Ad Events and Ad Objects
Overview
The videojs-contrib-ads, located in the videojs/videojs-contrib-ads GitHub repository, provides common functionality needed by video advertisement libraries and video ad integrations, reducing the code you have to write for your specific ad integration.
These events and objects are implemented in the Brightcove supplied FreeWheel and IMA3 plugins. These same events and objects are implemented in the SSAI plugins, except for the following:
player.ads.pod
interfaceplayer.ads.provider
object
Ad events
Each ad provider can emit a unique set of events. Typically, these events are used to track the state of ad playback for metrics purposes and to create custom UI's. It is suggested to implement the following events to encourage uniformity, and allow custom UIs and analytics providers to remain ad provider agnostic.
Event | Dispatched when: |
---|---|
ads-request |
Ad data is requested |
ads-load |
Ad data is available following an ad request |
ads-pod-started |
A linear ad pod has started |
ads-pod-ended |
A linear ad pod has completed |
ads-allpods-completed |
All linear ads are completed |
ads-ad-started |
Ad starts playing |
ads-ad-ended |
Ad completes playing |
ads-first-quartile |
Ad playhead crosses the first quartile |
ads-midpoint |
Ad playhead crosses the midpoint |
ads-third-quartile |
Ad playhead crosses the third quartile |
ads-pause |
Ad is paused |
ads-play |
Ad is resumed |
ads-volumechange |
Ad volume has been changed |
ads-click |
Ad is clicked |
These events can be listened for from the player object. Below is the code to listen for the ads-ad-started
object, as well as a screenshot of what the object passed to the event handler looks like.
videojs.getPlayer('myPlayerID').on('loadedmetadata',function(){
var myPlayer = this;
...
myPlayer.on('ads-ad-started',function( evt ){
console.log('ads-ad-started event passed to event handler', evt);
});
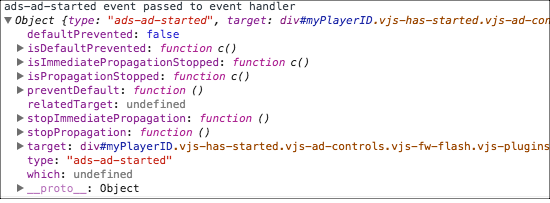
Ad objects
As you see, the event object passed to the event handler is not terribly informative. For this reason, there are two objects you can leverage from which you can gather more ad information, they are player.ads.ad and player.ads.pod.
From the screenshot below, you see the player.ads.ad object contains information on the ad type
and duration
of ad, among other details.

The player.ads.pod object contains similar information to player.ads.ad, with the addition of the number of ads in the pod. The first screenshot below shows an ad pod with a single ad, whereas the second shows a pod with three ads.


Google's AdsManager
There are methods and properties available from Google's google.ima.AdsManager Interface. You can use the interface's properties/methods that retrieve information. It is not advised to use the methods that perform actions, like destroy
, setAutoPlayAdBreaks
and stop
. For example, one method you can use is shown here:
AdsManager.getRemainingTime
Type: google.ima.AdsManager.getRemainingTime
Usage: myPlayer.ima3.adsManager.getRemainingTime()
Calling this method returns the amount of time remaining for the current ad. If an ad isn't available or has finished playing, it returns -1. For more information see Google's document on the method.