Video Metadata from mediainfo
mediainfo
object. The property is populated after the loadstart
event is dispatched.mediainfo property
The mediainfo
property is an object which contains information (metadata) on the current video in the player.
The mediainfo
property is an object which contains information (metadata) on the current video in the player. You populate the object with video information from your CMS (content management system) or database.
After the mediainfo
object is populated you can use it for convenient data retrieval when wishing to display video information, like the video name or description. The object is also used in analytics collection.
Fields in mediainfo
The fields present in the mediainfo
property are as follows:
Field Name | Description | Data Type |
---|---|---|
accountId |
Brightcove account | string |
adKeys |
For future support | N/A |
createdAt |
Creation date and time | UTC (2011-09-28T20:06:37.879Z) |
cuePoints |
List of cue points as an array of objects | array of objects |
customFields |
Key-value pairs of custom field names and associated values | Object |
description |
Short description, 250 characters maximum | string |
duration |
Length of video in seconds | numeric |
economics |
Contains either AD_SUPPORTED or FREE. If the media is marked FREE, neither IMA3 nor FreeWheel ads will play. | string |
id |
Video IDUnique identifier associated with the video | string |
link |
Object that contains link text and url properties | object |
longDescription |
Description, 5000 characters maximum | string |
name |
Video title | string |
poster |
URL to the poster image | string |
posterSources |
Array containing poster sources | array |
publishedAt |
Publication date and time | UTC (2011-09-28T20:06:37.879Z) |
rawSources |
Rendition information | array |
referenceId |
Video reference IDKey or identifier from external database or CMS | string |
sources |
List of renditions as an array of objects; each object includes at least two elements: type and src | array of objects |
tags |
Tags (metadata) associated with the video | array of strings |
textTracks |
Array that holds text tracks for captions, cue points, etc. | array |
thumbnail |
URL to the thumbnail image | string |
thumbnailSources |
Data structure containing poster thumbnails | string |
variants |
An array of objects containing multilingual metadata for the videos; the object properties: language , name , description , long_description , and custom_fields |
Array |
Populate mediainfo
Using the on()
method you wait for the loadstart
event to be dispatched, then in the corresponding event handler you access the values stored in mediainfo
object. From the code below note the use of console.log
that displays the mediainfo
object (lines 111-113).
<video-js id="myPlayerID"
data-video-id="4093643993001"
data-account="1752604059001"
data-player="VJvZIueYx"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1752604059001/VJvZIueYx_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.on('loadstart',function(){
console.log('mediainfo', myPlayer.mediainfo);
})
});
</script>
An example display from the console is shown here:
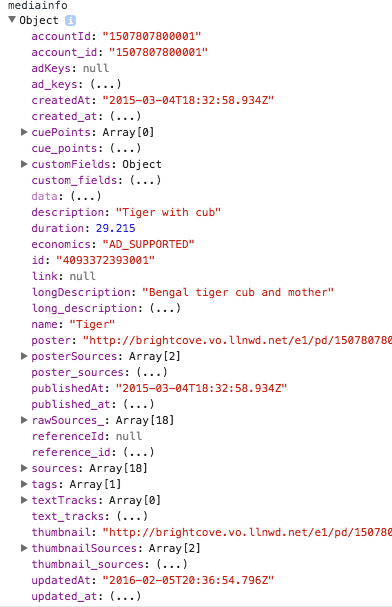
Populate the object
You can manually populate the mediainfo
property with metadata from your CMS or database. You can then use the mediainfo
object's sources
property to populate the player with a video rendition.
- Lines 32-37: In-page embed player implementation
- Lines 40-52: Manually creates the a video object; in actuality data comes from CMS or database and object built dynamically
- Line 54-55: Ensures the player is ready to use and assigns the player instance to a variable
- Line 56: Assigns the manually created video object to the player's
mediainfo
object - Line 57: Uses the player's
src
method to assign one or more video renditions, which are stored in themediainfo
object'ssources
property, to the player
<video-js id="myPlayerID"
data-account="3676484086001"
data-player="2433352b-a2e2-4b7e-9a15-2d9ec7f07e9d"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/3676484086001/2433352b-a2e2-4b7e-9a15-2d9ec7f07e9d_default/index.min.js"></script>
<script type="text/javascript">
//Populate video object (normally from database or CMS)
var videoObject = new Object();
videoObject.name = "Hand made video object test";
videoObject.id = "1234msb";
videoObject.description = "short description for hand made video object";
videoObject.accountId = "1507807800001";
videoObject.sources = [{
"src": "http://solutions.brightcove.com/bcls/assets/videos/Tiger.m3u8",
"type": "application/x-mpegURL"
}, {
"src": "http://solutions.brightcove.com/bcls/assets/videos/Tiger.mp4",
"type": "video/mp4"
}];
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.mediainfo = videoObject;
myPlayer.src(myPlayer.mediainfo.sources);
});
</script>
Display tags and custom fields
Both the tags and custom fields are stored in complex data structures. The customFields in an object and tags in an array. The following screenshot shows the custom fields and tags highlighted in the mediainfo
object.
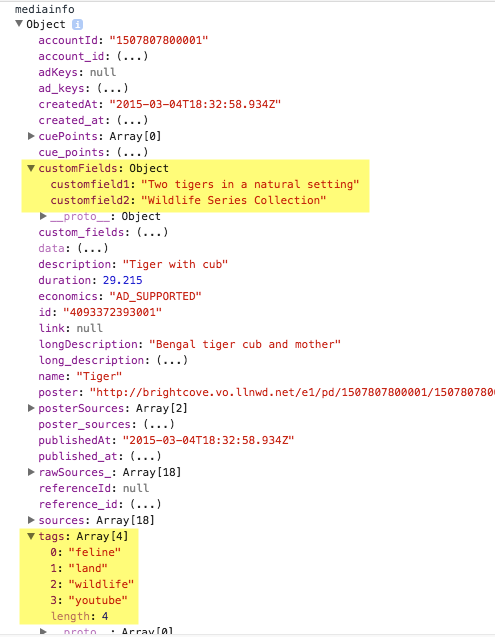
To display the data from these complex data structures you need two kinds of loops. A for
loop is used to iterate over the tags array (lines 16-20) and inject the dynamically built HTML unordered list into a <div>
( defined in line 9). A for-in
loop is used to iterate over the custom fields object (lines 24-27) and inject the dynamically built HTML unordered list into the <div>
.
<video-js id="myPlayerID"
data-video-id="4093643993001"
data-account="1752604059001"
data-player="VJvZIueYx"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1752604059001/VJvZIueYx_default/index.min.js"></script>
<div id="displayInfo"></div>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.on('loadstart',function(){
//Use JavaScript to display the tags
var numTags = myPlayer.mediainfo.tags.length;
displayInfo.innerHTML += "<h1>Tags:</h1><ul>";
for (var i = 0; i < numTags; i++) {
displayInfo.innerHTML += "<li>" + myPlayer.mediainfo.tags[i] + "</li>";
};
displayInfo.innerHTML += "</ul><br />";
//Use JavaScript to display custom fields
displayInfo.innerHTML += "<h1>Custom Fields:</h1><ul>";
for (var key in myPlayer.mediainfo.customFields) {
displayInfo.innerHTML += "<li><strong>" + key + "</strong>: " + myPlayer.mediainfo.customFields[key] + "</li>";
}
displayInfo.innerHTML += "</ul>";
})
});
</script>
The rendered HTML appears as shown in this screenshot.
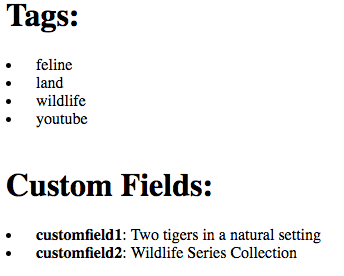