Brightcove Player Sample: Automatically Set Caption Language
Player example
When you start video playback, you should see the captions appear in the same language as the browser. The video in this example has captions for the following languages:
- English (us)
- German (de)
- Spanish (es)
- French (fr)
If your browser is set for one of these languages, the default caption should be set for that language. You can take this example and add more caption files for different languages.
See the Pen Auto-Caption by Yarianna Tineo (@yariannatineo_) on CodePen.
Source code
View the complete solution on GitHub.
Using the CodePen
Here are some tips to effectively use the above CodePen:
- Toggle the actual display of the player by clicking the Result button.
- Click the HTML/CSS/JS buttons to display ONE of the code types.
- Later in this document the logic, flow and styling used in the application will be discussed in the Player/HTML configuration, Application flow and Application styling sections. The best way to follow along with the information in those sections is to:
- Click the EDIT ON CODEPEN button in the CodePen and have the code available in one browser/browser tab.
- In CodePen, adjust what code you want displayed. You can change the width of different code sections within CodePen.
- View the Player/HTML configuration, Application flow and/or Application styling sections in another browser/browser tab. You will now be able to follow the code explanations and at the same time view the code.
Development sequence
Here is the recommended development sequence:
- Use the In-Page embed player implementation to test the functionality of your player, plugin and CSS (if CSS is needed)
- Put the plugin's JavaScript and CSS into separate files for local testing
- Deploy the plugin code and CSS to your server once you have worked out any errors
- Use Studio to add the plugin and CSS to your player
- Replace the In-Page embed player implementation if you determine that the iframe implementation is a better fit (detailed in next section)
For details about these steps, review the Step-by-Step: Plugin Development guide.
iframe or In-Page embed
When developing enhancements for the Brightcove Player you will need to decide if the code is a best fit for the iframe or In-Page embed implementation. The best practice recommendation is to build a plugin for use with an iframe implementation. The advantages of using the iframe player are:
- No collisions with existing JavaScript and/or CSS
- Automatically responsive
- The iframe eases use in social media apps (or whenever the video will need to "travel" into other apps)
Although integrating the In-Page embed player can be more complex, there are times when you will plan your code around that implementation. To generalize, this approach is best when the containing page needs to communicate to the player. Specifically, here are some examples:
- Code in the containing page needs to listen for and act on player events
- The player uses styles from the containing page
- The iframe will cause app logic to fail, like a redirect from the containing page
Even if your final implementation does not use the iframe code, you can still use the In-Page embed code with a plugin for your JavaScript and a separate file for your CSS. This encapsulates your logic so that you can easily use it in multiple players.
Safari mechanism for user preferred language
Since the Safari browser uses native captions, additional code is necessary to handle Safari's specific mechanism for setting user preferred language.
To see an example of how captions are automatically set by URL parameters, go to Set captions via URL parameter.
Here is a sample code to set captions automatically by <meta>
tags:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="page_language" content="en">
<title>Auto Captions Language</title>
</head>
<body>
<h2>English Page</h2>
<a href="index.html">Return</a>
<div style="width: 960px; height: 540px;">
<video-js id="myPlayerID"
data-account="1752604059001"
data-player="default"
data-embed="default"
controls=""
data-video-id="6008592337001"
data-playlist-id=""
data-application-id=""
width="960" height="540"
class="vjs-fill">
</video-js>
<script src="https://players.brightcove.net/1752604059001/default_default/index.min.js"></script>
</div>
<script>
videojs.registerPlugin('autoCaptions',function(options){
var player = this;
// Use "loadeddata" event. Using "loadedmetadata" event might not work on Safari
player.on("loadeddata", function () {
var browser_language = document.getElementsByName('page_language')[0].getAttribute('content')
var tracks = player.textTracks();
console.log(tracks.length)
// +++ Loop through captions +++
for (var i = 0; i < (tracks.length); i++) {
track_language = tracks[i].language.substr(0, 2);
// +++ When the caption language equals the browser language, then set it as default +++
if (track_language) {
if (track_language === browser_language) {
tracks[i].mode = "showing";
} else {
tracks[i].mode = "disabled";
}
}
}
});
});
videojs("myPlayerID").ready(function() {
var myPlayer = this;
var tracks = null;
var lang = document.getElementsByName('page_language')[0].getAttribute('content');
myPlayer.autoCaptions({"lang":lang});
});
</script>
</body>
</html>
API/Plugin resources used
API Methods | API Events |
---|---|
on() | loadedmetadata |
myPlayer.textTracks() |
Player/HTML configuration
This section details any special configuration needed during player creation. In addition, other HTML elements that must be added to the page, beyond the in-page embed player implementation code, are described.
Player configuration
No special configuration is required for the Brightcove Player you create for this sample.
Other HTML
No other HTML elements are added to the page.
Application flow
The basic logic behind this application is:
- Get the browser language
- Retrieve the caption tracks
- Loop over the captions
- Set the default caption language
Get the browser language
Find the code which is labeled:
// +++ Get the browser language +++
Use navigator.language
to determine the language being used in the browser. Check for navigator.userLanguage
with IE browsers earlier than 10.
Retrieve the caption tracks
Find the code which is labeled:
// +++ Get the captions +++
Use the myPlayer.textTracks()
method to retrieve all the tracks that are displayed in the captions menu. An example of one of the captions is shown here:
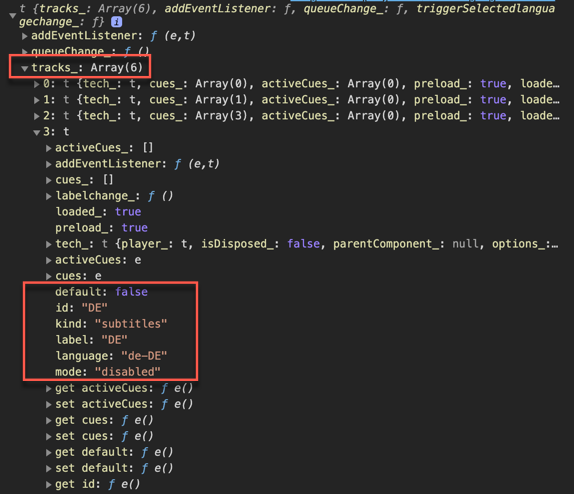
Notice that the object contains both the country label and country code (language). In the JavaScript code, we are searching for the item.track.language
since it is possible that the item.track.label
could be translated by the browser.
Loop over the captions
Find the code which is labeled:
// +++ Loop over the captions +++
Loop over the available captions tracks.
The first two items returned are created automatically and will not have a language defined. These are for the captions settings
and captions off
items that appear when you select the cc button.
Set the default caption language
Find the code which is labeled:
// +++ Set the default caption language +++
When the caption language is equal to the browser language, then set it as the default, by setting the item.track.mode
to a value of "showing"
.
Turn the other caption tracks off by setting the item.track.mode
to a value of "disabled"
.
Application styling
No CSS is needed for this sample.
Plugin code
Normally when converting the JavaScript into a Brightcove Player plugin nominal changes are needed. One required change is to replace the standard use of the ready()
method with the code that defines a plugin.
Here is the very commonly used start to JavaScript code that will work with the player:
videojs.getPlayer('myPlayerID').ready(function() {
var myPlayer = this;
});
You will change the first line to use the standard syntax to start a Brightcove Player plugin:
videojs.registerPlugin('pluginName', function(options) {
var myPlayer = this;
...
});
As mentioned earlier, you can see the plugin's JavaScript code in this document's corresponding GitHub repo: auto-language.js.
Using the plugin with a player
Once you have the plugin's CSS and JavaScript files stored in an Internet accessible location, you can use the plugin with a player. In Studio's PLAYERS module you can choose a player, then in the PLUGINS section add the URLs to the CSS and JavaScript files, and also add the Name and Options, if options are needed.