Player Catalog
Basic syntax
The basic syntax for using the catalog
is as follows:
- Methods:
playerName.catalog.methodName()
Several of the catalog
methods will pass an object of parameters that define the request. The catalog parameters object is defined in the next section, and will be referenced in the methods' descriptions, where applicable.
catalog parameters object
The following table describes the properties in the CatalogParams
object. This true object (not a constructor) defines the format for parameters to be used with many catalog requests.
Name | Type | Description |
---|---|---|
type |
string |
The type of request to make. Must be one of video, playlist, or search. |
policyKey |
string |
The policy key for this account. This will default to the policy key of the player.** Note that if you pass a different account ID as a parameter, you must also pass the corresponding policy key for that account. |
deliveryConfigId |
string |
The delivery rules id. See Overview: Delivery Rules. |
[id] |
string |
A video or playlist ID or reference ID prefixed by "ref:". Required for video and playlist requests. DEPRECATED: For backward-compatibility, this is supported as a search query instead of |
[q] |
string |
A search query. Required for search requests, ignored for others. |
[adConfigId] |
string |
A Video Could SSAI ad configuration ID. |
[tveToken] |
string |
An optional TVE token to be sent as a query string parameter. |
[limit] |
string |
Supported for playlist and search types only. Limit the number of videos returned. |
[offset] |
string |
Supported for playlist and search types only. The number of videos to skip. |
[sort] |
string |
Supported for search type only. How the videos should be sorted for searches. |
[bcovAuthToken] |
string |
For use with Brightcove Playback Authorization Service (PAS). |
getVideo( )
method
The getVideo(params | videoID,callback,[adConfigId]) => XMLHttpRequest
method makes a catalog
request for the video with the specified id and invokes a callback when the request completes.
Parameters
-
params | videoID: An object of type catalog parameters OR a string containing the video ID or a reference ID prefixed with ref:.
-
callback: A function that will be called when the request completes, whether it's successful or not. It is invoked with two arguments:
-
The first argument will contain an error object, if an error occurs. This object contains details of the error. If no error occurs, this object is null.
-
If no error occurs, the second argument is the requested video object. If an error occurs, this value contains the empty string.
-
-
[adConfigId]: DEPRECATED A Video Cloud ad configuration ID that will be passed along to the Playback API. Instead, pass the adConfigId in the initial params object.
Returned value
The catalog
call itself returns an object of type XMLHttpRequest. An example of this object follows:
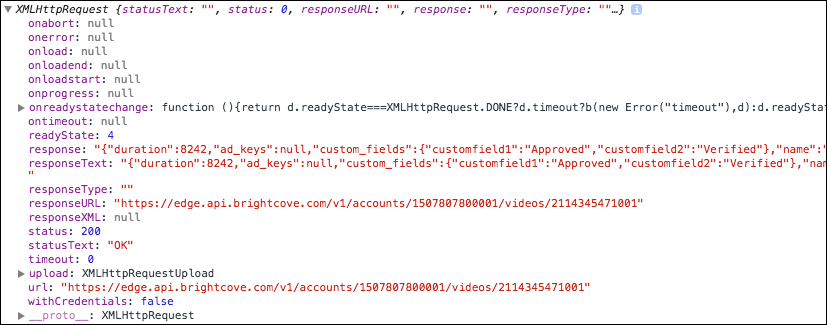
Although the call returns an XMLHttpRequest object, you will most likely never deal with that object. The reason is that in the callback function the JSON response is automatically parsed and a usable object is what is passed to the callback function, in this case a video object.
Example: Video ID as string
The following example shows a use of the getVideo()
method:
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="3bc25a34-086a-4060-8745-dd87af3d53b4"
data-embed="default"
data-video-id=""
controls=""></video-js>
<script src="https://brightcove.net/1507807800001/3bc25a34-086a-4060-8745-dd87af3d53b4_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.catalog.getVideo('2114345471001', function(error, video){
//deal with error
myPlayer.catalog.load(video);
});
});
</script>
Example: Using catalogParams
The equivalent of the code just above, but using the CatalogParams object appears as follows:
<script>
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
catalogParams = {};
catalogParams.type = 'video';
catalogParams.id = '2114345471001';
myPlayer.catalog.getVideo(catalogParams, function(error, video){
//deal with error
myPlayer.catalog.load(video);
});
});
</script>
getPlaylist( )
method
The getPlaylist(params | playlistID,callback,[adConfigId]) => XMLHttpRequest
method makes a catalog
request for the playlist with the specified id and invokes a callback when the request completes.
Parameters
-
params | playlistID: An object of type catalog parameters OR a string containing the playlist ID or a reference ID prefixed with ref:.
-
callback: A function that will be called when the request completes, whether it's successful or not. It is invoked with two arguments:
-
The first argument will contain an error object, if an error occurs. This object contains details of the error. If no error occurs, this object is null.
-
If no error occurs, the second argument is the requested video object. If an error occurs, this value contains the empty string.
-
-
[adConfigId]: DEPRECATED A Video Cloud ad configuration ID that will be passed along to the Playback API. Instead, pass the adConfigId in the initial params object.
Returned value
The catalog
call itself returns an XMLHttpRequest. Although the call returns an XMLHttpRequest object, you will most likely never deal with that object. The reason is that in the callback function the JSON response is automatically parsed and a usable object is what is passed to the callback function, in this case a playlist object.
Example
The following example shows a use of the getPlaylist()
method follows:
<video-js id="myPlayerID"
data-account="1752604059001"
data-player="f50a2d3c-af51-4d8c-84e3-0c7cdec0edbe"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1752604059001/f50a2d3c-af51-4d8c-84e3-0c7cdec0edbe_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.catalog.getPlaylist('1754200320001', function(error, playlist){
//deal with error
myPlayer.catalog.load(playlist);
})
});
</script>
load( ) method
The load(mediaobject)
method loads a video or playlist object into the player. This method will update the player's mediainfo
property, and update the video source and poster. In most cases, you would call this method with the result of a call to getVideo()
or getPlaylist()
. If you'd like to be notified when mediainfo
has been updated, listen for the loadstart
event.
Parameters
-
mediaobject: A video or playlist object to load. This object should have the same format as the response object from a call to
getVideo()
orgetPlaylist()
method.
Example
THe following example retrieves a video and loads it into the player.
<video-js id="myPlayerID"
data-account="1507807800001"
data-player="3bc25a34-086a-4060-8745-dd87af3d53b4"
data-embed="default"
data-video-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/3bc25a34-086a-4060-8745-dd87af3d53b4_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.catalog.getVideo('2114345471001', function(error, video) {
//deal with error
myPlayer.catalog.load(video);
});
});
</script>
get() method
The get(params, [callback]) ⇒ Promise | XMLHttpRequest
method permits searching the catalog
using CMS/Playback API: Videos Search syntax. Under the hood, this method uses the Playback API.
Parameters
-
params: An object of type catalog parameters.
-
callback: A function that will be called when the request completes, whether it's successful or not. It is invoked with two arguments:
-
The first argument will contain an error object, if an error occurs. This object contains details of the error. If no error occurs, this object is null.
-
If no error occurs, the second argument is the requested video object. If an error occurs, this value contains the empty string.
-
Returned value
The method returns a Promise or an object of type XMLHttpRequest. If you are using a callback function, the JSON response is automatically parsed and a usable object is what is passed to the callback function. The exact structure of the returned data in the callback will vary depending what was searched for.
Callback example
The following example does the following:
-
Builds a catalogParams object that will be passed as an argument with the function. The search will query for videos with the string Desert in the video's name.
-
Performs the search using the
get()
method. Note that the callback function will use the parameters namederrorObj
andvideosReturned
. -
Use the
playlist()
method to assign the returned videos to the Brightcove Player.
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
catalogParams = {};
catalogParams.type = 'search';
catalogParams.policyKey = 'BCpkADawqM3dv_...Cv8-nlTX';
catalogParams.q = 'name:Desert';
console.log('catalogParams',catalogParams);
myPlayer.catalog.get(catalogParams,function(errorObj,videosReturned){
console.log('videosReturned',videosReturned);
myPlayer.playlist(videosReturned);
});
});
</script>
The following screenshot shows the two console.log()
results in the code above. The catalogParams
shows the parameters for the search, and the videosReturned
array shows the three videos returned by the search.
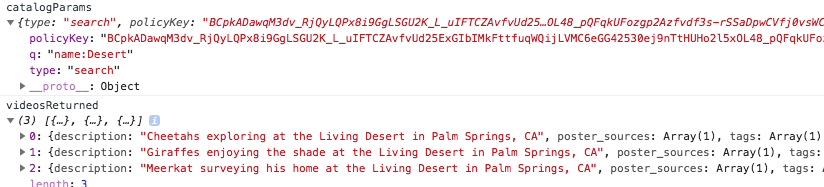
Promise example
In this code the same search is done using get()
method as shown just above, but the result is handled as a Promise.
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
catalogParams = {};
catalogParams.type = 'search';
catalogParams.policyKey = 'BCpkADawqM3dv_...Cv8-nlTX';
catalogParams.q = 'name:Desert';
myPlayer.catalog.get(catalogParams).then(function(videosReturned){
console.log('videosReturned',videosReturned);
myPlayer.playlist(videosReturned);
}).catch(function(errorObj){
console.log('errorObj',errorObj);
})
});
</script>
For more information on Promises, see Using JavaScript Promises.
getSearch() method
The getSearch(params, [callback], [adConfigId]) ⇒ XMLHttpRequest
method permits searching the catalog using CMS/Playback API: Videos Search syntax. Under the hood, this method uses the Playback API.
Parameters
-
params: An object of type catalog parameters.
-
callback: A function that will be called when the request completes, whether it's successful or not. It is invoked with two arguments:
-
The first argument will contain an error object, if an error occurs. This object contains details of the error. If no error occurs, this object is null.
-
If no error occurs, the second argument is the requested video object. If an error occurs, this value contains the empty string.
-
- [adConfigId]: DEPRECATED A Video Cloud ad configuration ID that will be passed along to the Playback API. Instead, pass the adConfigId in the initial params object.
Returned value
The catalog
call itself returns an object of type XMLHttpRequest. Although the call returns an XMLHttpRequest object, you will most likely never deal with that object. The reason is that in the callback function the JSON response is automatically parsed and a usable object is what is passed to the callback function. The exact structure of the returned data in the callback will vary depending what was searched for.
Example
The following example does the following:
-
Builds a CatalogParams object that will be passed as an argument with the function. The search-enabled policy key is passed. The search will query for videos with the string desert in the video's name.
-
Performs the search using the
getSearch()
method. Note that the callback function will use the parameters namederrorObj
andvideosReturned
. -
Use the
playlist()
method to assign the returned videos to the Brightcove Player.
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
catalogParams = {};
catalogParams.type = 'search';
catalogParams.policyKey = 'BCpkADawqM3dv_...-rSSaDpwCVfj0vsWCv8-nlTX';
catalogParams.q = 'name:Desert';
console.log('catalogParams',catalogParams);
myPlayer.catalog.getSearch(catalogParams,function(errorObj,videosReturned){
console.log('videosReturned',videosReturned);
myPlayer.playlist(videosReturned);
});
});
</script>
The following screenshot shows the two console.log()
results in the code above. The catalogParams
shows the required parameters for the search, and the videosReturned
array shows the three videos returned by the search.
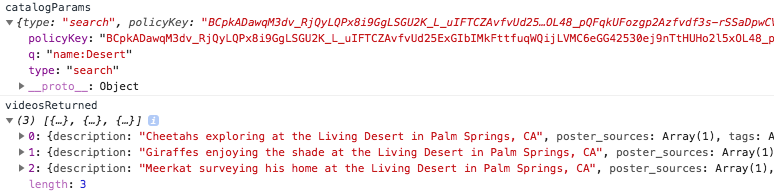
getSequence() method
The getSequence(sequences, callback, [adConfigId]) ⇒ Array.<XMLHttpRequest>
method permits passing multiple catalog
operations and retrieves all video objects from those multiple catalog
operations. You can have three types of operations, those being:
- search
- video
- playlist
In practical terms, this means you could retrieve the following combinations and the returned data is an array of video objects:
- Multiple playlists
- A playlist and several additional, individual videos
- Using search, all videos with a certain string in the name, description or tag.
Of course, the sequences just listed are only a few of a nearly infinite number of combinations.
Parameters
-
sequences: An object of type catalog parameters OR an array of objects representing a sequence of
catalog
operations. -
callback: A function that will be called when the request completes, whether it's successful or not. It is invoked with two arguments:
-
The first argument will contain an error object, if an error occurs. This object contains details of the error. If no error occurs, this object is null.
-
If no error occurs, the second argument is the requested video object. If an error occurs, this value contains the empty string.
-
-
[adConfigId]: An ad configuration ID that will be passed along with each request.
Note that if a specific video is returned in more than one of the operations, it will be in the result multiple times.
Returned value
The catalog
call itself returns an object of type XMLHttpRequest. Although the call returns an XMLHttpRequest object, you will most likely never deal with that object. The reason is that in the callback function the JSON response is automatically parsed and a usable object is what is passed to the callback function. The exact structure of the returned data in the callback will vary depending what was searched for.
Example
The following example uses a sequence of three catalog
operations. The first is a search for the 10 oldest videos in the catalog
, the second an individual video is added, and lastly a playlist's videos are added to the result.
<script>
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
// Declare object for catalog search
catalogParams = {};
// Declare mySequences array which will hold
// three different types of catalog operations
mySequences = [];
// Declare 3 objects for different catalog operations
mySequences[0]={};
mySequences[1]={};
mySequences[2]={};
// Define the object for catalog search
// Search will retrieve 10 oldest videos in catalog
catalogParams.policyKey = 'BCpkADawqM3dv_-rSSaDpwCVfj0vsWCv8-nlTX';
catalogParams.q = 'type:*';
catalogParams.sort = 'created_at';
catalogParams.limit = 10;
catalogParams.type = 'search';
mySequences[0] = catalogParams;
// Create a video retrieval catalog operation
catalogParams = {};
catalogParams.type = 'video';
catalogParams.id = '5755775186001';
mySequences[1] = catalogParams;
// Create a playlist retrieval catalog operation
catalogParams = {};
catalogParams.type = 'playlist';
catalogParams.id = '5531423971001';
mySequences[2] = catalogParams;
// Display mySequences array
console.log('mySequences',mySequences);
// Perform sequence of catalog operations
myPlayer.catalog.getSequence(mySequences,function(errorObj,videosReturned){
// Display video objects returned
console.log('videosReturned',videosReturned);
// Assign video objects as a playlist
myPlayer.playlist(videosReturned);
});
});
</script>
The following screenshot shows the structure of the mySequences
array, followed by the array of video objects returned:
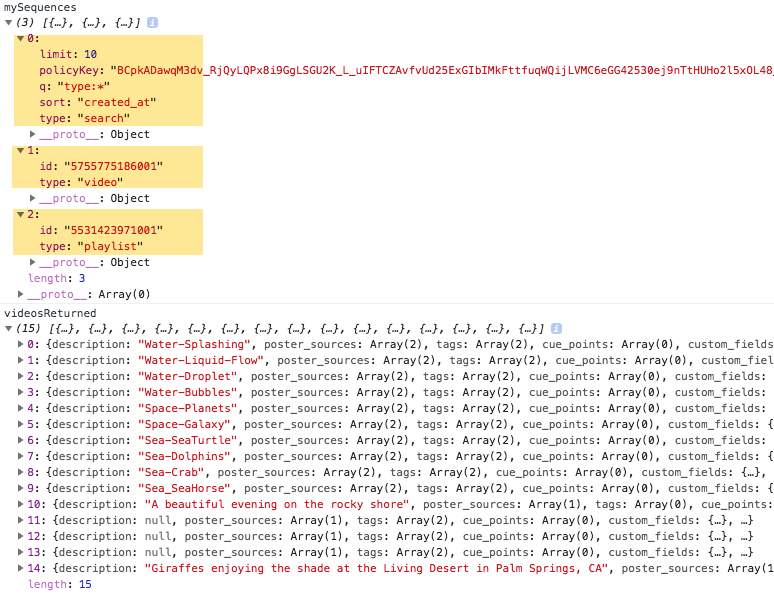
getLazySequence() method
The getLazySequence(sequences, callback, [adConfigId]) ⇒ XMLHttpRequest
method behaves the same as the getSequence() method, except that lazy loading of videos is employed. This means that when the videos for a sequence operation are retrieved, the next sequence operation's videos won't be retrieved until the last video from the previous operation is reached. A special case exists if a sequence returns only a single video, in which case the next sequence operation is performed and more videos are retrieved.
autoFindAndLoadMedia() method
The autoFindAndLoadMedia([opts]) ⇒ Object | undefined
method looks for the last-defined video or playlist ID, requests it from the API, and loads it into the player. This method defines the default behavior for the Brightcove Player.
Parameters
options
: Any of the follow options are recognized:-
adConfigId
(number): A numeric value for an ad configuration that will be passed along to the playback API. Will be called ad_config_id in the end. -
embedHasPoster
(boolean): Whether or not the original embed had a poster. -
embedHasSources
(boolean): Whether or not the original embed had sources. -
embedHasTracks
(boolean): Whether or not the original embed had tracks. -
playlistId
(string): A playlist ID provided in the player configuration. -
search
(string | Object): A search string/object that was provided in the player config or embed. Seeget()
method for more info on the supported parameters. -
sequence
(Object | Array.Object): A search string/object that was provided in the player config or embed. Seeget()
method for more info on the supported parameters. -
videoId
(string): A video ID provided in the player configuration.
-
The following example gets the video object based on the videoId
and loads it into the player:
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.videoId = '5141730843001';
myPlayer.catalog.autoFindAndLoadMedia(options);
});
</script>
The following example gets the playlist object based on playlistId
and loads it into the player. It also gets the video object based on playlistVideoId
and loads it as the first video to play in the playlist:
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.playlistId = '4845949311001';
options.playlistVideoId = '4845831078001'
myPlayer.catalog.autoFindAndLoadMedia(options);
});
</script>
transformVideoResponse( ) method
The transformVideoResponse(data)
method converts a raw video object from either the CMS API or the Playback API into a format compatible with the player and browsers. This includes duration times and https image compatibility. It also re-arranges the sources to ensure that the sources that are expected to provide the highest quality viewing experience on this platform are attempted first.
Parameters
-
data
: A raw video object parsed from acatalog
API response.
An example use of the method follows:
for (var i=0; i<limitValue; i++) {
mediaData.videos[i] = myPlayer.catalog.transformVideoResponse (mediaData.videos[i], myPlayer);
}
For the complete code sample, see the Playback API Paging document.
Returned value
An object that is more consistent with the player and browser environment.
catalog events
There are three catalogs events for which you can listen. They are:
-
catalog_request
: An event dispatched when a request to thecatalog
is made. -
catalog_response
: An event dispatched when a response from thecatalog
is received. The JSON formatted response contains the raw data the catalog API returned. See the Response information content later in this section for more details. -
catalog_request_error
: An event dispatched when there is an error when interacting with thecatalog
.
The following shows the console log of the events from a request to, and response from, the catalog
:
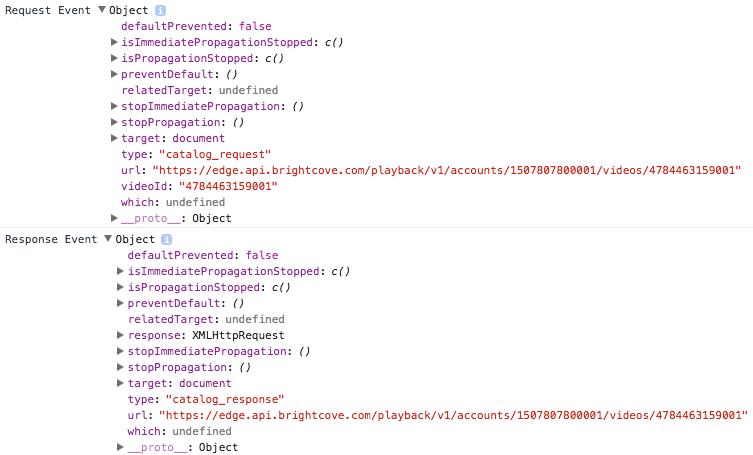
You see many standard properties of an event object. Of special interest for catalog
events is type and url.
The following shows a console log of a catalog
request error object:
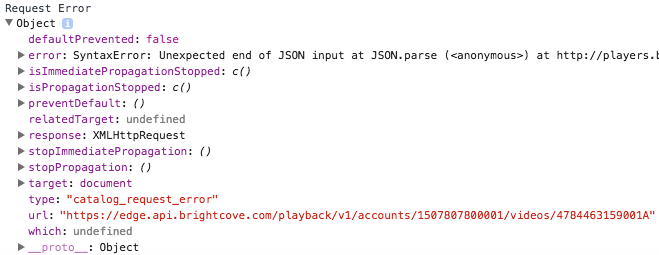
Response information
The catalog_response
event object contains a JSON formatted response
property that contains the raw data from the catalog API response. The following shows the console log of the response property:
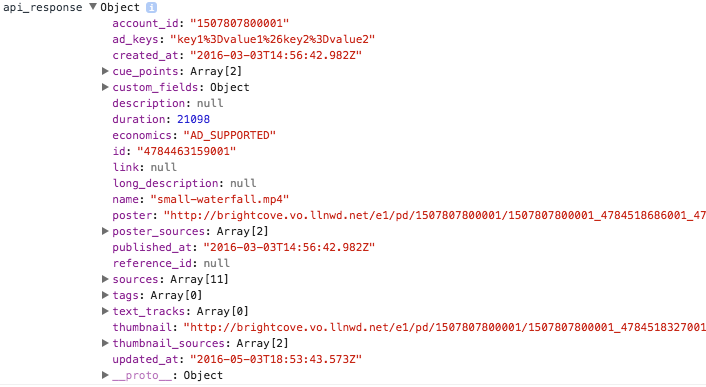
To actually use data from the response property, you need to parse the raw JSON formatted string into an object. The following shows accessing the name
property:
myPlayer.on('catalog_response',function(evt){
var api_response = JSON.parse(evt.response.response);
console.log('api_response.name',api_response.name);
});
Below is the JSON of an example response
property:
{
"description": null,
"poster_sources": [{
"src": "http://brightcove.vo.llnwd.net/e1/pd/1507807800001/1507807800001_4784518686001_4784463159001-vs.jpg?pubId=1507807800001&videoId=4784463159001"
}, {
"src": "https://brightcove.hs.llnwd.net/e1/pd/1507807800001/1507807800001_4784518686001_4784463159001-vs.jpg?pubId=1507807800001&videoId=4784463159001"
}],
"tags": [],
"cue_points": [{
"id": "4784326156001",
"name": "First cue point",
"type": "CODE",
"time": 3.0,
"metadata": "5;This is the first CTA;http://www.brightcove.com",
"force_stop": false
}, {
"id": "4784326155001",
"name": "Second cue point",
"type": "CODE",
"time": 13.0,
"metadata": "3;This is the second CTA;http://docs.brightcove.com/",
"force_stop": false
}],
"custom_fields": {},
"account_id": "1507807800001",
"sources": [{
"avg_bitrate": 513000,
"width": 480,
"duration": 21098,
"size": 1357587,
"stream_name": "mp4:1507807800001/1507807800001_4784519206001_4784463159001.mp4&1483545600000&c190f37500f15373c964858e54b4e2a1",
"codec": "H264",
"asset_id": "4784519206001",
"container": "MP4",
"height": 270,
"app_name": "rtmp://brightcove.fcod.llnwd.net/a500/e1/uds/rtmp/ondemand"
}, {
"avg_bitrate": 513000,
"width": 480,
"src": "http://brightcove.vo.llnwd.net/e1/uds/pd/1507807800001/1507807800001_4784519206001_4784463159001.mp4?pubId=1507807800001&videoId=4784463159001",
"size": 1357587,
"height": 270,
"duration": 21098,
"container": "MP4",
"codec": "H264",
"asset_id": "4784519206001"
}, {
"avg_bitrate": 1804000,
"width": 960,
"src": "https://brightcove.hs.llnwd.net/e1/uds/pd/1507807800001/1507807800001_4784519221001_4784463159001.mp4?pubId=1507807800001&videoId=4784463159001",
"size": 4752091,
"height": 540,
"duration": 21098,
"container": "MP4",
"codec": "H264",
"asset_id": "4784519221001"
}, {
"type": "application/x-mpegURL",
"src": "http://c.brightcove.com/services/mobile/streaming/index/master.m3u8?videoId=4784463159001&pubId=1507807800001",
"container": "M2TS",
"codec": "H264"
}, {
"type": "application/x-mpegURL",
"src": "https://secure.brightcove.com/services/mobile/streaming/index/master.m3u8?videoId=4784463159001&pubId=1507807800001&secure=true",
"container": "M2TS",
"codec": "H264"
}],
"name": "small-waterfall.mp4",
"reference_id": null,
"long_description": null,
"duration": 21098,
"economics": "AD_SUPPORTED",
"published_at": "2016-03-03T14:56:42.982Z",
"text_tracks": [],
"updated_at": "2016-05-03T18:53:43.573Z",
"thumbnail": "http://brightcove.vo.llnwd.net/e1/pd/1507807800001/1507807800001_4784518327001_4784463159001-th.jpg?pubId=1507807800001&videoId=4784463159001",
"poster": "http://brightcove.vo.llnwd.net/e1/pd/1507807800001/1507807800001_4784518686001_4784463159001-vs.jpg?pubId=1507807800001&videoId=4784463159001",
"link": null,
"id": "4784463159001",
"ad_keys": "key1%3Dvalue1%26key2%3Dvalue2",
"thumbnail_sources": [{
"src": "http://brightcove.vo.llnwd.net/e1/pd/1507807800001/1507807800001_4784518327001_4784463159001-th.jpg?pubId=1507807800001&videoId=4784463159001"
}, {
"src": "https://brightcove.hs.llnwd.net/e1/pd/1507807800001/1507807800001_4784518327001_4784463159001-th.jpg?pubId=1507807800001&videoId=4784463159001"
}],
"created_at": "2016-03-03T14:56:42.982Z"
}
catalog errors
The catalog
will throw errors when there are issues retrieving or initially playing the video or playlist. The errors are detailed below.
catalog Error | Description |
---|---|
Video ID as string |
The player has been configured with an invalid Video Cloud account ID. |
VIDEO_CLOUD_ERR_RESOURCE_NOT_FOUND |
The default playlist ID is invalid. |
VIDEO_CLOUD_ERR_VIDEO_NOT_FOUND |
The default video is invalid. |
VIDEO_CLOUD_ERR_NOT_PLAYABLE |
The Video Cloud video is not playable. Frequent causes of this error include when a video ID is deactivated or when the scheduled availability has expired. |
Handle bc-catalog-error
It is possible that handling errors in the normal ready()
section in the script
block can cause issues. For instance, it can happen that the bc-catalog-error
event could be dispatched before the player is ready, and if you listen for the error in the ready()
section, you will not be able to handle the error. This issue can occur when using geo-filtering, a video is unpublished, a video is out of scheduling range, or in a different account. You may find that there is not a problem in your code, but the issue can browser dependent, so test thoroughly.
If this is a possibility in your implementation, your error handling code should use the one()
event handling method to listen for the bc-catalog-error
in a separate code block rather than inside the ready()
section.
In the code sample below, you see a code block handle the bc-catalog-error
(line 62), and also a code block for ready
(line 71).
<video-js id="myPlayerID"
data-account="1486906377"
data-player="77a8e8b7-e8d1-4a3c-8a1b-292ba8233006"
data-embed="default"
data-video-id="4040394419001"
controls=""></video-js>
<p id="textTarget"></p>
<script src="https://players.brightcove.net/1486906377/77a8e8b7-e8d1-4a3c-8a1b-292ba8233006_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function(){
var myPlayer = this,
specificError;
if (myPlayer.catalog.error !== undefined) {
specificError = myPlayer.catalog.error.data[0];
console.log('bc-catalog-error:', specificError);
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
console.log('in ready');
});
</script>
Using custom error
The best solution to handle a specific bc-catalog-error maybe using a custom error. The following code shows a plugin that uses a custom error to notify a viewer of an inactive video why the video won't play:
videojs.registerPlugin('inactiveErrorCheck', function(options) {
var myPlayer = this;
myPlayer.one('bc-catalog-error', function(){
var specificError;
myPlayer.errors({
'errors': {
'inactive-error': {
'headline': options.inactiveMessage,
'dismiss': false
}
}
});
if (typeof(myPlayer.catalog.error) !== 'undefined') {
specificError = myPlayer.catalog.error.data[0];
if (specificError !== 'undefined' & specificError.error_code == "VIDEO_NOT_PLAYABLE") {
myPlayer.error({code:'inactive-error'});
};
};
});
});
The code to use the plugin, utilizing an in-page embed player implementation, follows:
<video-js id="myPlayerID" data-video-id="5350958927001"
data-account="1507807800001"
data-player="default"
data-embed="default"
data-application-id=""
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/default_default/index.min.js"></script>
<script type="text/javascript" src="inactive-error-check.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options =[];
options.inactiveMessage = "This is the message to display for inactive videos.";
myPlayer.inactiveErrorCheck(options);
});</script>
If you are adding the plugin via the PLUGINS section in Studio, you would supply the correct URL where you stored your JavaScript code, then in the Name, Option (JSON) entry supply:
-
Name: inactiveErrorCheck
-
Options: { "inactiveMessage": "This is the message to display for inactive videos." }
Handle geo-filtering error
As mentioned earlier geo-filtering can cause catalog errors. You can handle them very similarly to what was just shown. Code will be shown that puts a message below the "errored" player, as shown here:
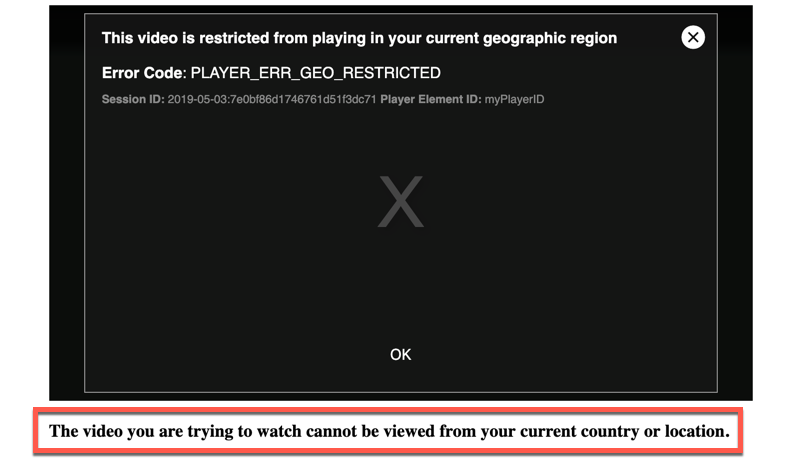
The following code catches the catalog error, then checks to see if it is a geo-filtering issue. If it is, then an appropriate message is displayed in the HTML page, just below the player, as shown in the screenshot.
<video-js id="myPlayerID"
data-video-id="4040394419001"
data-account="1486906377"
data-player="XJhO7JGxY"
data-embed="default"
data-application-id=""
controls=""
width="640"
height="360"></video-js>
<script src="//players.brightcove.net/1486906377/XJhO7JGxY_default/index.min.js"></script>
<br>
<div id="textTarget"></div>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function() {
var myPlayer = this,
specificError;
if (myPlayer.catalog.error !== undefined) {
specificError = myPlayer.catalog.error.data[0];
console.log('myPlayer.catalog.error', myPlayer.catalog.error);
if (specificError !== undefined & specificError.error_subcode == 'CLIENT_GEO') {
document.getElementById('textTarget').innerHTML = '<strong>The video you are trying to watch cannot be viewed from your current country or location.</strong>';
};
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
console.log('in ready');
});
</script>
Handle IP restriction error
The following code is analogous to what was just shown above for geo-filtering, except for IP restrictions:
<video-js id="myPlayerID"
data-video-id="5981021521001"
data-account="5977711152001"
data-player="iYNDnCGt9"
data-embed="default"
data-application-id=""
controls=""
width="640"
height="360"></video-js>
<script src="//players.brightcove.net/5977711152001/iYNDnCGt9_default/index.min.js"></script>
<br>
<div id="textTarget"></div>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').one('bc-catalog-error', function() {
var myPlayer = this,
specificError;
if (myPlayer.catalog.error !== undefined) {
specificError = myPlayer.catalog.error.data[0];
console.log('myPlayer.catalog.error', myPlayer.catalog.error);
if (specificError !== undefined & specificError.error_subcode == 'CLIENT_IP') {
document.getElementById('textTarget').innerHTML = '<strong>The video you are trying to watch cannot be viewed from your current IP address.</strong>';
};
};
});
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
console.log('in ready');
});
</script>
Request limit
Since the API responses are cached, there is no rate limit on catalog
requests.