Programmatically Localizing Brightcove Player
Document structure
First the process for localizing the In-Page Embed implementation is shown, then the iframe implementation. The document concludes by demonstrating how to edit or add to localized vocabulary if you wish to localize content on the page surrounding the player.
Include language(s)
The first task for localization is to include the language(s) you wish to use for localization. You can see the languages available at the Video.js language page, shown here.
- ar.json - Arabic
- de.json - German
- en.json - English
- es.json - Spanish
- fr.json - French
- ja.json - Japanese
- ko.json - Korean
- zh-Hans.json - Mandarin Chinese, Simplified script
- zh-Hant.json - Mandarin Chinese, as written in Traditional script
The content of the German (de) file is shown below. The file shows the words/phrases that will be translated automatically.
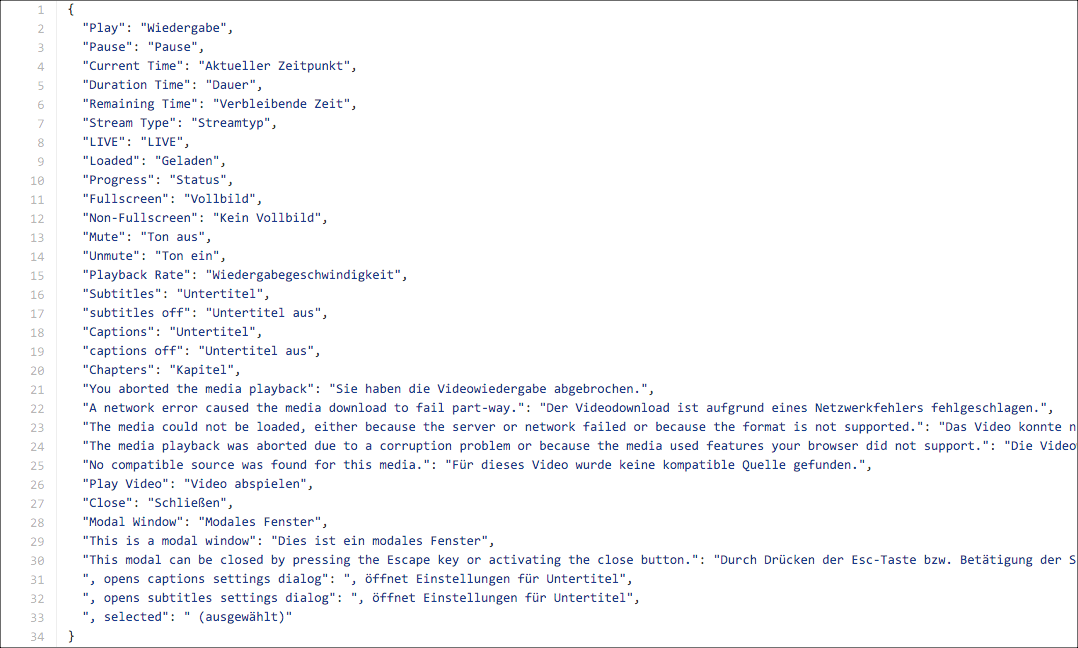
Once you have decided on what languages to support for localization, you must configure your player to include them. You do this using the Player Management API. You could use code patterned on the following curl statement to add language support when creating the player:
curl \
--header "Content-Type: application/json" \
--user $EMAIL \
--request POST \
--data '{
"name": "Localization Example",
"configuration": {
"languages": [
"de",
"es"
],
"media": {
"sources": [{
"src":"http://solutions.brightcove.com/bcls/assets/videos/Tiger.mp4",
"type":"video/mp4"
}]
}
}
}' \
https://players.api.brightcove.com/v2/accounts/$ACCOUNT_ID/players
If the player already exists, and you wish to add language support, you could use code patterned on the following curl statement to update the player:
curl \
--header "Content-Type: application/json" \
--user $EMAIL \
--request PATCH \
--data '{
"languages": [
"de",
"es"
]
}' \
https://players.api.brightcove.com/v2/accounts/$ACCOUNT_ID/players/$PLAYER_ID/configuration
Now you'll publish the player to make the changes to the published player. Enter the following command:
curl \
--header "Content-Type: application/json" \
--user $EMAIL \
--request POST \
https://players.api.brightcove.com/v2/accounts/$ACCOUNT_ID/players/$PLAYER_ID/publish
An example player configuration with languages set appears as follows:
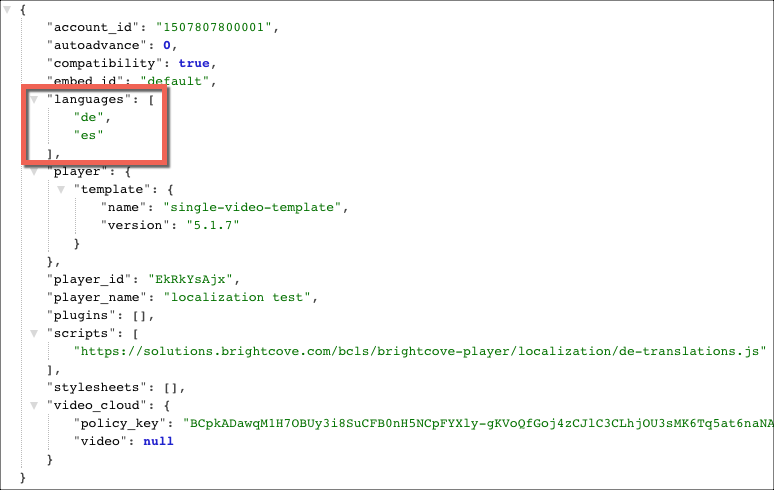
Set language
To use the localized control names, the user can set the preferred language in the browser. The exact steps vary depending on browser and OS (broad guidance is given in the next paragraph). If the browser's preferred language is English, or a language not available, you will see the default, or English, text.
Browsers do not have perfectly standardized locale detection, so it can be a bit complicated. Broadly, browsers look to determine the desired language in this order:
- Check if the embedding page has a
lang
attribute on the<html>
element. - Check browser specific locale configurations.
- Fall back to English.
iframe query parameter
If you are using the iframe implementation of the player you can set the language for that player by including a query parameter in the src
attribute. Added to the existing query parameter that has the video ID, for instance ?videoId=4607746980001
, you can set the language using &language=
followed by the language code. For example, here is an iframe player implementation setting the language to German:
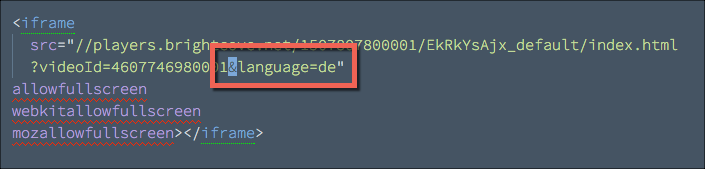
Results
As mentioned in the opening paragraph of this document, the results of a localized player are not easily seen. To check for successful localization you can inspect the big-play-button. Use a browser's debugging tools to inspect the button.
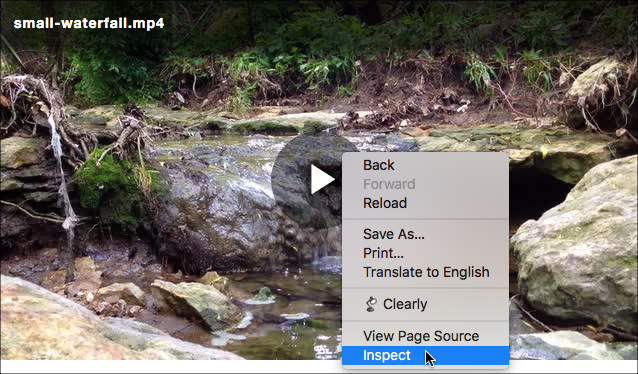
Drill down into the HTML until you see the text for the button, which in the non-localized version is Play Video.
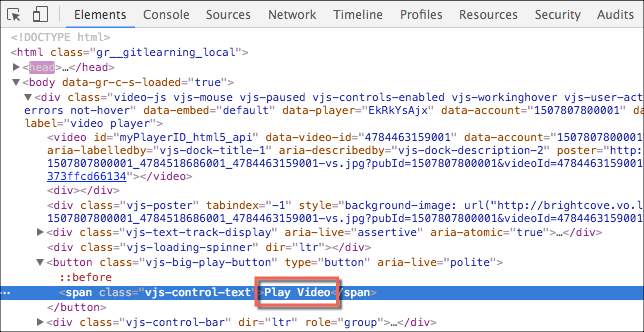
After following the steps shown above, the player will be localized. In the following screenshot the button is localized for German.
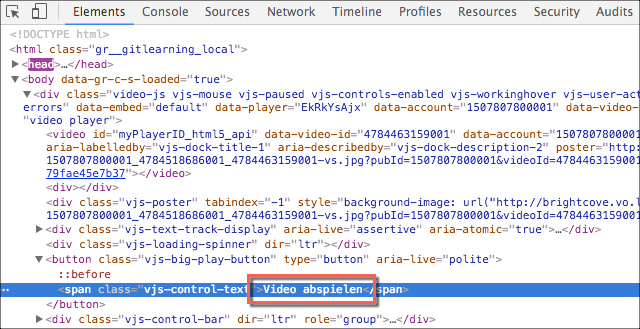
iframe implementation
Localizing the iframe implementation of Brightcove Player is the same as localizing the In-Page Embed version. All you need to do is include the wanted languages and set the preferred language in the browser. Both of these procedures are detailed earlier in this document. There is no code to modify.
Alter/Add to language
If you wish to change, or add to, the given localizations found at the Video.js language page it is quite easy to do. You can copy the given translations and then modify as you wish. For instance, below are the German translations with Hello and Goodbye added to the list. (For brevity's sake, not all the control elements translations are included.)
videojs.addLanguage("de", {
"Play": "Wiedergabe",
"Pause": "Pause",
"Current Time": "Aktueller Zeitpunkt",
"Hello": "Guten Tag",
"Goodbye": "Auf Wiedersehen"
});
This JavaScript could be in the HTML page itself, included as a source in a <script>
tag, or add the script to the player's configuration using Studio. The URL to the file is added in the Plugins section of the player's properties. Use the Add a File button to add the JavaScript file, as shown here:
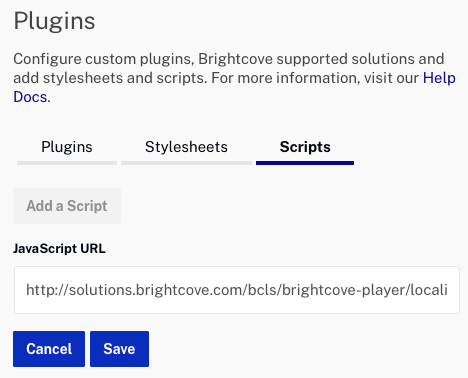
Once the added vocabulary is in the language file, you can use the player's localize()
method to access it. The following code snippet shows the localized word for Hello being used in the HTML page, followed by a screenshot of the results.
<p id="textTarget">In here: </p>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
document.getElementById("textTarget").innerHTML += myPlayer.localize("Hello");
});
</script>
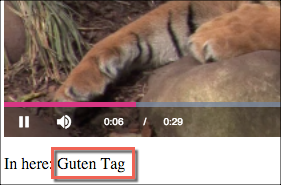