Player example
In this sample you click the Add Player button to dynamically build and load the player, and then play the video.
See the Pen 18169-loading-player-dynamically by Brightcove Learning Services (@rcrooks1969) on CodePen.
Source code
View the complete solution on GitHub.
Using the CodePen
Here are some tips to effectively use the above CodePen:
- Toggle the actual display of the player by clicking the Result button.
- Click the HTML/CSS/JS buttons to display ONE of the code types.
- Later in this document the logic, flow and styling used in the application will be discussed in the Player/HTML configuration, Application flow and Application styling sections. The best way to follow along with the information in those sections is to:
- Click the EDIT ON CODEPEN button in the CodePen and have the code available in one browser/browser tab.
- In CodePen, adjust what code you want displayed. You can change the width of different code sections within CodePen.
- View the Player/HTML configuration, Application flow and/or Application styling sections in another browser/browser tab. You will now be able to follow the code explanations and at the same time view the code.
Development sequence
Here is the recommended development sequence:
- Use the In-Page embed player implementation to test the functionality of your player, plugin and CSS (if CSS is needed)
- Put the plugin's JavaScript and CSS into separate files for local testing
- Deploy the plugin code and CSS to your server once you have worked out any errors
- Use Studio to add the plugin and CSS to your player
- Replace the In-Page embed player implementation if you determine that the iframe implementation is a better fit (detailed in next section)
For details about these steps, review the Step-by-Step: Plugin Development guide.
iframe or In-Page embed
When developing enhancements for the Brightcove Player you will need to decide if the code is a best fit for the iframe or In-Page embed implementation. The best practice recommendation is to build a plugin for use with an iframe implementation. The advantages of using the iframe player are:
- No collisions with existing JavaScript and/or CSS
- Automatically responsive
- The iframe eases use in social media apps (or whenever the video will need to "travel" into other apps)
Although integrating the In-Page embed player can be more complex, there are times when you will plan your code around that implementation. To generalize, this approach is best when the containing page needs to communicate to the player. Specifically, here are some examples:
- Code in the containing page needs to listen for and act on player events
- The player uses styles from the containing page
- The iframe will cause app logic to fail, like a redirect from the containing page
Even if your final implementation does not use the iframe code, you can still use the In-Page embed code with a plugin for your JavaScript and a separate file for your CSS. This encapsulates your logic so that you can easily use it in multiple players.
API/Plugin resources used
API Methods |
---|
play() |
muted() |
Player/HTML configuration
This section details any special configuration needed during player creation. In addition, other HTML elements that must be added to the page, beyond the in-page embed player implementation code, are described.
Player configuration
No special configuration is required for the Brightcove Player you create for this sample. Of course, for this sample the player's code is NOT initially placed in the HTML page, it is dynamically built and injected.
Other HTML
A <button>
is added to the HTML page that triggers the building and injection of the player. Also, a <div>
element is added as the injection location.
Application flow
The basic logic behind this application is:
- Set variable values with the account ID, player ID and video ID you wish to use.
- On button click, a valid
<video>
tag is built using simple string concatenation and that string injected into the HTML page. - A valid player's
<script>
tag is also built and injected into the HTML page. When this is done, the JavaScript will be executed on the page, dispatching anonload
event. In theonload
event handler, call the function that initializes the player. - In the called function, use the
bc()
method to initialize the generic video tag into a Brightcove Player, then call theplay()
method.
Supply desired account ID, player ID and video ID
Find the code which is labeled:
// ### Set the data for the player ###
Assign variables the desired values for player configuration.
Build the player's in-page embed implementation code, and inject into the page
Find the code which is labeled:
// ### Build the player and place in HTML DOM ###
This is JavaScript work. Use string concatenation to build a valid <video>
tag. You then inject the tag into the HTML page. Next you build a valid Brightcove Player <script>
tag. When you append this to the HTML page, the JavaScript will automatically be executed. This creates an onload
event, for which you can write a handler.
Initialize the player as a Brightcove Player, and play the video
Find the code which is labeled:
// ### Initialize the player and start the video ###
You use the standard bc()
method to initialize the generic player as a Brightcove Player, then play the video using the play()
method.
Application styling
The only CSS controls the size of the player.
iframe implementation
The iframe implementation can be used in a very similar way as shown above. Sample code is available. If you wish to have the video play on load, the best thing to do is in the Player Module in the Video Cloud Studio. Set the player's Auto-Play Video on Player Load property under the Playback setting to anything but “Do Not Auto-Play” option.
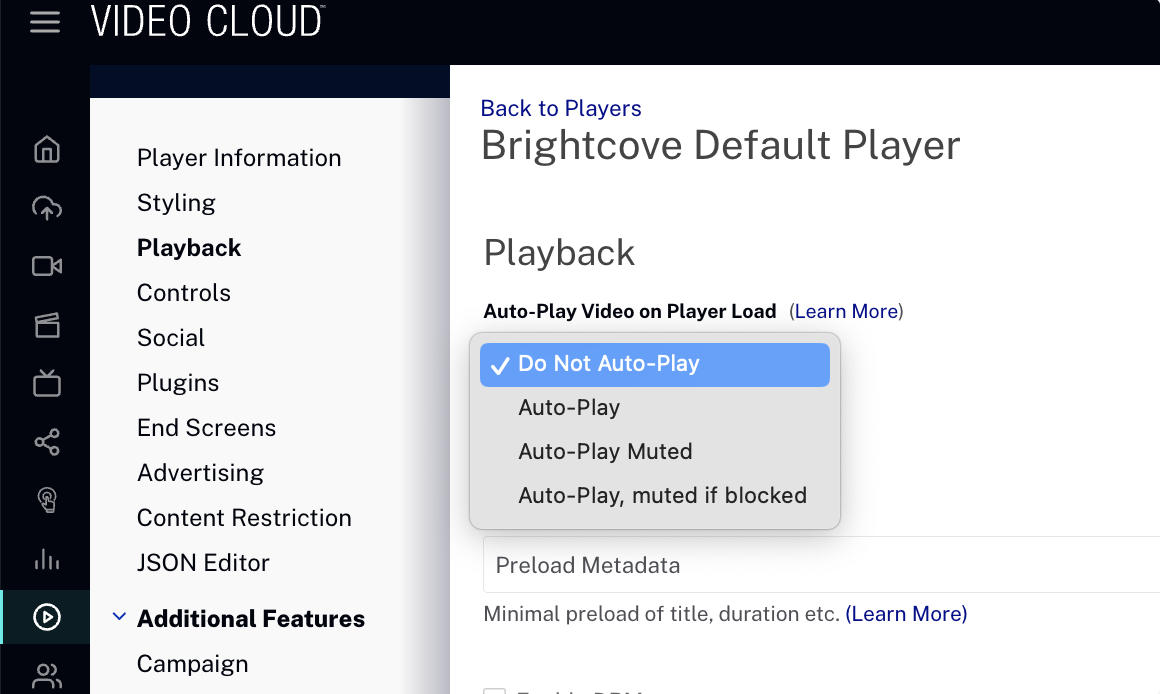
Plugin code
This sample's code will not run as a Brightcove Player plugin as the player must exist before a plugin can be registered with it. Of course, you can save the JavaScript in a separate file and import it if you choose.
Removing a player
You may wish to dynamically remove a player. To do so you should use the myPlayer.dispose()
method. You do NOT want to remove the player from the DOM via JavaScript as this could cause memory leaks. When you use the dispose()
method, the player will remove itself from the DOM.