Localizing Plugins
Add language(s)
You can choose the languages to support in a plugin. For each language you wish to support you must have a set of key-value pairs with the English language as the key and the corresponding localized language as a value. You do this by using Videojs' addLanguage()
method. The following code shows adding German translations to a page:
videojs.addLanguage('de', {
"Hello": "Guten Tag",
"Goodbye": "Auf Wiedersehen",
});
Localize content
Once you have added the languages and vocabulary you wish to use in the localization process you can use the player's localize()
method to perform the actual localization. The following shows a string being injected into an HTML <span>
, then appended to the end of the video tag:
textSpan = document.createElement('span');
textSpan.className = "localizeStyle"
textSpan.innerHTML = myPlayer.localize("Hello") + "/" + myPlayer.localize("Goodbye");
myPlayer.el().appendChild(textSpan);
The localize()
method takes the English key word as a string argument, myPlayer.localize("Hello")
, and displays that string unless the browser's preferred language is available, in which case the localized word will be substituted.
Set display language
To display the localized text, the user must set the preferred language in the browser. The exact steps vary depending on browser and OS.
If the browser's preferred language is English, or a language not available, you will see the default, or English, text.
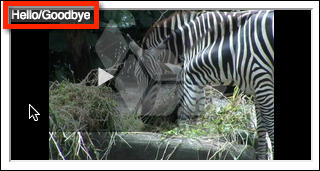
If the browser's preferred language is set to an available language, you will see the localized version, as shown here:
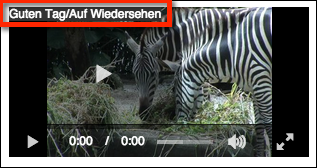
Plugin code
As documented in the Step-by-Step: Plugin Development, you put the code into a file that contains the plugin.
- Lines 27,37: Standard syntax to define a Brightcove Player plugin. Notice for later reference the name of the plugin is pluginLocalize.
- Lines 28-31: Adds German language localization.
- Lines 32-36: Dynamically creates an HTML
span
element, populates it and appends it to the player code.
videojs.registerPlugin('pluginLocalize', function() {
videojs.addLanguage('de', {
"Hello": "Guten Tag",
"Goodbye": "Auf Wiedersehen",
});
var myPlayer = this,
textSpan = document.createElement('span');
textSpan.className = "localizeStyle"
textSpan.innerHTML = myPlayer.localize("Hello") + "/" + myPlayer.localize("Goodbye");
myPlayer.el().appendChild(textSpan);
});
For testing purposes, use the plugin locally in a page that utilizes the embed_in_page player implementation.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>Localize Plugin Example</title>
<link href="localize-plugin.css" rel="stylesheet">
</head>
<body>
<video-js id="myPlayerID"
data-account="1752604059001"
data-player="9a2e3f23-d63b-43c3-aae9-21f11548e812"
data-embed="default"
data-video-id="3851389913001"
controls=""></video-js>
<script src="https://players.brightcove.net/1752604059001/9a2e3f23-d63b-43c3-aae9-21f11548e812_default/index.min.js"></script>
<script type="text/javascript" src="localize-plugin.js"></script>
<script>videojs.getPlayer('myPlayerID').pluginLocalize();</script>
</body>
</html>
Add plugin to player
Once you have confirmed the plugin is functioning correctly, you will want to add the plugin to the player. To do this, follow these steps:
- Open the PLAYERS module and either create a new player or locate the player to which you wish to add the plugin.
- Click the link for the player to open the player's properties.
- Click Plugins in the left navigation menu.
- Next, click Add a Plugin > Custom Plugin.
- For the Plugin Name enter
pluginLocalize
. - For the JavaScript URL, enter:
http://solutions.brightcove.com/bcls/brightcove-player/localization/localize-plugin.js
- For the CSS URL, enter:
http://solutions.brightcove.com/bcls/brightcove-player/localization/localize-plugin.css
- You do not have to enter any options for this plugin.
- Check to be sure your form appears similar to the following:
- Click Save.
- To publish the player, click Publish & Embed > Publish Changes.
- To close the open dialog, click Close.
Once you have saved and published, you can alter the configuration by click on the plugin name in the Plugins list.
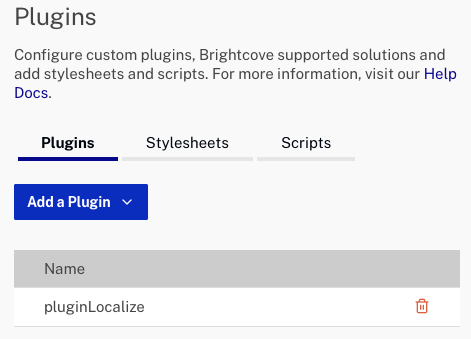
The plugin is now part of the player. This permits you to use the iframe player implementation with no other supporting code and have the plugin's functionality available.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>Localize Plugin - iframe</title>
</head>
<body>
<iframe src='https://players.brightcove.net/1752604059001/9a2e3f23-d63b-43c3-aae9-21f11548e812_default/index.html'
allowfullscreen allow='encrypted-media'></iframe>
</body>
</html>
Localizing Brightcove Plugins Loaded In-Page
When a Brightcove plugin is loaded in-page via a <script> rather than added in Studio, any language translations associated with that plugin will not automatically be included. If you wish to localize a Brightcove plugin that has been loaded in-page, you have to manually include the language files for each language you want to support.
This can be accomplished either by:
- Adding the language files as custom Scripts in Studio.
- Loading the language files in <script> tags after the player, before the plugin script.
The language file URLs have the following format:
https://players.brightcove.net/<plugin-name>/<plugin-version>/lang/<language-code>.js
Here is an example of the second approach using the Quality Selection plugin:
// Load the player
<video-js id="myPlayerID"
data-account="175260405001"
data-player="default"
data-embed="default"
controls=""
data-video-id="584280065001"
data-playlist-id=""
data-application-id=""
width="960" height="540">
<script src="https://players.brightcove.net/1752604059001/default_default/index.min.js"></script>
// Load translations for the Quality Selection plugin
<script src="https://players.brightcove.net/videojs-quality-menu/3/lang/fr.js"></script>
<script src="https://players.brightcove.net/videojs-quality-menu/3/lang/ko.js"></script>
<script src="https://players.brightcove.net/videojs-quality-menu/3/lang/zh-Hant.js"></script>
// Load the Quality Selection plugin
<script src="https://players.brightcove.net/videojs-quality-menu/3/videojs-quality-menu.min.js"></script>