Using the usingAdBlocker() method
Brightcove Player has as method that checks to see if an ad blocker is being used by the browser playing your video. This method is usingAdBlocker()
. Once the determination is made if an ad blocker is detected, your business rules will guide you on further actions. In the following example three ads will be displayed during the video, or if an ad blocker is detected a message is displayed below the player.
In case you don't have an ad blocker installed for testing, for this example the message boxed in red is displayed when an ad blocker is detected:
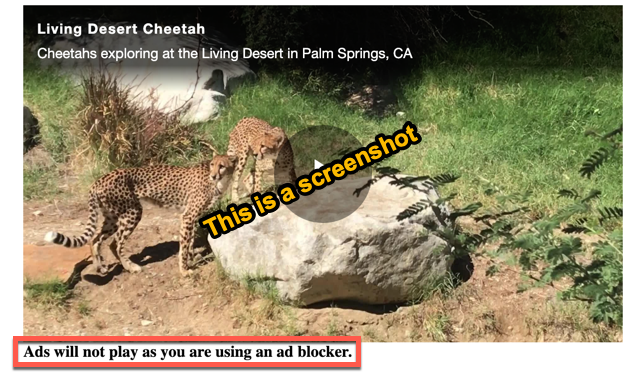
Implementation
The following code is a full example of using the usingAdBlocker()
method. Basically the code does the following:
- Uses a promise to check and see if an ad blocker is detected.
- If yes, a paragraph is dynamically created and inserted into a pre-existing
div
.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>Untitled Document</title>
</head>
<body>
<div style="width: 600px;">
<video-js id="myPlayerID"
data-account="1752604059001"
data-player="ZDaDSLULho"
data-embed="default"
controls=""
data-video-id="5701193190001"
data-playlist-id=""
data-application-id=""
width="600" height="337.5" class="vjs-fluid"></video-js>
</div>
<script src="https://players.brightcove.net/1752604059001/ZDaDSLULho_default/index.min.js"></script>
<div id="ad-blocker-note"></div>
<script>
videojs.getPlayer('myPlayerID').ready(function () {
var myPlayer = this;
myPlayer.usingAdBlocker().then( hasBlocker => {
if (hasBlocker) {
var newP = document.createElement("p");
newP = '<strong>Ads will not play as you are using an ad blocker.</strong>';
document.getElementById("ad-blocker-note").insertAdjacentHTML('afterbegin', newP);
}
});
</script>
</body>
</html>
Of course, you can use an if
statement for the JavaScript logic.
videojs.getPlayer('myPlayerID').ready(function () {
var myPlayer = this;
if (myPlayer.usingAdBlocker()) {
var newP = document.createElement("p");
newP = 'This ad will not play as you are using an ad blocker.';
document.getElementById("ad-blocker-note2").insertAdjacentHTML('afterbegin', newP);
}
});