a video as a string
'//media.w3.org/2010/05/sintel/trailer.mp4'
- Array containing an object with the source and type defined
[{ src: '//media.w3.org/2010/05/sintel/poster.png', type: 'image/png' }]
- Object containing an item from the sources array
{ sources: [{ src: '//media.w3.org/2010/05/sintel/trailer.mp4', type: 'video/mp4' }] }
- In Video Cloud, you can use the
currentSrc()
method with thecontains()
method to be sure the currently playing video is from the currently loaded playlist.myPlayer.playlist.contains(myPlayer.currentSrc());
Returned value:
The method returns an object of type boolean.
currentIndex( ) method
The player.playlist.currentIndex( )
method retrieves the index of the current item in the playlist. This is identical to calling currentItem()
with no arguments.
Parameter:
- None
Returned value:
The method returns a value of type number. The returned value represents the array position of the video currently playing in the player. If the player is currently playing a non-playlist video, currentIndex()
will return -1.
currentItem( ) method
The player.playlist.currentItem(index)
method acts as both a getter and setter, either retrieving the current index of the video from the playlist playing in the player, or assigning a new video to play in the player, based on the index. If called without an argument the method performs the retrieval and acts as a getter, with an argument is assigns the value and acts as a setter.
Parameter (setter):
- index: A number that represents the zero-based array index from the playlist of the video to be loaded into the player.
Returned value (getter):
The method returns a value of type number. The returned value represents the array position of the video currently playing in the player. If the player is currently playing a non-playlist video, currentItem()
will return -1.
An example use of the method in a function to jump to a specific video follows:
function goto2(){
myPlayer.playlist.currentItem(2);
}
first( ) method
The player.playlist.first()
method plays the first item in the playlist. The new video playing in the player will be returned from this method call. The currentItem
will be updated with the new index.
Parameters:
- None
Returned value:
The method returns an object of type object. An example of this object for a manually created playlist follows:

An example of this object for a Video Cloud playlist follows:
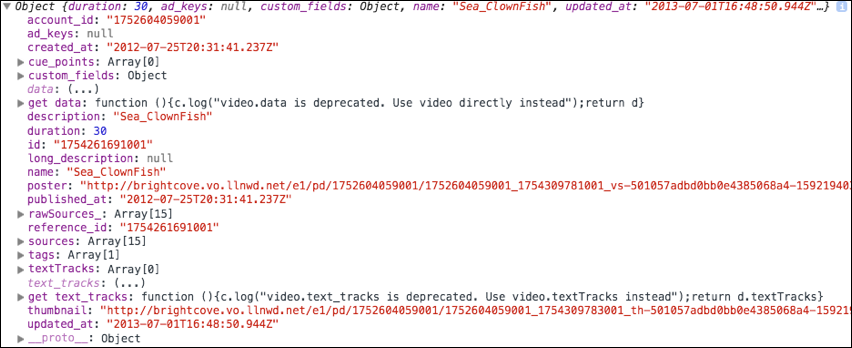
An example use of the method in a function to move to the next video follows:
function goToBeginning() {
var nextVidObject = myPlayer.playlist.first();
}
indexOf( ) method
The player.playlist.indexOf( item )
method checks the playlist for the presence of the object in the item
parameter, and if found, returns the zero-based index of the item. The method returns -1 if the item is not found.
Parameters:
- item: A value that represents a video element in the playlist.
Valid forms for the item parameter include:
- URL to a video as a string
'//media.w3.org/2010/05/sintel/trailer.mp4'
- Array containing an object with the source and type defined
[{ src: '//media.w3.org/2010/05/sintel/poster.png', type: 'image/png' }]
- Object containing an item from the sources array
{ sources: [{ src: '//media.w3.org/2010/05/sintel/trailer.mp4', type: 'video/mp4' }] }
- In Video Cloud you can use the
currentSrc()
method with theindexOf()
method to find the location of the currently playing video in the playlist.myPlayer.playlist.indexOf(myPlayer.currentSrc());
Returned value:
The method returns a value of type number. The value represents the zero-indexed position of the element, or -1 if the object is not found.
last( ) method
The player.playlist.last()
method plays the last item in the playlist. The new video playing in the player will be returned from this method call. The currentItem
will be updated with the new index.
Parameters:
- None
Returned value:
The method returns an object of type object. An example of this object for a manually created playlist follows:

An example of this object for a Video Cloud playlist follows:
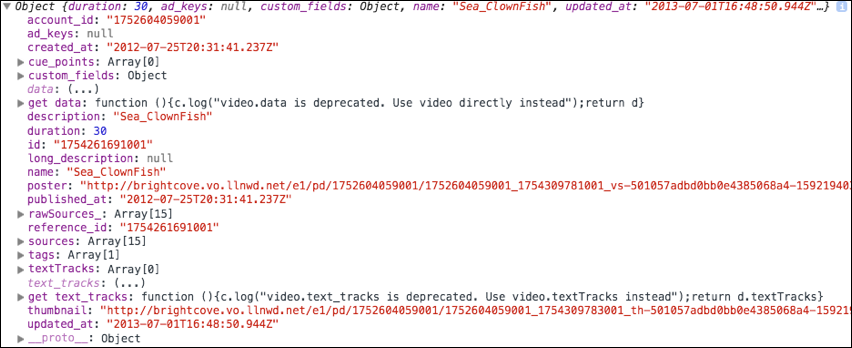
An example use of the method in a function to move to the next video follows:
function goToEnd() {
var nextVidObject = myPlayer.playlist.last();
}
lastIndex( ) method
The player.playlist.lastIndex( )
method returns the index of the last item in the playlist.
Parameter:
- None
Returned value:
The method returns a value of type number. The returned value represents the array position of the last video in the playlist.
next( ) method
The player.playlist.next()
method advances the player to the next item in the playlist. The new video playing in the player will be returned from this method call. The currentItem
will be updated with the new index. If you are at the end of the playlist, you will not be able to proceed past the end and nothing will be returned.
Parameters:
- None
Returned value:
The method returns an object of type object. An example of this object for a manually created playlist follows:

An example of this object for a Video Cloud playlist follows:
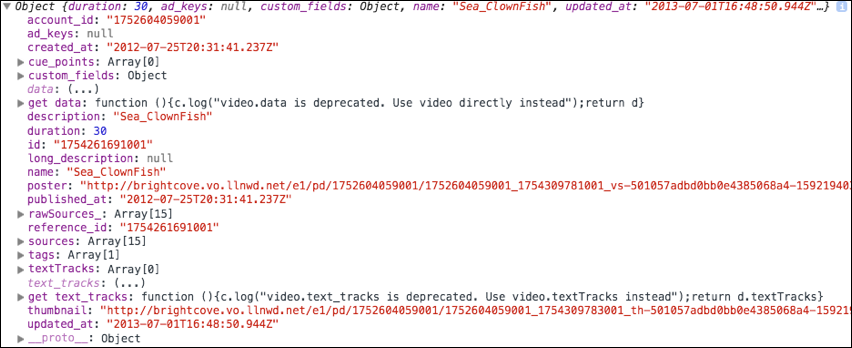
An example use of the method in a function to move to the next video follows:
function nextVideo() {
var nextVidObject = myPlayer.playlist.next();
}
nextIndex( ) method
The player.playlist.nextIndex( )
method returns the index of the next item in the playlist.
Parameter:
- None
Returned value:
The method returns a value of type number. The returned value represents the array position of the next video that will be played in the playlist.
If the player is on the last item, this method returns the last item's index. However, if the playlist repeats and is on the last item, the method returns 0. If the player is currently playing a non-playlist video, it will return -1.
playlist( ) method
The player.playlist(newPlayList)
method acts as both a getter and setter, either retrieving the playlist currently playing in the player, or assigning a playlist to the player to play. If called without an argument the method performs the retrieval and acts as a getter, with an argument is assigns the value and acts as a setter.
Parameter (setter):
- newPlaylist: An array of video sources/objects. The objects can either be manually created or simply a Video Cloud playlist ID or reference ID.
Although you can use the playlist()
method as a setter, it is a best practice to utilize Brightcove Player's catalog functionality to load a playlist, for example myPlayer.catalog.getPlaylist( playlistID )
and myPlayer.catalog.load( playlist )
. See the Player Catalog document for more information.
An example use of the method with a manually created playlist follows:
myPlayer = this;
myPlayer.playlist([{
"sources": [{
"src": "//solutions.brightcove.com/bcls/assets/videos/Sea_SeaHorse.mp4", "type": "video/mp4"
}],
"name": "Seahorse",
"thumbnail": "//solutions.brightcove.com/bcls/assets/images/Sea_Seahorse_poster.png",
"poster": "//solutions.brightcove.com/bcls/assets/images/Sea_Seahorse_poster.png"
}, {
"sources": [{
"src": "//solutions.brightcove.com/bcls/assets/videos/Sea_Anemone.mp4", "type": "video/mp4"
}],
"name": "Sea Anemone",
"thumbnail": "//solutions.brightcove.com/bcls/assets/images/Sea_Anemone_poster.png",
"poster": "//solutions.brightcove.com/bcls/assets/images/Sea_Anemone_poster.png"
}, {
"sources": [{
"src": "//solutions.brightcove.com/bcls/assets/videos/Tiger.mp4", "type": "video/mp4"
}],
"name": "Tiger",
"thumbnail": "//solutions.brightcove.com/bcls/assets/images/Tiger_poster.png",
"poster": "//solutions.brightcove.com/bcls/assets/images/Tiger_poster.png"
}, {
"sources": [{
"src": "//solutions.brightcove.com/bcls/assets/videos/Sea_ClownFish.mp4", "type": "video/mp4"
}],
"name": "Clownfish",
"thumbnail": "//solutions.brightcove.com/bcls/assets/images/Sea_ClownFish_poster.png",
"poster": "//solutions.brightcove.com/bcls/assets/images/Sea_ClownFish_poster.png"
}, {
"sources": [{
"src": "//solutions.brightcove.com/bcls/assets/videos/Sea_LionFish.mp4", "type": "video/mp4"
}],
Returned value (getter):
The method returns an array of objects.
An example of a returned Video Cloud playlist (an array of Video Cloud video objects) follows:
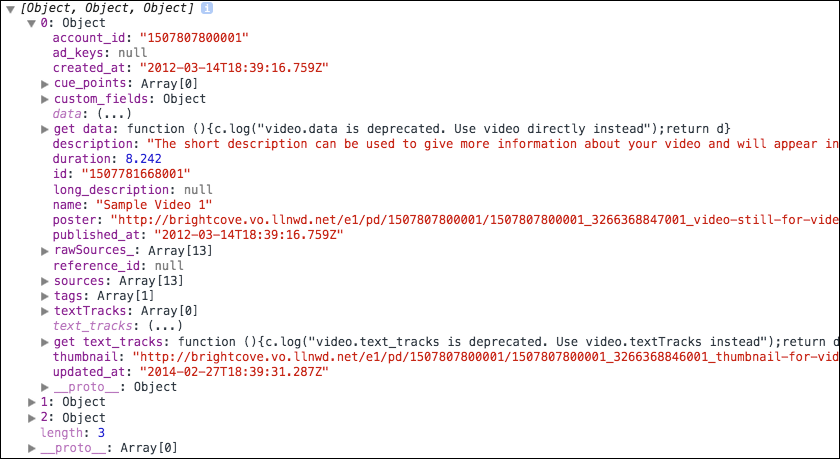
The following example shows using the catalog object's getPlaylist()
and load()
methods to load a Video Cloud playlist. As mentioned previously, this is the recommended approach when using Video Cloud playlists. For more information see the Player Catalog document.
myPlayer = this;
myPlayer.catalog.getPlaylist('1754200320001', function(error, playlist){
myPlayer.catalog.load(playlist);
console.log('mediainfo', myPlayer.mediainfo);
)};
You can use the object returned by the options()
method to retrieve the playlist ID. Here is a console.log
showing the syntax:
console.log('playlist id: ', myPlayer.options()['data-playlist-id']);
previous( ) method
The previous()
method plays the previous item in the playlist. The new video playing in the player will be returned from this method call. The currentItem
will be updated with the new index. If you are at the start of the playlist, you will not be able to proceed past the start and nothing will be returned.
Parameters:
- None
Returned value:
The method returns an object of type object. An example of this object for a manually created playlist follows:

An example of this object for a Video Cloud playlist follows:
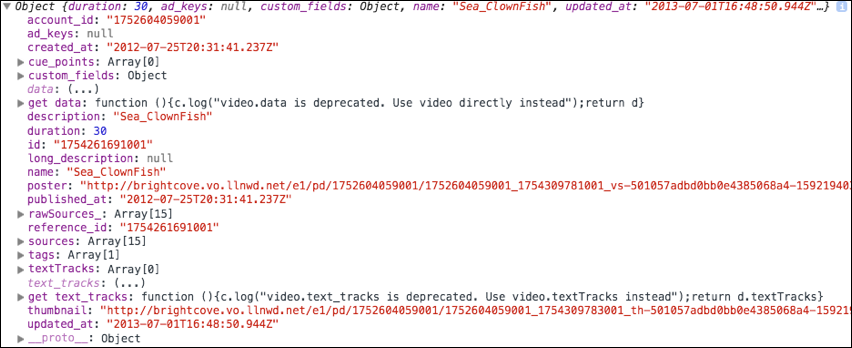
An example use of the method in a function to move to the previous video follows:
function previousVideo() {
myPlayer.playlist.previous();
}
previousIndex( ) method
The player.playlist.previsousIndex( )
method returns the index of the video proceeding the video currently playing in the player.
Parameter:
- None
Returned value:
The method returns a value of type number. The returned value represents the array position of the video proceeding the video currently playing in the player.
If the player is on the first item, the method returns 0. However, if the playlist repeats and is on the first item, returns the last item's index. If the player is currently playing a non-playlist video, it will return -1.
repeat() method
You can repeat a playlist after it has finished the last video in the playlist using the playlist.repeat()
method. This functionality plays the first video in the playlist once the last video in the playlist is finished.
Parameters:
- Boolean value indicating if the playlist should repeat or not; default is
false
Returned value:
The current value of playlist.repeat
The method sets the value by passing either true
or false
as the argument. If no argument is used, the method returns the current value. The following code shows the implementation:
- Lines 1-11: Standard playlist player code except an
id
is added with a value ofmyPlayerID
. - Line 16: Sets the playlist to repeat.
- Line 17: Shows the method in action as a getter. Displays in the console the value of
repeat
.
<video-js id="myPlayerID"
data-playlist-id="5455901760001"
data-account="1507807800001"
data-player="SyMOsyA-W"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/SyMOsyA-W_default/index.min.js"></script>
<div class="vjs-playlist"></div>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.playlist.repeat(true);
console.log('myPlayer.repeat()',myPlayer.playlist.repeat());
});
</script>
reverse( ) method
The player.playlist.reverse( )
method changes the order of the videos in the playlist so the first video becomes the last, and the last video becomes the first.
Parameter:
- None
Returned value:
The method does not return a value.
Reverses the order of the videos in the playlist. For example, the first video becomes the last, and the last video becomes the first.
The method dispatches the playlistsorted
event after reversing.
shuffle( ) method
The player.playlist.shuffle( )
method shuffles/randomizes the order of playlist items.
Parameter:
- Optional
rest
option: By default, the entire playlist is randomized. However, this may not be desirable in all cases, such as when a user is already watching a video. Whentrue
is passed for this option, it will only shuffle playlist items after the current item. For example, when on the first item, the method will shuffle only the second item and beyond. The syntax to use the parameter is:player.playlist.shuffle({rest: true});
Returned value:
The method does not return a value.
This method shuffles the items in the playlist using the Fisher-Yates shuffle algorithm.
The method dispatches the playlistsorted
event after shuffling.
If you wish to shuffle a playlist when the player is first loading, you must use this method in an event handler for the playlistsorted
event. This prevents the condition where the method tries to shuffle the playlist before it is loaded into the player. Sample syntax is:
myPlayer.on("duringplaylistchange", function() {
myPlayer.playlist.shuffle();
});
sort( ) method
The player.playlist.sort( )
method sorts the videos in a playlist in a manner identical to JavaScript's Array.prototype.sort() method.
Parameter:
- compareFunction: A function that defines how the videos should be sorted.
Returned value:
The method does not return a value.
An example use of the method to sort the videos by duration
follows:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.on('loadedmetadata', function(){
myPlayer.playlist.sort(function(a, b) {
return a.duration - b.duration;
});
});
});
The method dispatches the playlistsorted
event after reversing.
beforeplaylistitem event
The beforeplaylistitem
event is dispatched before switching to a new content source within a playlist (i.e., when any of currentItem()
, first()
, etc. is called) but before the player's state has been changed.
duringplaylistchange event
The duringplaylistchange
event is dispatched after the contents of the playlist are changed when calling playlist()
, but before the current playlist item is changed. The event object has several special properties:
nextIndex
: The index from the next playlist that will be played first.nextPlaylist
: A shallow clone of the next playlist.previousIndex
: The index from the previous playlist (will always match the current index when this event triggers, but is provided for completeness).previousPlaylist
: A shallow clone of the previous playlist.
Caveats
During the firing of this event, the playlist is considered to be in a changing state, which has the following effects:
- Calling the main playlist method,
player.playlist([...])
will throw an error. - Playlist navigation methods,
first
,last
,next
andprevious
, are rendered inoperable. - The
currentItem()
method only acts as a getter. - While the sorting methods,
sort
,reverse
andshuffle
, will continue to work, they do not dispatch theplaylistsorted
event.
Why use this event
This event provides an opportunity to intercept the playlist setting process before a new source is set on the player and before the playlistchange
event fires, while providing a consistent playlist API. One use-case might be shuffling a playlist that has just come from a server, but before its initial source is loaded into the player or the playlist UI is updated:
player.on('duringplaylistchange', function() {
// Remember, this will not trigger a "playlistsorted" event!
player.playlist.shuffle();
});
player.on('playlistchange', function() {
videojs.log('The playlist was shuffled, so the UI can be updated.');
});
playlistchange event
The playlistchange
event is dispatched asynchronously whenever the playlist is changed. When this event is dispatched the player will start loading the first video in the new playlist. It is fired asynchronously to let the browser start loading the first video in the new playlist.
playlistitem event
The playlistitem
event is dispatched when switching to a new content source within a playlist (i.e., when any of currentItem()
, first()
, etc. is called) after the player's state has been changed, but before playback has been resumed.
playlistsorted event
The playlistsorted
event is dispatched when any method is called that changes the order of playlist items, those being sort()
, reverse()
or shuffle()
.
playOnSelect
This value is a configuration option that when set to true
causes a clicked video from the playlist to start playing, even if the current video in the player was previously paused. By default, clicking a new video from the playlist will load the new video but keep the player paused if it was paused beforehand.
Information on to manipulate this value can be found in the Player Configuration Guide - Playlists section.
Visual playlists
If you wish to have a visual representation of the playlist in your page you must configure the player to do so. You can update the configuration using Studio, in the PLAYERS > Styling section, as shown here (you can choose either a vertical or horizontal playlist):
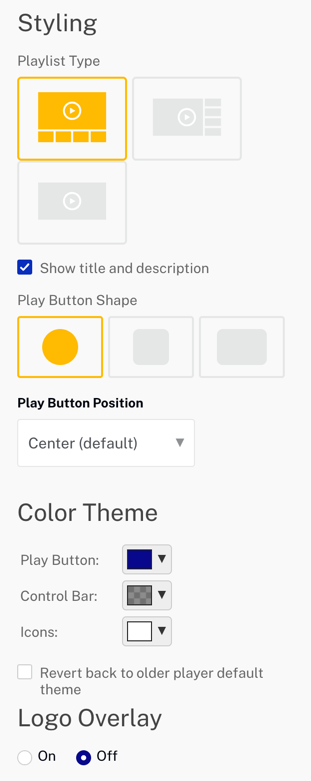
If you wish, you can also so use the Player Management API to configure you player for playlists. See the Playlists section of the Player Configuration Guide for complete information.
You can programmatically check to see if playlists are enabled by checking if the value of player.playlistUi
is defined.
console.log('myPlayer.playlistUi:', myPlayer.playlistUi);
Catalog playlists
As mentioned earlier in the document the playlist can be a Video Cloud playlist. You can retrieve a Video Cloud playlist using the player.catalog.getPlaylist()
method. You then load the playlist into the player using the player.catalog.load()
method. The following code shows this usage:
myPlayer = this;
myPlayer.catalog.getPlaylist('1754200320001', function(error, playlist){
myPlayer.catalog.load(playlist);
console.log('mediainfo', myPlayer.mediainfo);
});