Overview
The Playlist UI Plugin contains the options you can use to customize playlist behavior. The numerous options provide changes to playlists, including their layout, behaviors and implementation strategies. The following examples show the two basic layouts for playlists, vertical and horizontal. For each example a small set of behaviors are listed for an introduction of what can be customized.
Vertical layout
This playlist example below demonstrates:
- The vertical playlist located on the right side of the player has a show/hide button and the playlist is automatically sized and positioned. This is default behavior when using the Standard (iframe) player implementation.
- The playlist is initially displayed. This can be altered by using the
hideOnStart
option. -
Near the end of the video an overlay appears that displays the time to the next video and the next video's thumbnail. This is default behavior and
controlled via the
nextOverlay
option.
Horizontal layout
This playlist example below demonstrates:
- A horizontal playlist located below the video.
-
As well as the overlay being displayed near the end of the video, as is true in the example above, an endscreen shows the time before the next video
begins playing. This is because the
autoadvance
option is non-zero and the endscreen displays for the pause between videos. The endscreen can be altered by using thenextEndscreen
option.
Player/Playlist association
By default, the Playlist UI Plugin will handle finding the correct playlist container element for a given player. This means it will find the first empty
.vjs-playlist
element in the DOM and use that. However, the plugin offers more explicit associations that can be made
between a player and playlist container when you are building complex workflows with multiple players.
data-for
attribute
The data-for
attribute can be applied to the playlist container to associate it with a player's
id
, for example:
<video-js id="myPlayerID"
...></video-js>
<div class="vjs-playlist" data-for="myPlayerID"></div>
This is the most specific method of explicit association available. It takes precedence over other method(s).
data-player
and data-embed
attributes
The data-player
and data-embed
attributes can be applied to the playlist container to
associate it with a Brightcove Player. Note that both attributes must be used for the associate to function properly. In the following example, only the
second <div>
tag will hold the playlist for the player as a specific association is made. The first
<div>
will be empty.
<video-js data-playlist-id="5455901760001"
data-account="1507807800001"
data-player="SJLNAJye7"
data-embed="default"...></video-js>
<script src="https://players.brightcove.net/1507807800001/SJLNAJye7_default/index.min.js"></script>
<div class="vjs-playlist"></div>
<div class="vjs-playlist" data-player="SJLNAJye7" data-embed="default"></div>
Add/Remove playlist items
The Playlist UI plugin supports adding and removing playlist items individually instead of only allowing complete replacement of the entire playlist. The add
and remove
methods are now added to the playlist object. And it also adds two new events playlistadd
and playlistremove
.
These methods and events were introduced via the underlying open-source videojs-playlist plugin, which is bundled into this plugin. You can refer to the open-source documentation for specifics. Here is an example of how these new methods might be used:
var samplePlaylist = [{
sources: [{
src: 'aaa.mp4',
type: 'video/mp4'
}]
}];
player.playlist(samplePlaylist);
// Log the playlistadd and playlistremove events
player.on('playlistadd', 'playlistremove', ({count, index, type}) => {
player.log(`saw ${type} event`, count, index);
player.log(`playlist total length is now ${player.playlist().length}`);
});
// Adding one item to a playlist.
// Playlist will contain two items after this call: aaa.mp4, bbb.mp4
player.playlist.add({
sources: [{
src: 'bbb.mp4',
type: 'video/mp4'
}]
});
// Adding multiple items to a playlist at index 1.
// Playlist will contain four items after this call: aaa.mp4, ccc.mp4, ddd.mp4, and bbb.mp4
player.playlist.add([{
sources: [{
src: 'ccc.mp4',
type: 'video/mp4'
}]
}, {
sources: [{
src: 'ddd.mp4',
type: 'video/mp4'
}]
}], 1);
// Removing a single item from a playlist.
// Playlist will contain three items after this call: ccc.mp4, ddd.mp4, and bbb.mp4
player.playlist.remove(0);
// Removing multiple items from a playlist.
// Playlist will contain one item after this call: bbb.mp4
player.playlist.remove(1, 2);
Options
You may pass in an options object to the plugin upon initialization. This object may contain any of the following options:
autoadvance
-
autoadvance
:- Type:
number
- Default:
undefined
- Determines if, and for how long, a pause between videos in a playlist occurs. For complete details see the autoadvance section in the Playlist API Guide.
- Type:
hideOnStart
-
hideOnStart
:- Type:
boolean
- Default:
false
- Determines whether the playlist is initially hidden from view. This is only functional when using the iframe player implementation, which is logical since the show/hide playlist functionality is only available in the iframe. This option does NOT work with a horizontal playlist.
-
Requirements/dependencies:
playlistPicker
:true
- iframe embed
horizontal
:false
- Type:
horizontal
-
horizontal
:- Type:
boolean
- Default:
false
- Displays the playlist horizontally below the player instead of vertically.
-
Requirements/dependencies:
playlistPicker
:true
- Type:
nextButton
-
nextButton
:- Type:
boolean
- Default:
true
-
If
true
, a button is added for moving to the next playlist item. The button can be disabled by setting the option tofalse
. The button is added to the control bar to the right of the play button.
- Type:
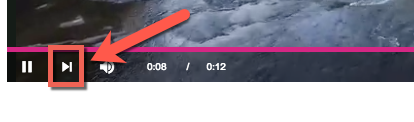
nextEndscreen
nextEndscreen
:- Type:
boolean
- Default:
true
-
If
true
, the player will display a modal endscreen over the player after playback. The endscreen can be disabled by setting the option tofalse
. This endscreen will preview the upcoming video in the playlist. Mode details on the option are:-
The
autoadvance
option must be set to a value greater than zero (otherwise, there is no time to display the endscreen and it is skipped). - The displayed countdown represents the time remaining until the
autoadvance
trigger. - The player must not include an implementation of the custom endscreen plugin or the social plugin configured to display after a video. If either condition is detected, the endscreen will not be displayed.
-
The
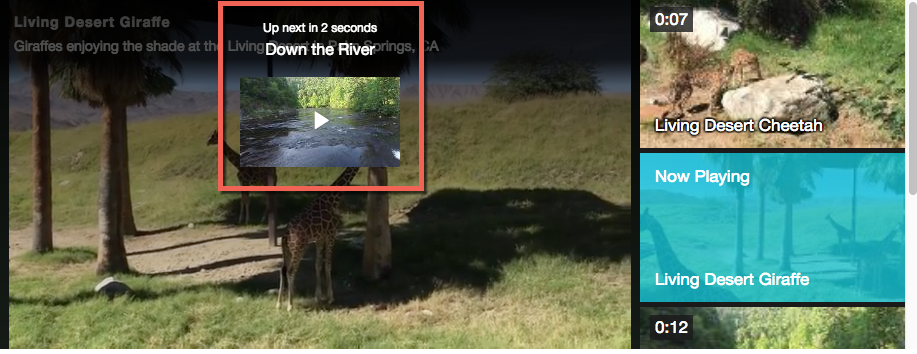
nextOverlay
-
nextOverlay
:- Type:
boolean
- Default:
true
-
If
true
, the player will display a small overlay in the lower-right corner of the player. The overlay can be disabled by setting the option tofalse
. This overlay will preview the upcoming video in the playlist. Mode details on the option are:- There must be a subsequent playlist item.
- The
autoadvance
option must be set greater than or equal to zero. - The countdown represents the time remaining in the video plus any
autoadvance
wait time. - The overlay appears 10 seconds from the end of the video. However, if the video is less than 30 seconds long, it appears at the 2/3 point of the duration.
- If the overlay is dismissed by the user, it will remain dismissed until the source is changed. It will re-appear for new sources.
- If the Up Next endscreen is enabled, the overlay will hide when the video ends. Otherwise, it will stay open until the next video.
- Type:
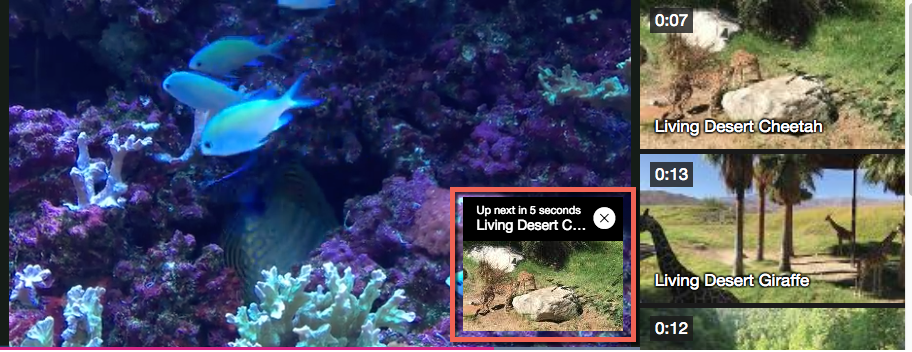
playlist
-
playlist
:- Type:
array
- Default:
undefined
- Used to pass the initial playlist data. For complete details see the playlist section in the Playlist API Guide.
- Type:
playlistPicker
-
playlistPicker
:- Type:
boolean
- Default:
true
-
Determines whether or not to include a visual list of videos in the playlist so the user can click on them. The
hideOnStart
andhorizontal
options modify its appearance and behavior. WhenplaylistPicker
isfalse
, other UI elements can still be shown using thenextButton
,nextEndscreen
andnextOverlay
options.
- Type:
playOnSelect
-
playOnSelect
:- Type:
boolean
- Default:
false
-
The
playOnSelect
option is used to control if the video should begin playing when the user clicks a video in the playlist. When the option is set totrue
, selecting a new video from the playlist will start playing that video, even if the player was previously paused. By default, clicking a new video from the playlist will load the new video but keep the player paused if it was paused beforehand. -
Requirements/dependencies:
playlistPicker
:true
- Type:
repeat
-
repeat
:- Type:
boolean
- Default:
false
- Repeats a playlist after it has finished the last video in the playlist. This functionality plays the first video in the playlist once the last video in the playlist is finished.
- Type:
showDescription
-
showDescription
:- Type:
boolean
- Default:
false
- Display video descriptions for each playlist item.
- Type:
shuffle
-
shuffle
:- Type:
boolean
- Default:
false
- Shuffle the playlist items each time new data is loaded.
- Type:
Using the options
You have two ways you can utilize the option:
- In Studio's PLAYERS > PLUGINS section.
- Using JavaScript with the player.
Using Studio
In Studio, if you have selected the player to use playlists in the player properties' Styling section you can set some of the above options in the UI. The following options are available in the Playback section for playlist players:
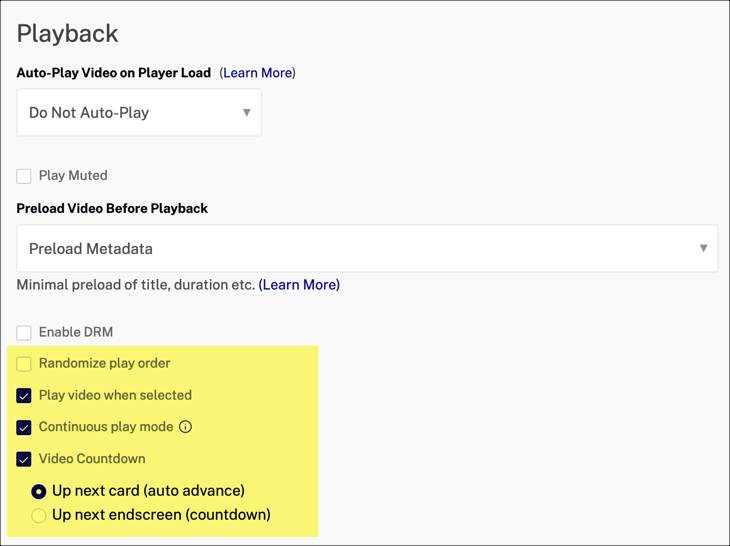
If you select Continuous play mode you can choose a Video Countdown option. The visual representation of the choices is shown here:
Up next card
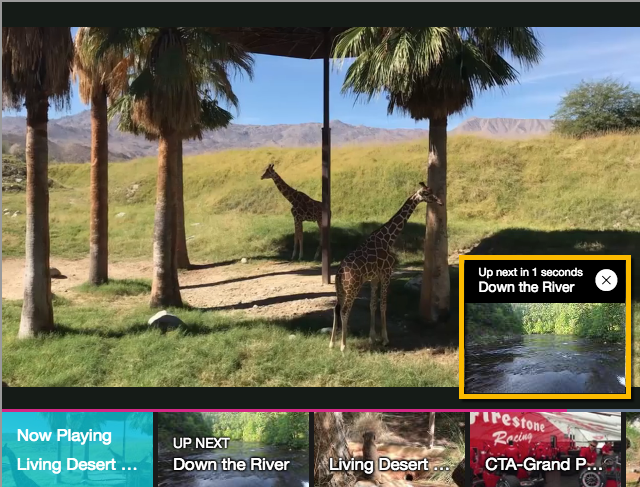
Up next endscreen
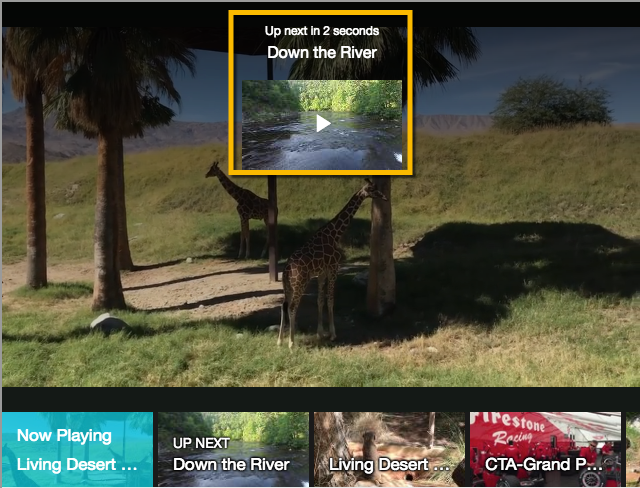
Using JavaScript
To implement the options in code, you create an object, assign desired options their respective values, then pass the options object when calling the plugin:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this,
options = {};
options.horizontal = true;
options.nextButton = false;
myPlayer.bcPlaylistUi(options);
});
Changelog
See the Playlist UI Plugin Release Notes.
For historical release notes, see the changelog here.