Player example
You see a styled Brightcove Player for playing audio assets. After clicking play, you will see on the right side of the control bar a download icon. Clicking this icon will cause the audio asset playing to be downloaded via the browser.
There is a VERY similar sample using video rather than audio assets in the Brightcove Player Sample: Download Video Plugin document.
See the Pen Download Audio Plugin by Brightcove Learning Services (@rcrooks1969) on CodePen.
Source code
View the complete solution on GitHub.
Using the CodePen
Here are some tips to effectively use the above CodePen:
- Toggle the actual display of the player by clicking the Result button.
- Click the HTML/CSS/JS buttons to display ONE of the code types.
- Later in this document the logic, flow and styling used in the application will be discussed in the Player/HTML configuration, Application flow and Application styling sections. The best way to follow along with the information in those sections is to:
- Click the EDIT ON CODEPEN button in the CodePen and have the code available in one browser/browser tab.
- In CodePen, adjust what code you want displayed. You can change the width of different code sections within CodePen.
- View the Player/HTML configuration, Application flow and/or Application styling sections in another browser/browser tab. You will now be able to follow the code explanations and at the same time view the code.
Development sequence
Here is the recommended development sequence:
- Use the In-Page embed player implementation to test the functionality of your player, plugin and CSS (if CSS is needed)
- Put the plugin's JavaScript and CSS into separate files for local testing
- Deploy the plugin code and CSS to your server once you have worked out any errors
- Use Studio to add the plugin and CSS to your player
- Replace the In-Page embed player implementation if you determine that the iframe implementation is a better fit (detailed in next section)
For details about these steps, review the Step-by-Step: Plugin Development guide.
iframe or In-Page embed
When developing enhancements for the Brightcove Player you will need to decide if the code is a best fit for the iframe or In-Page embed implementation. The best practice recommendation is to build a plugin for use with an iframe implementation. The advantages of using the iframe player are:
- No collisions with existing JavaScript and/or CSS
- Automatically responsive
- The iframe eases use in social media apps (or whenever the video will need to "travel" into other apps)
Although integrating the In-Page embed player can be more complex, there are times when you will plan your code around that implementation. To generalize, this approach is best when the containing page needs to communicate to the player. Specifically, here are some examples:
- Code in the containing page needs to listen for and act on player events
- The player uses styles from the containing page
- The iframe will cause app logic to fail, like a redirect from the containing page
Even if your final implementation does not use the iframe code, you can still use the In-Page embed code with a plugin for your JavaScript and a separate file for your CSS. This encapsulates your logic so that you can easily use it in multiple players.
API/Plugin resources used
API Methods | API Events | API Properties |
---|---|---|
on() |
loadstart |
player.mediainfo |
player.controlBar.customControlSpacer |
Player/HTML configuration
This section details any special configuration needed during player creation. In addition, other HTML elements that must be added to the page, beyond the in-page embed player implementation code, are described.
Player configuration
No special configuration is required for the Brightcove Player you create for this sample.
Other HTML
No other HTML elements are added to the page.
Application flow
The basic logic behind this application is:
- Get audio asset name and extract the MP4 renditions from the sources array.
- Loop over all the renditions and extract the MP4 versions.
- Sort the MP4 array on the
size
attribute, largest to smallest. - Assign the largest rendition to a variable.
- Create a clickable image that downloads the rendition inside an HTML
div
element. - Place the download asset image element in the DOM.
Get audio asset name and extract the MP4 renditions from the sources array
Find the code which is labeled:
// ### Get video name and the MP4 renditions ###
First the videoName
variable is assigned the name of the video (with spaces removed) for use in the HTML anchor tag dynamically built later in the code. Next the renditionsAra
variable is assigned all the possible renditions for the video.
Of course, for this plugin to function correctly you must have at least one MP4 rendition in the sources.
Loop over all the video renditions and extract the MP4 versions
Find the code which is labeled:
// ### Loop over videos and extract only MP4 versions ###
Loop over the array and assign to the mp4Ara
variable renditions where the container
is MP4, AND the rendition has a src
property. The MP4 renditions appear twice in the sources
array, once with a src
property and once with a stream_name
property. The first is for progressive download and the second for streaming.
The following screenshots show the console displaying all renditions and then the filtered renditions showing on MP4 renditions.
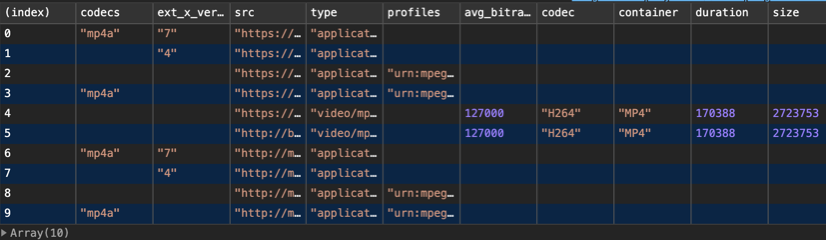

Sort the MP4 array on the size
attribute, largest to smallest
Find the code which is labeled:
// ### Sort the renditions from highest to lowest on size ###
Sort the mp4Ara
using JavaScript's sort()
method
Assign the largest video to a variable
Find the code which is labeled:
// ### Extract the highest rendition ###
Assign the highestQuality
variable the zeroth index value from the sorted MP4 renditions array.
Create an element that contains an clickable image inside an HTML div
element
Find the code which is labeled:
// ### Build the download image element ###
At the top of the JavaScript code HTML div
and img
elements are created and stored in variables. Those elements are then manipulated to dynamically build an HTML element that functions as a download button. An example of how the dynamically created element might appear follows:

Place the download button element in the DOM
Find the code which is labeled:
// ### Place the download button ###
In this case, the download button is placed in the controlbar's spacer. First, a reference to the spacer element is obtained, then an attribute set to position the new element in the spacer is defined, and lastly the new element placed in the spacer.
Application styling
The CSS does two jobs, they are:
- Styles the player for an "audio only" look.
- Sizes and positions the download button for placement in the controlbar.
Plugin code
Normally when converting the JavaScript into a Brightcove Player plugin nominal changes are needed. One required change is to replace the standard use of the ready()
method with the code that defines a plugin.
Here is the very commonly used start to JavaScript code that will work with the player:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
...
});
You will change the first line to use the standard syntax to start a Brightcove Player plugin:
videojs.registerPlugin('pluginName', function(options) {
var myPlayer = this;
...
});
As mentioned earlier, you can see the plugin's JavaScript code in this document's corresponding GitHub repo: download-audio.js.
Using the plugin with a player
Once you have the plugin's CSS and JavaScript files stored in an Internet accessible location, you can use the plugin with a player. In Studio's PLAYERS module you can choose a player, then in the PLUGINS section add the URLs to the CSS and JavaScript files, and also add the Name and Options, if options are needed.
Version 5 backwards compatibility
If you wish to have the plugin work with both versions 5 and 6 a few changes must be made to the JavaScript. The changes will be listed, then a skeleton of the JavaScript displayed.
- Add the following variable declaration at the top of the JavaScript file. The variable defines how to register a plugin for both version 5 and version 6:
var registerPlugin = videojs.registerPlugin || videojs.plugin;
- Change the old first line of the JavaScript from:
videojs.getPlayer('myPlayerID').ready(function() {
registerPlugin("downloadAudio", function() {
- Create a function below all existing code that calls the now defined plugin:
videojs.getPlayer('myPlayerID').ready(function() { var player = this; player.downloadAudio(); });
- Check to be sure the structure of the code appears as follows:
var registerPlugin = videojs.registerPlugin || videojs.plugin; registerPlugin("downloadAudio", function() { // Create variables and new div, anchor and image for download icon var myPlayer = this, videoName, totalRenditions, mp4Ara = [], highestQuality, spacer, newElement = document.createElement("div"), newImage = document.createElement("img"); // REST OF JavaScript ... }); videojs.getPlayer('myPlayerID').ready(function() { var player = this; player.downloadAudio(); });