Retrieving the bitrate
You can use the following code to retrieve the bitrate of the currently playing rendition:
player.tech(true).vhs.playlists.media().attributes.BANDWIDTH
The following shows how to use the code with an Advanced player implementation:
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="default"
data-embed="default"
controls=""
data-video-id="6201753345001"
data-playlist-id=""
data-application-id=""
width="960" height="540"></video-js>
<script src="https://players.brightcove.net/1507807800001/default_default/index.min.js"></script>
<script>
videojs.getPlayer('myPlayerID').ready(function () {
var myPlayer = this;
myPlayer.on('play', function () {
console.log('Bitrate of playing rendition :', myPlayer.tech(true).vhs.playlists.media().attributes.BANDWIDTH);
})
});
</script>
The result, in this instance, would appear in the console as follows:
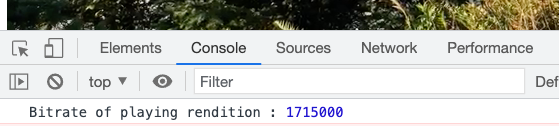
Using the console
You can retrieve the same information in the console. Given the player is assigned an ID, in this case myPlayerID
, the following can be used to get the bitrate of the currently playing rendition:

Usage details
Please note the following details when using the code:
- The code will return the bitrate based on what is defined in the HLS or DASH manifests for a demuxed video playlist or a muxed video/audio playlist.
- The code will NOT work with the following:
- MP4 encoded videos
- When native playback is used, like HLS on Safari
- When only a media playlist is sent without a manifest. This will NOT work:
while this willhttps://d2zihajmogu5jn.cloudfront.net/bipbop-advanced/gear2/prog_index.m3u8
https://d2zihajmogu5jn.cloudfront.net/bipbop-advanced/bipbop_16x9_variant.m3u8
- The returned value could be a bit ahead of where playback is currently happening as Brightcove Player is always buffering content ahead of current playback location.
- If you wish to see information on the currently playing video's rendition playlist, you can use one of the following:
- The Segment Metadata text track to get information about the currently playing demuxed video playlist or muxed video/audio playlist.
- The mediainfo property where you can view the
sources
array, an example of which is shown here:
- It's not possible to get the bitrate information of the audio content, whether it's muxed or demuxed.
What playlist is used?
In the code a playlist is referenced, but the player implementation was only playing a single video:
myPlayer.tech(true).vhs.playlists.media().attributes.BANDWIDTH
This is NOT referring to a playlist of videos. Rather it is referencing an HLS manifest of renditions, which is also referred to as a playlist. For instance, in this video there are both HLS and MP4 renditions (the HLS renditions, or HLS playlist, are highlighted):
