Introduction
The IMA plugin integrates the Brightcove player with Google's Interactive Media Ads (IMA) for HTML5 version 3. This allows you to request and track VAST, VPAID and VMAP ads for your player. For details about Google IMA, see the Using the IMA HTML5 SDK Version 3 document.
Player sample
The sample video below demonstrates the use of the IMA Plugin. Play the video to see a pre-roll, a skippable mid-roll at 5 seconds, then finally a post-roll.
Testing the ad server
The first thing you should do is verify the validity of the ad tag you are planning to use. Be sure you have copied its URL and browse to the following page: Video Suite Inspector (clicking this link will cause the page to open in a new window or tab).
Paste your ad tag URL into the Input type form input field. Click Test Ad and you will see your ad(s) play, with the Google supplied video interspersed. If your ad tag is not working in this test environment, it will not work with Brightcove Player.
Implement using Players module - Advertising section
In this section of the document you will use Studio to implement advertising using the Advertising section. In this case, you are limited to what options the form provides. If you wish to customize the implementation using some of the many available options that happen NOT to be provided in this section, use the Implement using Players module - Plugins section, which provides the opportunity to supply options via JSON.
To implement the IMA Plugin using the Players module, follow these steps:
- Open the PLAYERS module and either create a new player or locate the player to which you wish to add advertising functionality.
- Click the link for the player to open the player's properties.
- Click Advertising in the left navigation menu.
- Check the Enable Client-Side (IMA) checkbox.
- Enter the Server URL for the ad server.
-
Select the Request Ads setting.
- On load - Ads are requested immediately when the player loads (this is normally the best experience for GAM / VPAID).
- On play - The first ad request is delayed until playback is initiated.
-
On demand - All ad requests will be initiated programmatically using the
player.ima3.adrequest()
method. This mode does not support preroll or postroll ads. - On cue point - Ad requests will be initiated on a dispatched ad cue point. See the Displaying Ads Using Ad Cue Points document for complete details.
-
Select the ad VPAID Mode. The VPAID Mode is used to enable VPAID 2 support in IMA ads.
- Enabled - Play VPAID ads in an iframe with a different domain
- Insecure - Play VPAID ads in an iframe with the same domain
- Disabled - VPAID ads throw an error
- Set the Timeout value. This is the maximum amount of time to wait, in milliseconds, for an ad to initialize before playback.
- Make a choice for Hard timeouts. Unchecking this option could result in a slow loading advertisement interrupting video playback.
- Set the Maximum Number of Redirects. This specifies the maximum number of redirects before the subsequent redirects will be denied, and the ad load aborted. The number of redirects directly affects latency and thus user experience.
- For the Plugin Version, it is strongly recommended you use the latest version.
-
View an example, completed form:
- Click Save.
- To publish the player, click Publish & Embed > Publish Changes.
- To close the open dialog, click Close.
When the changes to the advertising properties are saved, the IMA plugin will be configured as part of the Plugin settings. The JavaScript and CSS will be hidden since you added them via the Advertising section.
The IMA plugin does support additional properties that are not available in this section of the UI. See the next section of this document for a way to use more configuration options.
Implement using Players module - Plugins section
If you wish to configure the IMA3 plugin beyond the options provided in the Advertising section, you can use the Plugins section, which provides a way to supply options via JSON.
To implement the IMA3 Plugin you will add the function name of the plugin and the URLs to the plugin's JavaScript and CSS files:
- Open the PLAYERS module and either create a new player or locate the player to which you wish to add the plugin.
- Click the link for the player to open the player's properties.
- Click Plugins in the left navigation menu.
- From the Add a Plugin dropdown, select Custom Plugin.
- For the Plugin Name enter
ima3
. -
For the JavaScript URL, enter:
https://players.brightcove.net/videojs-ima3/4/videojs-ima3.min.js
-
For the CSS URL, enter:
https://players.brightcove.net/videojs-ima3/4/videojs-ima3.css
-
Enter the configuration options in the Options(JSON) text box.
{ "serverUrl": "//pubads.g.doubleclick.net/gampad/ads?sz=400x300&iu=%2F6062...", "timeout": 5000, "debug": true }
- Click Save.
- To publish the player, click Publish & Embed > Publish Changes.
- To close the open dialog, click Close.
JSON vs JavaScript notation
If you examine the Options section above, you see the configuration information is supplied in JSON format. If you are using code to implement your IMA3 plugin, the notation for setting options with JavaScript is slightly different.
Here you see options in JSON format you could use in Studio:
{
"requestMode": "onload",
"serverUrl": "//solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap.xml?cust_params={mediainfo.ad_keys}",
"timeout": 5000
}
If you are using JavaScript to configure your options, the JSON format would work, but you could also use the JavaScript syntax as shown here:
{
requestMode: 'onload',
serverUrl: '//solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap',
timeout: 5000
}
Options
The video.js IMA3 plugin is built on top of the video.js ads framework and accepts any options that the ad framework provides. Take a look at the ad framework README for details on the current set of overrideable settings. Both the IMA plugin specific options and ad framework options are documented here.
The options are:
- clickTrackingElement
- debug
- debugContribAds
- disableCustomPlaybackForIOS10Plus
- hardTimeouts
- ima3SdkSettings
- requestMode
- serverUrl
- showVpaidControls
- timeout
- useMediaCuePoints
- usePlayerAutoplayHandling
- vpaidMode
clickTrackingElement
Type: HTMLElement
Default Value: undefined
If the HTML ad tech is being used in custom ad playback mode, this specifies an alternative HTML element to be used to track advertisement taps on devices that don't support input events over the video element. More details are available in the parameter documentation for the IMA AdDisplayContainer. If you provide a click tracking element, it is your responsibility to show and hide it at the appropriate times on the appropriate platforms. In most cases, it's best to leave this setting undefined and allow the plugin and IMA to manage ad interaction.
debug
Type: boolean
Default Value: false
If debug is set to true, the ads framework will output additional information about its current state during playback. This can be handy for diagnosing issues or unexpected behavior in an ad integration.
This option is part of the ad framework and is configured as follows:
player.ima3({
debug: true
});
The following screenshot shows the extensive information displayed by turning debug on.
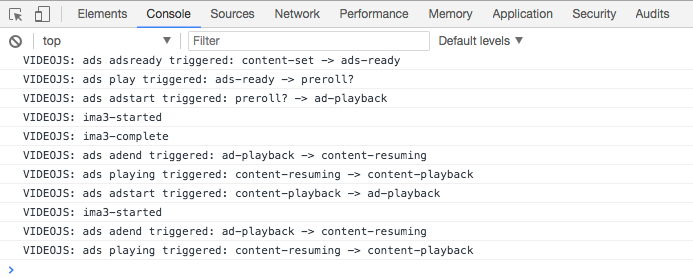
debugContribAds
Type: boolean
Default Value: false
Used to enable debug logging for videojs-contrib-ads. For ID3 oncue
requests to work, the ID3 TXXX frame received must contain JSON data with the following fields:
- Ad cue tag format:
- name: Required - must be the string
adCue
- id: Required - a unique value to identify the ad given that times are relative in live streams
- serverUrl: Optional - Override the
serverUrl
in the IMA3 config for this ad only - duration: Optional - appended as a
breaklength
parameter to the server url
{"name": "adCue", "id": 123}
- name: Required - must be the string
- Ad cancellation tag format:
- name: Required - must be the string value
adCancel
- id: Required - a unique value to identify the ad cancellation given that times are relative in live streams
{"name": "adCancel", "id": 234}
- name: Required - must be the string value
disableCustomPlaybackForIOS10Plus
Type: boolean
Default Value: false
This property is part of the ima3SdkSettings
object. It acts as a getter and setter to control whether to disable custom
playback on iOS 10+ browsers. Behavior is as follows:
- When true, ads will play inline if the content video is inline, which enables skippable ads. However, the ad will stay inline and not support iOS's native fullscreen.
- When false, ads will play in the same player as your content.
See the iOS skippable ads section of this document for an example of usage of this setting.
hardTimeouts
Type: boolean
Default Value: true
Abandon ads that finish loading after they have timed out. This will prevent slow preroll ads from interrupting after the
timeout
has elapsed and content video has begun playing.
This option is part of the ad framework and is configured as follows:
player.ima3({
hardTimeouts: true
});
Setting this option to false
will result in content flash before ads.
ima3SdkSettings
Type: object
Default Value: undefined
If provided, the properties of this object are used to set the page-level Ima3SdkSettings when the IMA SDK has finished loading. The properties of this object are expected to be the camel-cased equivalent of setter methods on the SDK settings object, minus the 'set' prefix. For example, if you provided this object when initializing the plugin:
player.ima3({
ima3SdkSettings: {
'numRedirects': 3,
'ppid': 'publisher-provided-id'
}
}
Then the video.js IMA plugin would run code that looks something like this when IMA had loaded:
window.google.ima.ImaSdkSettings.setNumRedirects(3);
window.google.ima.ImaSdkSettings.setPpid('publisher-provided-id');
requestMode
Type: string
Default Value: onload
This option is part of the ad framework is configured as follows:
player.ima3({
requestMode: 'onplay'
});
There are four possible values for this option:
-
onload
: Ads are requested immediately when the player loads. This is normally the best experience for GAM / VPAID. -
onplay
: The first ad request is delayed until playback is initiated. This is important for ad networks which consider ad requests to be plays, so you would not want an ad request before a play request. This will cause ads trafficked to eventually drop, or the customer will receive less for the ads shown. -
ondemand
: Ads will only play when initiated using theplayer.ima3.adrequest()
method manually. This mode does not support preroll or postroll ads. -
oncue
:This option behaves differently depending upon if it is use with theuseMediaCuePoints
option.Using cue points with Video Cloud
You can create ad cue points for a video in Studio, then have an advertisement played when the cue point is triggered. For full details see the Display Ads Using Ad Cue Points document.
Using cue points in a live stream
Ads are requested based on ID3 cue points in a live stream. For this type of request to function correctly, the ID3 TXXX frame received must contain JSON data with the following fields:
name
: Required; must be the string valueadCue
id
: Required; A unique value to identify the ad given that times are relative in live streamsserverUrl
: Optional; Appended as a break length parameter to the server urlduration
: Optional; ad duration
Example:
{"name": "adCue", "id": 123}
You can also cancel ID3 cue points in a live stream created by an
adCue
by usingadCancel
. The following format must be used for the object sent:{"name": "adCancel", "id": 123}
Both the
name
andid
are required.- Live ID3 ad cues are not supported on Android.
- Live ID3 ad cancellation cues are not supported on iOS.
serverUrl
Type: string
or function
Default Value (a generic Google ad):
//pubads.g.doubleclick.net/gampad/ads?sz=400x300&iu=%2F6062%2Fiab_vast_samples&ciu_szs=300x250%2C728x90&gdfp_req=1&env=vp&output=xml_vast2&unviewed_position_start=1&url=[referrer_url]&correlator=[timestamp]&cust_params=iab_vast_samples%3Dlinear
Here, you will provide the URL to your ad server. This option can be configured in Brightcove Studio as shown above. You can also set the value in code. Two examples are shown next. (Remember, you should test the ad tag on your server before trying to use it in the plugin.)
If the value is a string, it represents the ad server URL from where ads are requested and can be set in code as follows:
player.ima3({
serverUrl: 'your ad server'
});
If the value is a function, the function parameter is a callback
function that should be called with your server URL as
the argument. This provides support for asynchronous processes such as header bidding. In the following example you see information from the
mediainfo
object used to determine which ads URL should be used based on an account ID:
// Initialize IMA plugin
myPlayer.ima3({
serverUrl: function(callback) {
if (myPlayer.mediainfo.id === '4034552795001') {
callback('https://pubads.g.doubleclick.net/.../url1');
} else {
callback('https://pubads.g.doubleclick.net/.../url2');
}
},
requestMode: 'onload',
debug: true,
debugContribAds: true
});
showVpaidControls
Type: boolean
Default Value: false
Set to true
to show custom Brightcove controls over VPAID ads. They may or may not work depending on the VPAID
implementation, so Brightcove suggests testing this feature with your ads before enabling it in production.
timeout
Type: number
Default Value: 4000
The maximum amount of time, in milliseconds, to wait for ads to play before an ad break is skipped.
This option is part of the ad framework is configured as follows:
player.ima3({
timeout: 5000
});
In internal Brightcove testing it was found that four seconds seemed to be long enough to accommodate slow initialization in most cases, but still short enough that failures to initialize didn't look like failures of the player or content video.
useMediaCuePoints
Type: boolean
Default Value: false
Enables the use of ad cue points (as defined in Studio) to trigger an ad to play.
For Video Cloud ad cuepoints to be used to trigger ads, the following is required in the plugin configuration:
useMediaCuePoints
: truerequestMode
: The stringoncue
serverUrl
: Must point to a valid VAST ad
If you are using Studio to setup advertising, when you select On cue point the useMediaCuePoints
and
requestMode
options will be set correctly for you.
usePlayerAutoplayHandling
Type: boolean
Default Value: false
Set to true
to use the Brightcove Player's core autoplay handling. Otherwise, uses the IMA3 Plugin's default autoplay handling.
vpaidMode
Type: string
Default Value: ENABLED
Specify VPAID 2 mode in the IMA HTML5 SDK.
There are three possible values for this option:
ENABLED
: Play VPAID ads in an iframe with a different domain.INSECURE
: Play VPAID ads in an iframe with the same domain.DISABLED
: VPAID ads throw an error.
This option is configured as follows:
player.ima3({
vpaidMode: 'DISABLED'
});
Properties
Only one property exists for the plugin:
- html5: This is the only ad tech that can be loaded when the plugin initializes.
Ad macros and the serverUrl
There are ad macros available to make your job easier when creating the ad server URL. These macros enable you to use variables in the server URL for which the IMA3 plugin will substitute appropriate values. For instance, the following server URL contains some of the variables:
{"serverUrl": "//myadserver.com/ad?video={mediainfo.id}&duration={player.duration}"}
The IMA3 plugins would substitute in the appropriate values, and the server URL actually used would appear as follows:
{"serverUrl": "//myadserver.com/ad?video=12340001&duration=60"}
The following is the complete list of variables for which substituted values will be used:
Macro | Description |
---|---|
{document.referrer} | Referring page URL. |
{mediainfo.ad_keys} |
Free form text string that can be added and edited in the Media module of Studio; you should use the query parameter in the form
|
{mediainfo.description} | Short description (250 chars max) |
{mediainfo.duration} | Duration of the video as reported by Video Cloud |
{mediainfo.id} | Video ID |
{mediainfo.name} | Video title |
{mediainfo.reference_id} | Reference ID |
{mediainfo.tags} | Tags (metadata) associated with the video |
{player.duration} |
Duration of video as measured by player (possibly slightly different from mediainfo.duration and probably more accurate). Note that this returns 0 if video is not loaded. Be careful timing your ad request with this macro.
|
{player.height} | The current player height |
{player.id} | Player ID |
{player.pageUrl} | The page URL: Returns document referrer if in an iframe, otherwise window location. |
{player.width} | The current player width |
{playlist.id} | Pulled from playlistinfo object |
{playlist.name} | Pulled from playlistinfo object |
{random} | A random number 0-1 trillion (Used to create a unique impression. This prevents the ad from being cached in the browser and prevents impression discrepancies.) |
{timestamp} | Current local time in milliseconds since 1/1/70 |
{window.location.href} | Current page URL |
Default values and ad macros
You can give ad macros default values. A default value can be provided within a macro, in which case this value will be used where a variable is undefined. The syntax is:
{macro=default}
For instance,
http://example.com/ad/{pageVariable.adConf=1234}
would resolve to the following if window.adConf
is undefined:
http://example.com/ad/1234
Dynamic macros
Dynamic macros provide access to values in the following:
- The video's
customFields
object through themediainfo.customFields
variable. - The DOM's
window
object through thepageVariable
variable.
For example, you could use the following in your ad request:
//myadserver.com/ad?l={pageVariable.navigator.language}&category={mediainfo.customFields.type}
For the pageVariable
values, only certain value types are allowed to be used, as shown in this table:
Type | What Happens |
---|---|
String | Use without changes |
Number | Converted to string automatically |
Boolean | Converted to string automatically |
Null | Returns the string null |
Undefined | Logs warning and returns empty string |
Other | Logs warning and returns empty string |
TCF macros
If a consent management platform, or CMP (essentially a range of advertising technologies all rolled into one easy-to-use platform), supporting the GDPR Transparency and Consent Framework (TCF) is in use, additional macros are made available. The syntax is {tcf.*}
, with *
being a property in the tcData object.
The most commonly used macros are:
Name | Value |
---|---|
{tcf.gdprApplies} | Whether GDPR applies to the current session |
{tcf.tcString} | The consent string |
Since gdprApplies
is a boolean and many ad servers expect the value as an integer, an additional {tcf.gdprAppliesInt}
is provided which will return 1 or 0.
If the player is in an iframe, a proxy will be added if the TCF API is detected in any parent frame to gain consent with the postmessage
API. The CMP must be loaded before the player.
Default values in macros
A default value can be provided within a macro, in which case this value will be used where not resolvable e.g.
http://example.com/ad/{pageVariable.adConf=1234}
becomes
http://example.com/ad/1234
if window.adConf
is undefined.
Custom ad macros
Although the Dynamic macros technique described just above is the much preferred method to access specific
information via macros, you may have defined custom values you use when requesting ads from your ad server that you cannot reach via dynamic macros. In
this case, you can utilize your values by overriding the adMacroReplacement()
method. When you override this method it
allows you to pass your specialized values for the ad request.
The following is an example of overriding the adMacroReplacement()
method. In this example, the custom values are defined
as part of the page DOM, thus enabling ad requests to be customized on a per page basis. In this example,
mySite.category
is the location where the ad request information is stored.
brightcovePlayer.ima3.adMacroReplacement = function (url) {
var parameters = {
'{category}': mySite.category
};
for (var i in parameters) {
url = url.split(i).join(encodeURIComponent(parameters[i]));
}
return url;
}
Using specific values will help clarify exactly what is happening. Assume your request URL to the ad server is the following:
//myadserver.com/myads?adWord={category}
And assume the value dynamically assigned to mySite.category
in the page is the string
fishing-pole. Then after your version of the adMacroReplacement()
method is called
your ad request URL would appear as:
//myadserver.com/myads?adWord=fishing-pole
To summarize, when you override the adMacroReplacement()
method it allows you to use custom values as ad macros, and
dynamically assign values to the URL ad request.
Methods
When you need to interact with the IMA SDK you need to wait for the ima3-ready
event to be dispatched before successful use of the SDK can
take place. This includes the following two methods.
player.ima3.adrequest( )
Calling this method will create an on-demand adrequest immediately upon receiving an ad response. Invoking this method creates a new IMA adManager which means that any previous ad response information (for instance, a postroll ad returned in a previous VAST response) will be lost. Brightcove recommends that you only use this method in cases where up-front knowledge of ad timings isn't known or you will be making all ad calls on-demand. In all other cases, it makes sense to put all ad data in the initial VAST call on plugin initialization.
The following are two important points to consider when using player.ima3.adrequest( )
:
- The method is for use with the ondemand request mode only.
- The method is not recommended for prerolls as content will play before the ad request completes, resulting in a flash of content.
Parameters
-
adRequestUrl
String
Path to a VAST ad tag. You can and should pass relative URLs. This parameter is optional and the configuredserverUrl
is used if no parameter is passed.
Returns:
- Nothing
Example
player.ima3.adrequest('//pubads.g.doubleclick.net/gampad/ads?sz=640x360&iu=/6062/iab_vast_samples/skippable&ciu_szs=300x250,728x90&impl=s&gdfp_req=1&env=vp&output=xml_vast2&unviewed_position_start=1&url=[referrer_url]&correlator=[timestamp]');
player.ima3.setAdsRenderingSettings()
This method lets you set ads rendering settings for the IMA SDK for HTML5.
If there is no Ads Manager yet, this method saves your settings and when an Ads Manager is created it uses the settings you provided. If an Ads Manager
already exists, it is updated to use your settings. In either case, any new Ads Managers created in the future will also use the most recent settings you
provided. You can create an AdsRenderingSettings
object by accessing the IMA SDK directly.
A variety of settings are available.
Parameters
- google.ima.AdsRenderingSettings object
Returns:
- Nothing
Example:
var adsRenderingSettings = new google.ima.AdsRenderingSettings();
adsRenderingSettings.bitrate = 2500;
adsRenderingSettings.enablePreloading = true;
player.ima3.setAdsRenderingSettings(adsRenderingSettings);
Google's AdsManager
There are methods and properties available from Google's
google.ima.AdsManager Interface. You can
use the interface's properties/methods that retrieve information. It is not advised to use the methods that perform actions, like
destroy
, setAutoPlayAdBreaks
and stop
. For example, one
method you can use is shown here:
AdsManager.getRemainingTime
Type: google.ima.AdsManager.getRemainingTime
Usage: myPlayer.ima3.adsManager.getRemainingTime()
Calling this method returns the amount of time remaining for the current ad. If an ad isn't available or has finished playing, it returns -1. For more information see Google's documentation on the method.
Accessing the IMA SDK directly
A number of IMA settings are available on the plugin object at runtime. For instance, to determine the ad ID you would use:
var adId = player.ima3.currentAd.getAdId();
Be careful interacting directly with these properties. Calling the wrong method can lead to unexpected results and failure of advertisements to play properly.
adsLoader
Type: google.ima.AdsLoader
The object used to create ads requests. See
ima.AdsLoader. The Ads Loader may not be
available until adsready
has been fired by the plugin.
adsManager
Type: google.ima.AdsManager
The object responsible for playing ads. See
ima.AdsManager. The Ads Manager is not
available until adsready
has been fired by the plugin.
adDisplayContainer
Type: google.ima.AdDisplayContainer
The object responsible for managing the display elements for ads. See
ima.AdDisplayContainer. The Ads
Display container may not be available until adsready
has been fired by the plugin.
currentAd
Type: google.ima.Ad
When an ad is playing, an object that encapsulates information about the current ad. See ima.Ad.
Events
The plugin emits some custom event types during loading, initialization, and playback. You can listen for IMA3 and ad framework events just like you would any other event:
player.on('ima3-ready', function(event) {
console.log('event', event);
});
Event | Dispatched when: |
---|---|
ima3-ready | The ima3 plugin code has loaded and is ready load the IMA SDK. |
adserror | Indicates the SDK failed to load, but will also be triggered for other SDK-related errors. For example, if the AdsManager fails to initialize or start correctly. |
ima3-ad-error | An error has occurred in the IMA3 SDK. You should verify your ad configuration and settings to be sure your DoubleClick account is correctly configured. You can find common troubleshooting tasks at the DoubleClick support site or talk to your DoubleClick account representative. |
ads-request | Upon request ad data. |
ads-load | When ad data is available following an ad request. |
ads-ad-started | An ad has started playing. |
ads-ad-ended | An ad has finished playing. |
ads-pause | An ad is paused. |
ads-play | An ad is resumed from a pause. |
ads-ad-skipped | An ad is skipped. |
ads-first-quartile | The ad has played 25% of its total duration. |
ads-midpoint | The ad has played 50% of its total duration. |
ads-third-quartile | The ad has played 75% of its total duration. |
ads-click | A viewer clicked on the playing ad. |
ads-volumechange | The volume of the playing ad has been changed. |
ads-pod-started | The first ad in a linear ad pod (a sequenced group of ads) has started. |
ads-pod-ended | The last ad in a linear ad pod (a sequenced group of ads) has finished. |
ads-allpods-completed | All linear ads have finished playing. |
Re-dispatched ima3-
prefixed events
When the IMA3 plugin is used in HTML mode, all AdErrorEvents, AdEvents and
AdsManagerLoadedEvents are re-dispatched onto the player. When the events are re-dispatched, they are prefixed with
ima3-
. The following table shows the re-dispatched events:
Re-Dispatched Event |
---|
ima3-ad-error |
ima3-ads-manager-loaded |
ima3-all-ads-completed |
ima3-click |
ima3-complete |
ima3-content-pause-requested |
ima3-first-quartile |
ima3-hardtimeout |
ima3-loaded |
ima3-midpoint |
ima3-paused |
ima3-ready |
ima3-resumed |
ima3-started |
ima3-third-quartile |
ima3-volume-changed |
Live video and IMA3
If you are using version 3.1.0+ of the IMA3 plugin, you can use pre-rolls with a live event. The pre-roll will play when each viewer begins to watch the live event, not the one time the live event begins. When configuring your event in the Live module, as shown in the Creating and Managing Live Events using the Live Module document, you are prompted to select a player. You will want to configure advertising for the player you select, as shown in the Step-by-Step: Implementing Advertising document.
Note the following details of the implementation:
- Only pre-rolls will play. Mid-rolls and post-rolls are not supported.
- The Request Ads type must be either On load or On play.
- You must be using version 3.1.0 or later of the IMA3 plugin, as mentioned earlier.
Player Ad Libraries
The videojs/videojs-contrib-ads GitHub repository contains a plugin which provides common functionality needed by video advertisement libraries working with Brightcove Player. The plugin provides common functionality needed by video ad integrations and takes care of a number of concerns for ad integrators, reducing the code you have to write for your ad integration. The plugin is fully documented, with an index page as the best starting point.
There are methods, events and properties available for use from the plugin. Full details are provided in the videojs-contrib-ads API reference. A sampling of the tools available are provided here, starting with methods:
Method | Description |
---|---|
isInAdMode() | Returns true if the player is in ad mode. |
isWaitingForAdBreak() | This method returns true during ad mode if an ad break hasn’t started yet. |
isContentResuming() | This method returns true during ad mode after an ad break has ended but before content has resumed playing. |
The plugin also dispatches numerous events, a few are listed here:
Event | Description |
---|---|
adstart | Fired directly as a consequence of calling startLinearAdMode() . |
adend | fired directly as a consequence of calling endLinearAdMode() . |
readyforpreroll | Indicates that your ad plugin may start a preroll ad break by calling startLinearAdMode() . |
The plugin also provides numerous properties, a few are listed here:
Name | Data Type | Description |
---|---|---|
ads.ad.id | String | Unique identifier for an ad that plays |
ads.ad.index | Number | The index of the ad that plays at a specified time; the index would identify the ordinal value of an ad in an ad pod |
ads.ad.duration | Number | The duration of the ad in seconds |
ads.ad.type | String | Either PREROLL, MIDROLL or POSTROLL |
The following code demonstrates the use of the properties:
var myPlayer,
player = bc('myPlayerID');
player.ima3({
serverUrl: '//solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap.xml'
});
player.ready(function () {
myPlayer = this;
myPlayer.on('ads-ad-started', function (evt) {
console.log('ads-ad-started event passed to event handler', evt);
console.log('myPlayer.ads.ad.id', myPlayer.ads.ad.id);
console.log('myPlayer.ads.ad.index', myPlayer.ads.ad.index);
console.log('myPlayer.ads.ad.duration', myPlayer.ads.ad.duration);
console.log('myPlayer.ads.ad.type', myPlayer.ads.ad.type);
});
});
The output in the console from the above code is shown here:
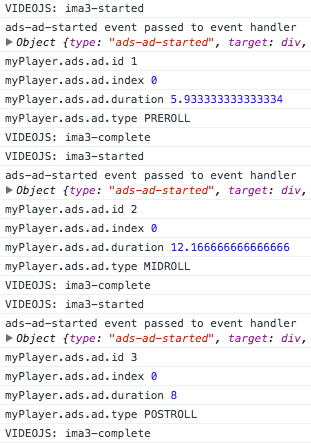
Implement using code
To implement the IMA3 Plugin the player needs to know the location of the plugin code, a stylesheet, the plugin name and plugin configuration options. The location of the plugin code and stylesheet are as follows:
https://players.brightcove.net/videojs-ima3/4/videojs-ima3.min.js
https://players.brightcove.net/videojs-ima3/4/videojs-ima3.css
The name of the plugin is ima3
, and an example set of options is:
{
"serverUrl": "//pubads.g.doubleclick.net/gampad/ads?sz=400x300&iu=%2F6062...",
"timeout": 5000
}
The following example uses the In-Page Embed implementation of the player to associate the IMA Plugin with a single instance of a player.
-
Line 12: Uses a
link
tag to include the plugin's CSS in thehead
of the HTML page. -
Line 15: Gives the
video
tag anid
attribute, with some value, in this case myPlayerID. -
Line 23: Uses a
script
tag to include the plugin's JavaScript in thebody
of the HTML page. -
Lines 26-29: Initializes the player using the
bc()
method, and then calls theima3()
method. -
Lines 30-33: On player
ready
, a reference to the player is created. The comment is shown to indicate where you can place code to add other player behaviors beyond IMA3 plugin setup and configuration.
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>IMA3 Plugin Code Example</title>
<style>
.video-js {
height: 344px;
width: 610px;
}
</style>
<link href="https://players.brightcove.net/videojs-ima3/4/videojs-ima3.css" rel="stylesheet">
</head>
<body>
<video-js id="myPlayerID"
data-video-id="3851380732001"
data-account="1752604059001"
data-player="Hy3gDJHu"
data-embed="default"
controls=""></video-js>
<script src="https://players.brightcove.net/1752604059001/Hy3gDJHu_default/index.min.js"></script>
<script src="https://players.brightcove.net/videojs-ima3/4/videojs-ima3.min.js"></script>
<script>
var myPlayer;
var player = bc('myPlayerID');
player.ima3({
serverUrl: '//solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap.xml'
});
player.ready(function() {
myPlayer = this;
//Do something
});
</script>
</body>
</html>
Hybrid implementation
So far in this document you have seen the IMA plugin implemented in Studio, then in code. Some customers like to perform a hybrid implementation where the basic plugin is added in Studio, but then configuration done in the page's JavaScript. This hybrid implementation is discussed in this section.
As you have just seen in the previous section, when implementing the IMA3 plugin purely in code you manipulate the plugin in function format. When you install the plugin in Studio, then configure in the page, you must treat the plugin as an object. For instance, assume you have installed the IMA3 plugin using Studio as shown here, noting that no Ad Tag is supplied:
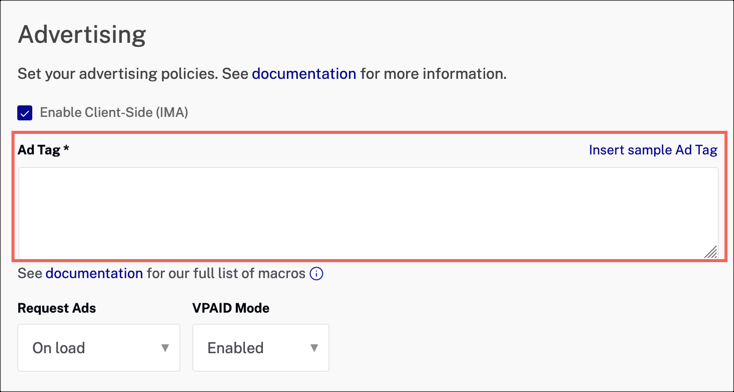
Now, in the JavaScript in the page you can assign property values (remember, treat the plugin as an object now) as shown here:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
myPlayer.ima3.settings.serverUrl = 'http://solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap.xml';
});
Of course, other properties can be assigned values in this manner.
Debugging assistance
There are two options for assistance when debugging ad playback issues. If you do nothing, in the console you will see only information on when ads started and finished:
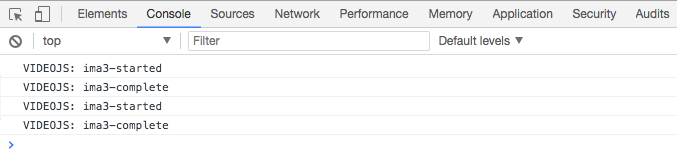
The first option is mentioned earlier in this document in the Options section. This turns on debugging at the plugin level. You will see additional debugging information:
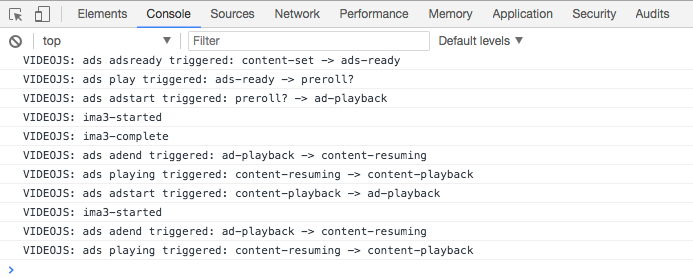
The second option utilizes a tool supplied by Google. You specify a sdkurl option with a value pointing to a JavaScript file supplied by Google. An example configuration is shown here:
var myPlayer = bc('myPlayerID');
myPlayer.ima3({
"serverUrl": "//solutions.brightcove.com/bcls/brightcove-player/vmap/simple-vmap.xml",
"debug": true,
"sdkurl": "//imasdk.googleapis.com/js/sdkloader/ima3_debug.js"
});
myPlayer.ready(function() {
myPlayer = this;
});
The expanded debugging information would appear similar to the following. (The yellow highlighted information is from the plugin's debugging and the blue highlighted information is generated by the Google tool.)
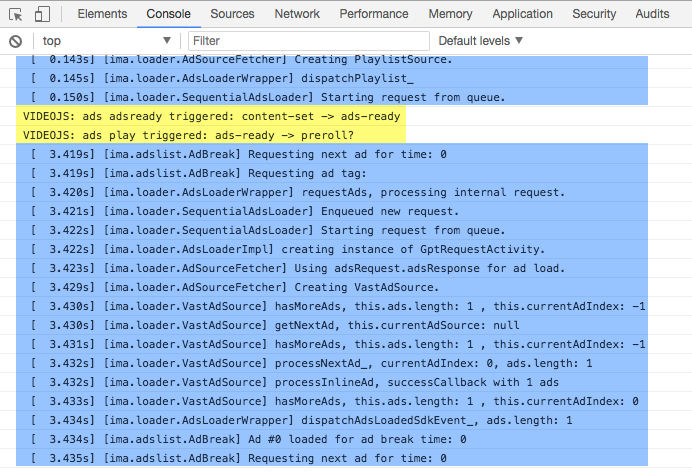
For more details on Google's IMA errors, see the Class google.ima.AdError section of Google's Google IMA HTML5 SDK APIs document.
Known issues
Picture-in-Picture disabled
The picture-in-picture control disabled when the IMA3 plugin is implemented. This is an intentional design decision.
Setting autoplay in Studio
Do NOT set autoplay in Studio if you are using the IMA3 plugin to display advertisements. Setting autoplay in Studio could cause ad playback failure. See the Autoplay Considerations document for further information.
Learn More and Ad Countdown Timer no longer displayed by default
A work around exists using the useStyledLinearAds
property from Google's IMA SDK. Set this value to true, as shown here:
adsRenderingSettings.useStyledLinearAds=true;
For more details, see Google's google.ima.AdsRenderingSettings documentation.
Chrome on Android: Unmute pre-roll, video will not autoplay
When using Chrome (latest version) on Android and unmute the player while pre-roll is playing, player does not start playing a video automatically after pre-roll ends. If you unmute the ad, the volume persistence feature of Brightcove Player will also unmute the content since the intention of the viewer is to hear the audio. However, on newer versions of Chrome Android, unmuted content cannot be auto-played and the user-gesture requirement to begin playback has been added. This is an OS/Device-level setting and not something Brightcove can override.
Preroll's Current Time display may not be accurate
This problem has to do with a limitation in the way the IMA SDK reports the current time. There is no work around documented at this time.
Unmuted midrolls may not play on Safari 11 Desktop
Unmuted midrolls may not play on Safari 11 Desktop due to a change in Google's IMA SDK. A new behavior was introduced to trigger an error and cancel an ad when autoplay is rejected by Safari 11 (Desktop and possibly iOS). Midrolls impacted in this way will trigger an ad error 400, with error code 1205 indicating autoplay was prevented.
Supported environments
To check for supported combinations of platforms, advertising standards and video media, see Google's Video Feature and SDK compatibility document. That document and the Video Suite Inspector will help you diagnose attempted IMA3 advertisement configurations that simply will not function.
Overlays and Fullscreen Transitions
video.js uses the fullscreen API where available. Different browsers implement the transition to fullscreen differently and that can produce discrepancies in appearance when transitioning into and out of fullscreen mode. In most implementations, the element that is being taken into fullscreen is geometrically scaled (i.e. zoomed) from its original to the target size. Most overlay advertisements are designed to be displayed at a fixed size, however, and so they may appear distorted until the animation completes.
Skippable ads on iOS devices
With the IMA3 plugin, skippable ads can be played on iPhones when the following conditions are met:
- The
playsinline
attribute is present on thevideo
element -
The
disableCustomPlaybackForIOS10Plus
setting is passed to the plugin, and set totrue
For the playsinline
attribute, simply include it as an attribute in the video
tag:
<video-js id="player"
width="640"
height="360"
data-video-id="4524585416001"
data-account="4360108595001"
data-player="r12ukws9l"
data-embed="default"
playsinline
controls=""></video-js>
For the disableCustomPlaybackForIOS10Plus
setting, assign it as a property of
ima3SdkSettings
:
player.ima3({
ima3SdkSettings: {
"disableCustomPlaybackForIOS10Plus": true
}
})
If you are using Studio and wish to use the setting there, add this to your IMA3 plugin configuration:
{
"ima3SdkSettings": {
"disableCustomPlaybackForIOS10Plus": true
}
}
For full details of this setting, see the disableCustomPlaybackForIOS10Plus entry in the
ima3SdkSettings
section of this document.
Skippable ad limitations:
- The Skip Ad button may be partially covered by the ad control bar on some mobile devices. This makes it difficult for end users to actually skip the ad. The user can pinch to zoom to make the Skip Ad button bigger on mobile devices to be able to click it.
-
iPhone without
playsinline
anddisableCustomPlaybackForIOS10Plus
- Skippable ads are not played. -
iPhone with
playsinline
anddisableCustomPlaybackForIOS10Plus
- Ads are played inline, but if using fullscreen, ads do not show, but audio for the ad will play. In other words, skippable ads in fullscreen do not function correctly. - iPad - Ads are played inline but skip is not available in fullscreen mode
iOS 10 iPad and iPhone: Preroll ads not working when using playsinline
and going fullscreen
If using playsinline
on iOS 10 iPad and iPhone to allow viewing a video not fullscreen, then a preroll ad starts, and the fullscreen button
is clicked, the ad will NOT continue to play. This is a limitation of the Google's IMA implementation, and Google does not have plans to correct the
issue.
Conflict with gpt_proxy.js
If using the GPT proxy script with IMA3, and the adTechOrder is HTML5 first, playback issues can occur. The IMA3 plugin will be affected when using any script that uses window.google or window.google.ima. It is suggested that you check if you're using Brightcove Player, and if so, don't load the proxy on those occasions.
Resize Skip Ad button
It is not possible to resize the Skip Ad button. The Brightcove Player API does not have methods or properties to specify the size of the Skip Ad button. At the developer level, if a publisher is using VPAID ads, the ads can implement their own Skip Ad button and logic to fit within the UI and element distribution of Brightcove Player.
Ads will not autoplay on iOS
Although not specific to the IMA3 plugin, know that autoplay
is restricted iOS, so ads will not start until a user
gesture is made.
Ad cue point issues
For issues specific to using ad cue points, see the Known issues section of the Displaying Ads Using Ad Cue Points document.
IMA3 SDK's url
parameter
Google Ad Manager's url
parameter is the full URL from which the ad request is sent. Note that this value is automatically set by the IMA SDK, and will in fact overwrite any values you may provide.
Changelog
See the IMA3 Plugin Release Notes.
For historical release notes, see the changelog here.