In this topic, you will learn how Brightcove Player implements digital rights management (DRM). The document first shows how to implement the DRM plugin, then is followed by details of the plugin and how it is implemented.
Introduction
Brightcove is embracing the following technologies to deliver DRM protected content to the widest possible variety of browsers and devices:
- MPEG-DASH with Native/EME supported CENC DRMs
- HLS with FairPlay
To use DRM with Brightcove Player you must:
- Produce DRM enabled content
- Enable Brightcove Player to use the DRM plugin
- In some cases, configure the DRM plugin to use your license server
Terminology
Implement using Players module
To implement the DRM Plugin in Studio, and enable DRM based on your account setup, follow these steps:
- Open the PLAYERS module and either create a new player or locate the player to which you wish to add DRM functionality.
- Click the link for the player to open the player's properties.
- Click Playback in the left navigation menu.
- Next, check the Enable DRM checkbox.
- To publish the player, click Publish & Embed > Publish Changes.
- To close the open dialog, click Close.
DRM plugin architecture
The DRM plugin (videojs-drm) is a wrapper around two plugins:
- videojs-silverlight
- videojs-contrib-eme
The videojs-drm plugin version 5 uses the player's built-in DASH playback capabilities. This utilizes VHS, which is the next and renamed version of the built-in videojs-contrib-hls plugin.
As of player 6.26.0 the player supports DASH multi-period. For earlier versions of the player, the Shaka player is required for DASH multi-period.
If you want to use the Shaka Player for DASH playback, like it did in version 4, you can include the following script along with videojs-drm version 5 script:
https://players.brightcove.net/videojs-shaka/1/videojs-shaka.js
The videojs-silverlight plugin allows for playback of DASH content on certain Internet Explorer browsers.
The videojs-contrib-eme plugin allows for playback of FairPlay HLS content.
Playback technologies used
Brightcove Player utilizes different DRM playback technologies with different browsers. The following details the DRM technologies used with Brightcove Player:
- FairPlay: Apple's DRM system
- PlayReady: Microsoft's DRM system
- Widevine: Google's DRM system
The following table details the relationship between the browser (latest version), format and playback technology used in Brightcove Player:
Browser | Format | DRM Playback Technology | Rendition Type Used to Deliver DRM Content |
---|---|---|---|
Chrome Desktop | HLS or DASH with Widevine | EME | HLS or MPEG-DASH |
Chrome Mobile1 | HLS or DASH with Widevine | EME | HLS or MPEG-DASH |
Internet Explorer2 | HLS or DASH with PlayReady | EME | HLS or MPEG-DASH |
Edge | HLS or DASH with Widevine | EME | HLS or MPEG-DASH |
Safari | HLS with FairPlay | Native | HLS |
Firefox | HLS or DASH with Widevine | EME | HLS or MPEG-DASH |
1DRM playback using Chrome Mobile on iOS is not supported.
Produce DRM content
There are two steps you must perform to create DRM enabled content:
- Contact your account manager to have your account(s) DRM-enabled. You can then configure your account with the proper licensing keys and ingest profiles to enable the creation of DRM protected content.
-
Produce DRM protected content. You can choose to either upload new content or re-encode existing content as DRM. This is done by selecting the Ingest Profile that produces your desired encryption technology.
You will need to produce DRM protected content which uses either MPEG-DASH manifests with segmented and encrypted videos, or HLS FairPlay content.
Implementing FairPlay playback in code
Implementing Widevine playback in code
Supporting other DRM providers
The plugin has implemented a path that allows customers to implement support for other DRM providers. This is mostly useful for Fairplay, as Fairplay requires custom logic necessary to get license information. Adding a vendor.name
to keySystems
on a given source will attempt to utilize this logic, and include the necessary certificate and license information. An example for Azure follows:
player.ready(function(){
player.eme();
player.src({
src: 'http://example.com/src-url.m3u8'
type: '',
keySystems: {
'com.apple.fps.1_0': {
vendor: {
name: 'azure'
},
certificateUri: 'https://example.com/your-certificate-uri.cer',
licenseUri: 'https://example.com/your-license-uri'
}
}
});
});
Here is an example for castLabs:
var player = videojs.getPlayer('myPlayerID');
player.ready(function(){
player.eme();
player.src({
src: 'http://example.com/src-url.m3u8'
type: '',
keySystems: {
'com.apple.fps.1_0': {
vendor: {
name: 'castlabs',
options: {
authToken: 'your-auth-token',
customData: 'your-custom-data'
}
}
certificateUri: 'https://example.com/your-certificate-uri.cer',
licenseUri: 'https://example.com/your-license-uri'
}
}
});
});
This example loads Widevine and PlayReady sources:
player.src({
type: 'application/dash+xml',
src: '<some src>',
keySystems: {
'com.widevine.alpha': '<license url>',
'com.microsoft.playready': '<license url>'
}
});
Enable debugging
To enable debugging for your DRM DASH content, add the following shaka scripts to your Brightcove Player:
<!-- Script for the drm plugin -->
<script src="https://players.brightcove.net/videojs-drm/5/videojs-drm.min.js"></script>
<!-- Script for the shaka plugin -->
<script src="https://players.brightcove.net/videojs-shaka/1/videojs-shaka.js"></script>
<!-- Script for shaka debug plugin -->
<script src="https://players.brightcove.net/videojs-shaka/1/videojs-shaka.debug.js"></script>
The following is an example of the debugging at player load:
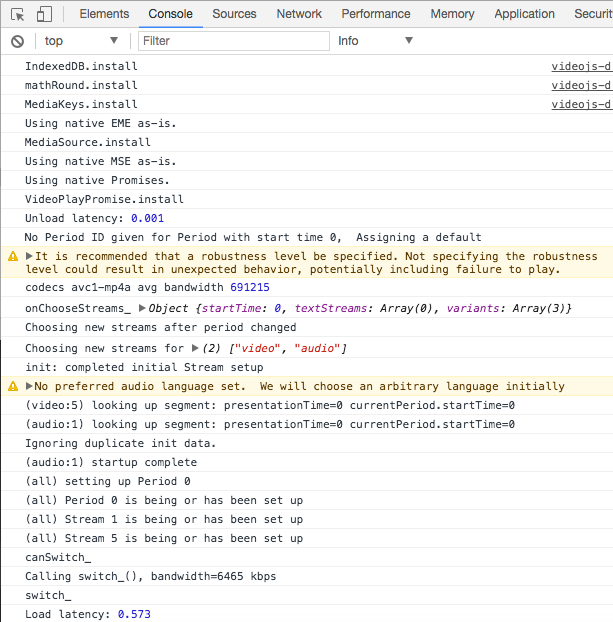
The following is an example of the debugging after the video has begun to play:
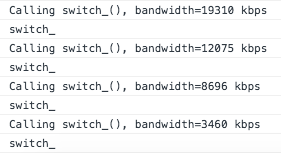
DASH-IF
The Brightcove Player supports DASH Industry Forum (DASH-IF) assets, passing through Brightcove data to Native/EME. See http://dashif.org for more information on DASH-IF. The following DASH-IF links are also helpful when using DASH:
Playback restrictions
To configure Brightcove Player to use Playback Restrictions, see here.
Known issues
- The Default (Auto Display) caption setting in the Media module's TEXT TRACK section is not supported when used in conjunction with DRM and in-manifest captions. In-manifest captions are used with the Brightcove products Dynamic Delivery and SSAI, for example. A workaround for this issue is to use the
<track>
tag with the Advanced Brightcove Player implementation. This is detailed in the Adding Captions to Videos Programmatically document. Note you must use thedefault
attribute with the<track>
tag. - DRM assets and Chrome: When using the Standard (iframe) player implementation with the DRM plugin,
allow="encrypted-media"
is required to be able to play DRM assets in Chrome.<iframe src="https://players.brightcove.net/123456789/BydO6uuuu_default/index.html?videoId=5783262319001" allowfullscreen width="640" height="360" allow="encrypted-media"></iframe>
- Special event for Silverlight/IE11: In most cases if you wish to programmatically interact with the player you would wait for either the
ready
orloadedmetadata
event to be dispatched. However, if you wish to programmatically interact with the player when using the Silverlight tech in IE11, AND playing DRM content, you should wait for thecanplay
event. - On iOS, only Safari provides the necessary DRM browser APIs (EME), WebViews do not currently have EME support. Therefore Fairplay DRM will only work on Safari.
Changelog
See the DRM Plugin Release Notes.
For historical release notes, see the changelog here.