Overview
The error messages plugin allows the player to display user-friendly messages when it encounters an error. The default style sheet displays messages as a semi-transparent overlay on top of the video element itself. You can change existing message text and add your own styles. You can even create custom messages that are triggered when you want.
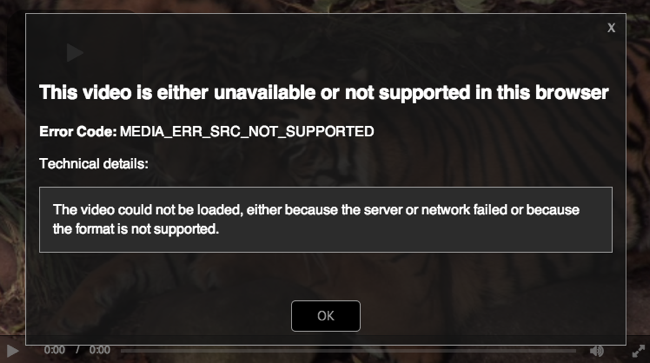
The error message shown in the image above was created by updating the player with an invalid src
value in the sources
property.
The error messages plugin is a default plugin and is automatically loaded with the Brightcove Player. However, you can choose not to load it. Without this plugin, you will see a limited set of error messages and some errors will only appear in the browser console. For details on how to not load a default plugin when you create a player, see the Player Plugin Overview document.
Get started
To update all instances of your player, you can implement a custom plugin using the Brightcove Players module from Studio. This approach is used in the following sections to update the error messages plugin for your player. If you choose to update this plugin from a page of code, it will only affect that instance of your player.
To update the plugin from your page code, first get a reference to the Brightcove Player. In this example, in the JavaScript we are creating a variable named myPlayer
and assigning it a reference to the player.
<video-js id="myPlayerID"
data-video-id="4443311217001"
data-account="1507807800001"
data-player="default"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/default_default/index.min.js"></script>
<script type="text/javascript">
videojs.getPlayer('myPlayerID').ready(function(){
var myPlayer = this;
Standard errors
This plugin has a set of default error messages for the standard HTML5 video errors based on the runtime error code value:
- Error code: 1
- Type: MEDIA_ERR_ABORTED
- Headline: The video download was cancelled
- Message: You aborted the media playback
- Error code: 2
- Type: MEDIA_ERR_NETWORK
- Headline: The video connection was lost, please confirm you're connected to the internet
- Message: A network error caused the media download to fail part-way. Currently most helpful for MP4 and/or progressive download video formats. See the Known issues section in this document for details.
- Error code: 3
- Type: MEDIA_ERR_DECODE
- Headline: The video is bad or in a format that can't be played on your browser
- Message: The media playback was aborted due to a corruption problem or because the media used features your browser did not support.
- Error code: 4
- Type: MEDIA_ERR_SRC_NOT_SUPPORTED
- Headline: This video is either unavailable or not supported in this browser
- Message: The media could not be loaded, either because the server or network failed or because the format is not supported.
- Error code: 5
- Type: MEDIA_ERR_ENCRYPTED
- Headline: The video you're trying to watch is encrypted and we don't know how to decrypt it
- Message: The media is encrypted and we do not have the keys to decrypt it.
If an error does not have an associated error code, a generic message is displayed:
- Error code: unknown
- Message: MEDIA_ERR_UNKNOWN
- Description: An unanticipated problem was encountered, check back soon and try again
Overriding text
There are three parts of the error message that you can change:
headline
: This is the message text at the top.type
: This is the Error Code: text.message
: This is the Technical details: text.
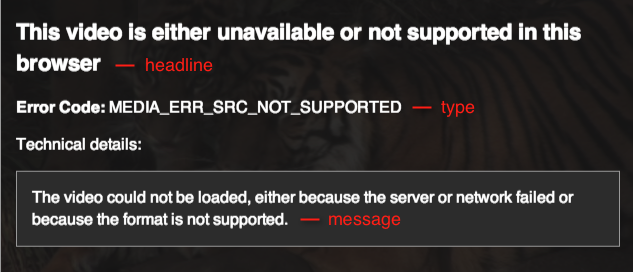
The example below shows how to override the message text for the standard error with an error code value of 4
. The properties are defined as follows:
plugins
: This property contains an array of properties and values. For this plugin, you only need to supply thename
property with the value oferrors
.options
: This property is used to pass data to the plugin.errors
: This property defines the error code that you want to update. Here we are overriding the message text for theheadline
,type
, andmessage
.
Using in page code
If you include the errors script in your code, you can override message text as follows:
myPlayer.errors({
"errors": {
"4": {
"headline": "This is a custom error message",
"type": "custom type",
"message": "these are details"
}
}
});
Using a custom plugin
If you want to update all instances of your player, create a custom plugin and add it to your player in Video Cloud Studio's Players module. For more information about plugins, see the Configuring Player Plugins document.
To create a plugin to override standard message text, follow these steps:
-
Create a JavaScript file stored in an Internet accessible location with your Brightcove Player plugin code. It should look similar to this, but with your own values:
videojs.registerPlugin('errorText', function() { var myPlayer = this; myPlayer.errors({ "errors": { "4": { "headline": "The Live Stream will begin soon", "type": "CUSTOM_TYPE", "message": "Please come back, once the live event has begun!" } } }); });
-
In the Players module, select Plugins from the left navigation.
-
Expand the Add a Plugin button, and select Custom Plugin.
Custom Plugin -
In the plugin details page, add values for:
- Plugin Name - Your plugin name
- JavaScript URL - The location of your player plugin from the first step
Plugin details - Select the Save button.
-
Publish your player.
Brightcove defined custom errors
Custom errors can also be defined. Brightcove has defined a number of custom errors, explained in this section, and you can also create custom errors, detailed in the next section.
- Brightcove recommends custom error code values be a string. You will see two of the provided errors use negative numbers for historical reasons, but alpha-numeric/descriptive strings are less likely to cause collision issues.
- Custom error messages can be named anything you want. Brightcove recommends that the type begin with
PLAYER_ERR
versus the standardizedMEDIA_ERR
to avoid confusion. - As detailed later in this section, you can make custom errors dismissible or not.
Five custom error messages have been added as a reference:
- Error code: -1
- Message: PLAYER_ERR_NO_SRC
- Description: No video has been loaded
- Error code: -2
- Message: PLAYER_ERR_TIMEOUT
- Description: Could not download the video
- Error code: Not set
- Message: PLAYER_ERR_DOMAIN_RESTRICTED
- Description: This video is restricted from playing on your current domain
- Error code: Not set
- Message: PLAYER_ERR_IP_RESTRICTED
- Description: This video is restricted at your current IP address
- Error code: Not set
- Message: PLAYER_ERR_GEO_RESTRICTED
- Description: This video is restricted from playing in your current geographic region
User defined custom errors
When defining your own custom errors Brightcove recommends you do not use a code. (You see in the section above that this is what Brightcove is now doing with the newer custom errors they are defining.) You should also consider using the PLAYER_ERR_
prefix for your custom errors for consistency's sake, but of course you can name them however you wish.
If you include the errors script in your code, you can add custom messages as follows:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
//custom error
myPlayer.errors({
"errors": {
"PLAYER_ERR_AGE_GATE": {
"headline": "You must be at least 18 years of age.",
"message": "Content may be considered inappropriate for some users."
}
}
});
Displaying custom errors
When you define custom errors, you need to let Brightcove Player know when you want to display it. To do this, create your own code to determine when the message should be displayed. Then use the error()
function to display the message as follows:
//display custom message
var age_appropriate = false;
myPlayer.on("loadstart", function () {
if(!age_appropriate) {
myPlayer.error({code:'PLAYER_ERR_AGE_GATE'});
}
});
Here the age_appropriate
variable is set to false
, but you would add your own code to determine when to display your custom error messages.
The error would be displayed to the user as follows:
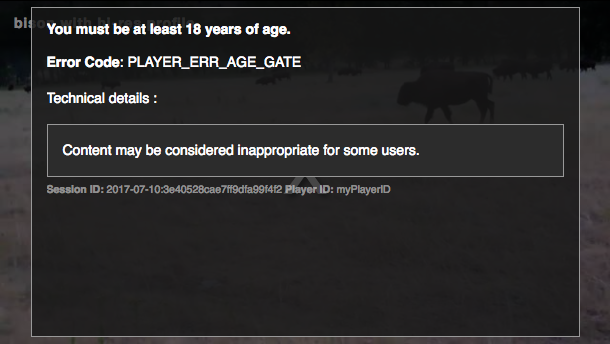
Making custom errors non-dismissible
By default a custom error message is dismissible by the video viewer. As the following screenshot shows, there is an OK button to click to dismiss the window, as well as an X in the top right corner.
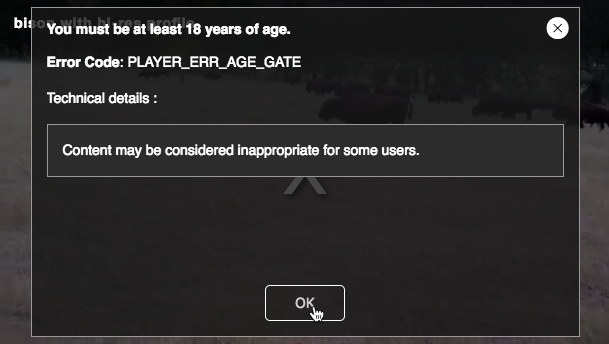
If you wish to NOT permit the video viewer to dismiss the error message, you can do that. When you call the error()
method, you can set the dismiss
property to false
.
myPlayer.error({code:'age-gate-error', dismiss: false});
When you do this the error message will appear as follows, with no way to dismiss the error.
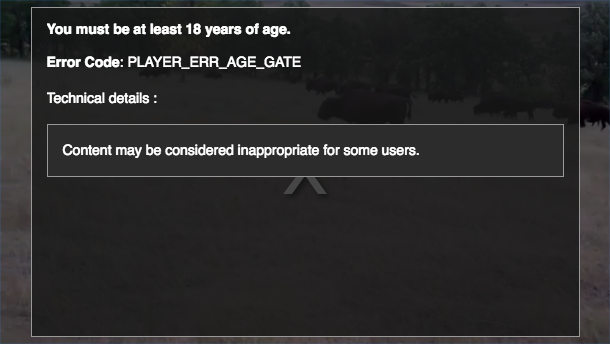
getAll() method
You can use the getAll()
method to see all the errors registered for a specific player. You can call the getAll()
method anytime after the errors plugin has been initialized, for instance after player.errors()
has been called. An example of calling the method is:
console.log('myPlayer.errors.getAll()',myPlayer.errors.getAll());
An example of the console display, with some errors expanded for details is:
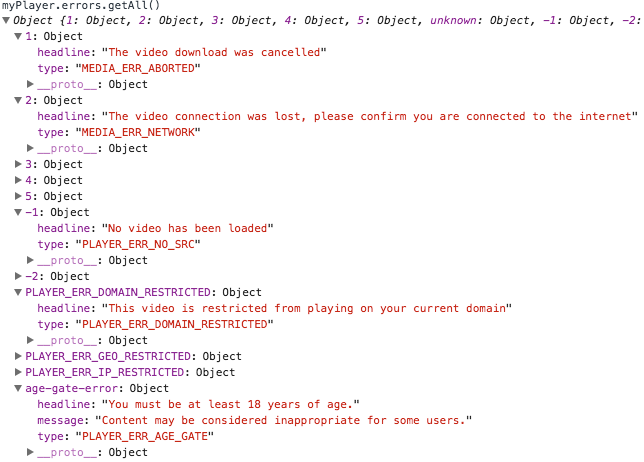
on()
method
You can also listen for all errors using the on()
method, using the following:
myPlayer.on('error'), function () {
...
}
If you are playing ads and want to catch all errors, you need to use this:
myPlayer.on(['error','aderror')], function () {
...
}
Dispatch errors
In development you may wish to dispatch errors to test if your configuration changes are successful. You can do this using code similar to that shown in the following code snippet. In this case, a button is added to the code so you can dispatch the error at a chosen time.
<video-js id="myPlayerID"
data-video-id="4443311217001"
data-account="1507807800001"
data-player="default"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1507807800001/default_default/index.min.js"></script>
<p><button onclick="changeVideo()">change video</button></p>
<script type="text/javascript">
var changeVideo;
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
changeVideo = function(){
myPlayer.error({code:'2'});
}
});
</script>
Localize errors
The errors plugin supports multiple languages. To add language support, after the plugin has loaded load the particular language file you wish to use:
<script src="lang/es.js"></script>
You can then use the techniques shown in the Programmatically Localizing Brightcove Player document to localize error messages.
bc-catalog-error
It is possible that handling errors in the normal ready()
section in the script block can cause issues. For instance, it can happen that the bc-catalog-error event could be dispatched before the player is ready, and if you listen for the error in the ready()
section, you will not be able to handle the error. This issue can occur when using geo-filtering, a video is inactive, a video is out of scheduling range, or in a different account. You may find that there is not a problem in your code, but the issue can browser dependent, so use caution.
For example, here is plugin code that displays a message when a video is inactive:
videojs.registerPlugin('inactiveErrorCheck', function() {
var myPlayer = this;
myPlayer.one('bc-catalog-error', function(){
var specificError;
myPlayer.errors({
'errors': {
'inactive-video-error': {
'headline': 'The video you are trying to watch is inactive.',
'dismiss': false
}
}
});
if (typeof(myPlayer.catalog.error) !== 'undefined') {
specificError = myPlayer.catalog.error.data[0];
if (specificError !== 'undefined' & specificError.error_code == "VIDEO_NOT_PLAYABLE") {
myPlayer.error({code:'inactive-video-error'});
};
};
});
});
Exaggerated error rate
If you are getting what seems like an unreasonable number of reported errors, it is important to know that you can get duplicate error events for the same session, creating this exaggerated error rate. Brightcove Player sends errors to analytics at the exact time, and in the same quantity, as they are reported to the player. For instance, if there is no media in a player, and code somehow calls play()
one thousand times in a row, one thousand PLAYER_ERR_NO_SRC errors will be reported to analytics.
If there are a few sessions with tons of errors skewing the analytics, you should consider using the following logic to get a better sense of the actual issues:
number_of_sessions_with_errors / total_number_of_sessions
rather than
count of errors/number of views
Known issues
- When loading videos in Edge on Windows 10 (both within the Studio and in public URLs), a MEDIA_ERR_SRC_NOT_SUPPORTED error is displayed and the video cannot be played.
-
On Android devices and iPhones, the tap events for error messages do not bubble up to the parent video element. This means that you can not close an error message once it appears. This behavior may be a problem if the user is in fullscreen mode, because they will have no way to leave this state.
This issue is currently being worked on and should be fixed in a future player release. For now, you can try a work-around like this:
player.on("touchstart", function(e) { if (player.error_) { player.error(null); e.preventDefault(); } })
Changelog
The errors plugin is now integrated into the player and changes to the plugin functionality will be reported in Brightcove Player release notes.
For historical release notes, see the changelog here.